Affiliate links on Android Authority may earn us a commission. Learn more.
How to open CSV files in Python - store and retrieve large data sets
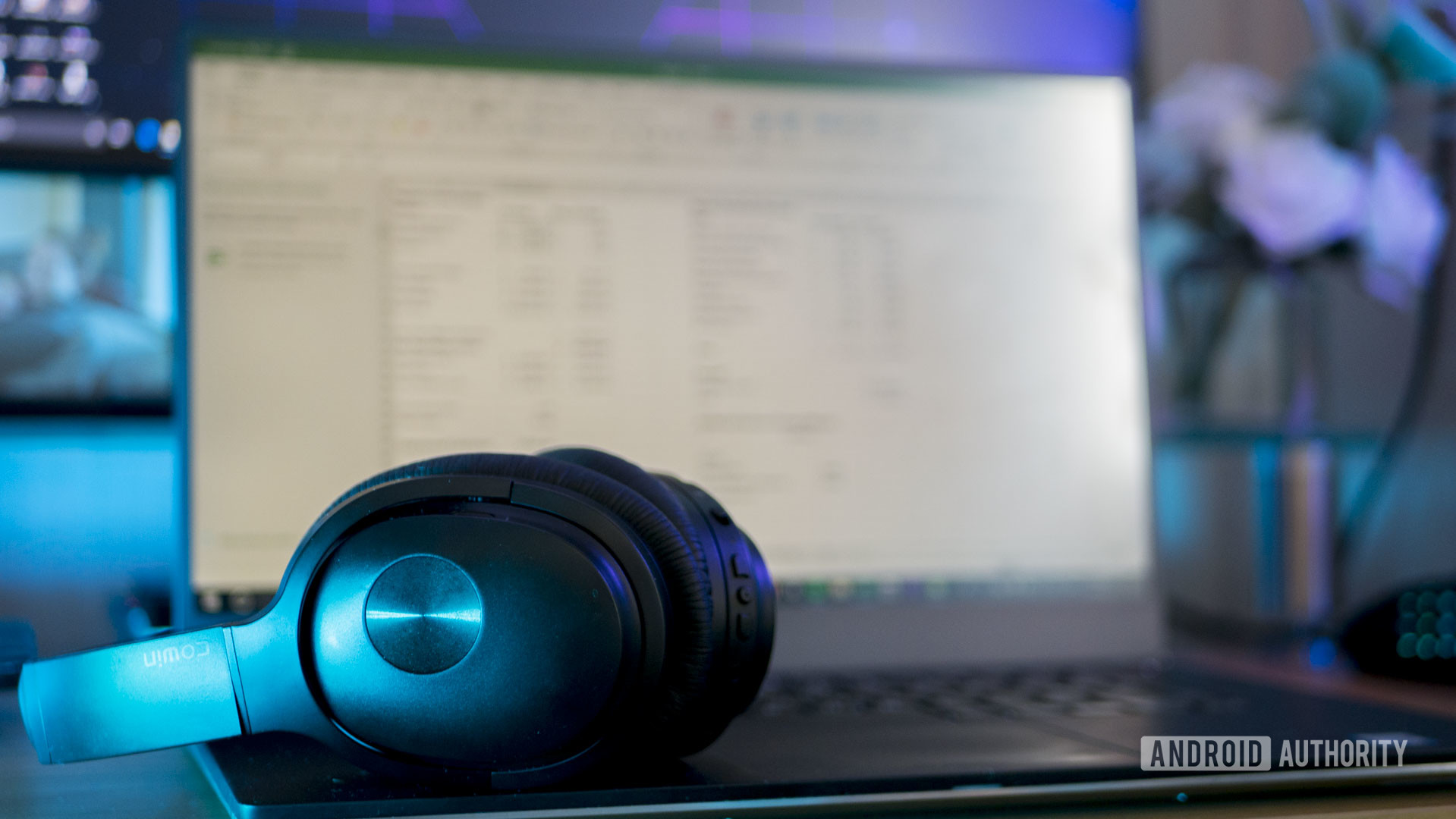
A CSV file is a “comma-separated values” file. In plain English, this is a text file that contains an unusually large amount of data. More often than not, this is used in order to create databases of information, where each unit of data is separated by a comma. Hence the name!
Being able to manipulate, load, and store large amounts of data is a hugely beneficial skill when programming. This is particularly true in Python, seeing as Python is such a popular option for machine learning and data science.
Read on then, and we’ll explore how to read CSV files in Python!
How to read CSV files in Python by importing modules
To get started, we’re first going to create our CSV file.
