Affiliate links on Android Authority may earn us a commission. Learn more.
Build your first basic Android game in just 7 minutes (with Unity)
Published onNovember 18, 2017
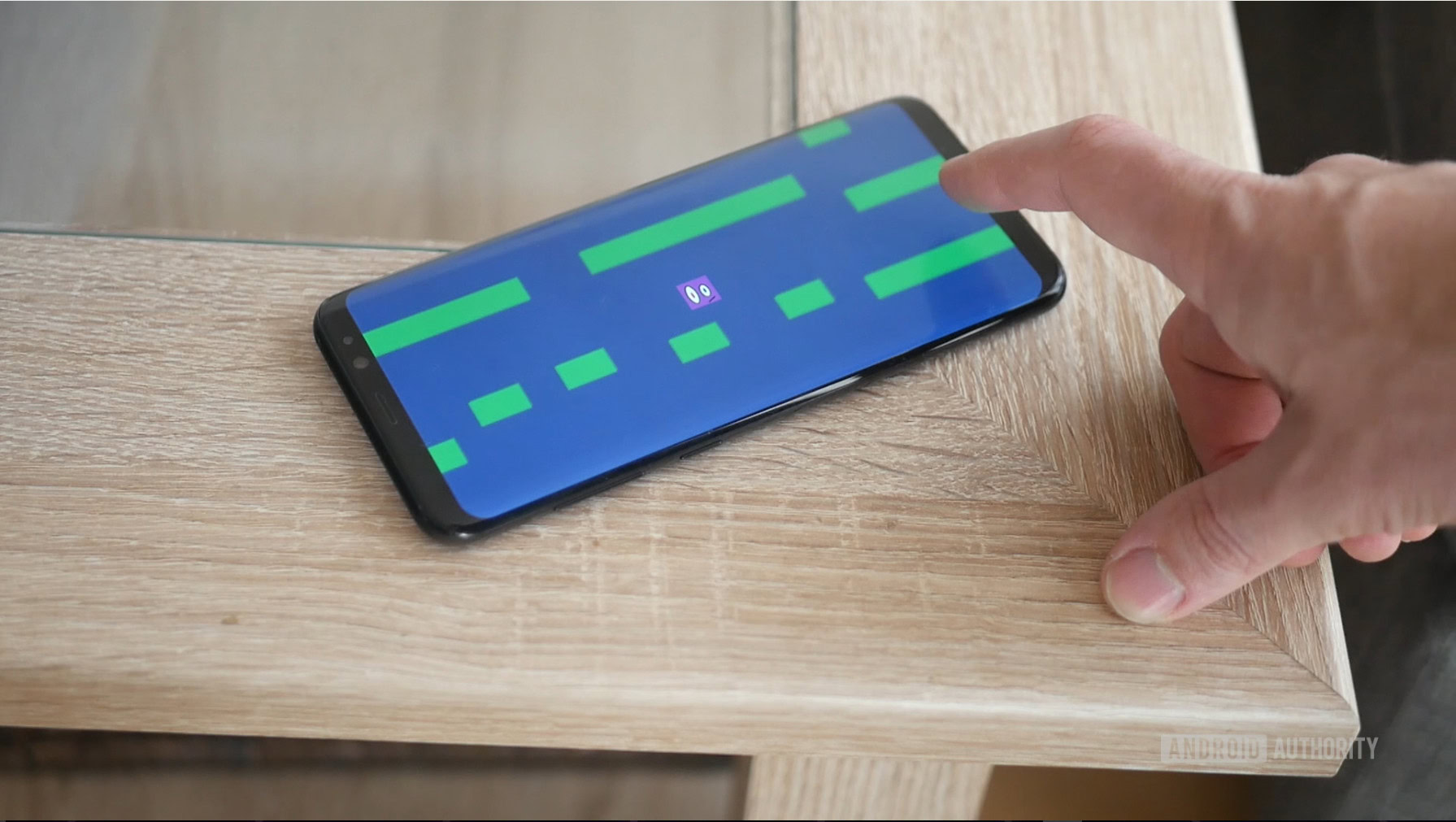
Making a fully working game for Android is much easier than you might think. The key to successful Android development— or any kind of development— is to know what you want to achieve and find the necessary tools and skills to do it. Take the path of least resistance and have a clear goal in mind.
When it comes to creating games, the best tool in my opinion is Unity. Yes, you can make a game in Android Studio, but unless you’re experienced with Java and the Android SDK it will be an uphill struggle. You’ll need to understand what classes do. You’ll need to use custom views. You’ll be relying on some additional libraries. The list goes on.
Unity is a highly professional tool that powers the vast majority of the biggest selling titles on the Play Store.
Unity on the other hand does most of the work for you