You can do this in Excel by creating a simple spreadsheet and then choosing to save it as a CSV file. I made a little list of exercises, which looks like so:
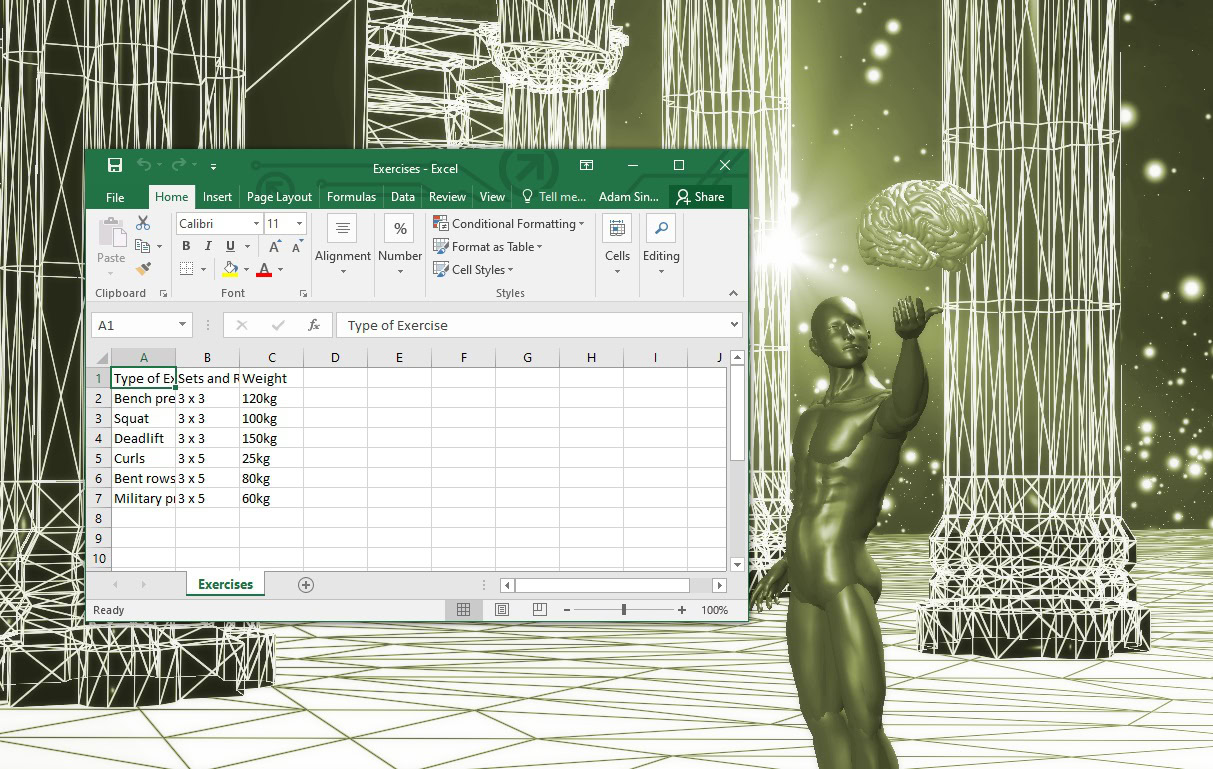
If we open this up as a text file, we see it is stored like this:
Type of Exercise,Sets and Reps,Weight
Bench press,3 x 3,120kg
Squat,3 x 3,100kg
Deadlift,3 x 3,150kg
Curls,3 x 5,25kg
Bent rows,3 x 5,80kg
Military press ,3 x 5,60kg
The top line defines the values, and each subsequent line includes three entries!
So, how do we open this in Python? Fortunately, there is no need to build a CSV parser by scratch! Rather, we can simply use ready-made modules. The one we’re interested in is called, you guessed it, CSV!
We do that like so:
import csv
Now, we can open the CSV file and print that data to the screen:
with open('c:\\Python\\Exercises.csv') as csv_file:
csv = csv.reader(csv_file, delimiter=',')
for row in csvFile:
print(row)
We can also split the data if we want to do fancy things with it:
for row in csvFile:
if lineCount > 0:
print(f'Perform {row[0]} for {row[1]} sets and reps, using {row[2]}.')
lineCount += 1
As you can see, this will simply run through the file, extract each piece of data, and then write it out in plain English.
Or, what if we want to pull out a specific row?
for row in csv:
if lineCount == 2:
print(f'Perform {row[0]} for {row[1]} sets and reps, using {row[2]}.')
lineCount += 1
Finally, what if we want to write to a CSV file? In that case, we can use the following code:
with open('C:\\Python\\Exercises2.csv', mode='w') as training_routine:
training_routine = csv.writer(trainingRoutine, delimiter=',', quotechar='"', quoting=csv.QUOTE_MINIMAL)
training_routine.writerow(['Exercise', 'Sets and Reps', 'Weight'])
training_routine.writerow(['Curls', '3 x 5', '25kg'])
training_routine.writerow(['Bench Press', '3 x 3', '120kg'])
How to open CSV files in Python manually
Remember that a CSV file is actually just a text document with a fancy formatting. That means that you actually don’t need to use a module if you want to know how to open CSV files in Python!
Also read: How to become a data analyst and prepare for the algorithm-driven future
You can quite simply write to a text file like so:
my_file = open("Exercises3.csv", "w+")
my_file.write("Exercise,Sets and Reps,Weight\nCurls,3 x 5,25kg\nBench Press,3 x 3,120kg")
my_file.close()
This actually makes it fairly simple to take the contents of a list, dictionary, or set, and turn them into a CSV! Likewise, we could read our files in a similar way and then simply break the data down by looking for commas. The main reason not to do this, is that some CSV files will use slightly different formating, which can cause problems when opening lots of different files. If you’re just working with your own files though, then you’ll have no trouble!
Also read: How to read a file in Python and more
And there you have it: now you know how to open CSV files in Python! And with that, you’ve dabbled in your first bit of JSON development and even a bit of data science. Feel proud!
What are you going to do with this knowledge? Let us know in the comments below! And if you want to learn more skills like this, then we recommend checking out our list of the best online Python courses. There you’ll be able to further your education with courses like the Python Data Science Bundle. You can get it for $37 right now, which is a huge saving on the usual $115.98!
For more developer news, features, and tutorials from Android Authority, don’t miss signing up for the monthly newsletter below!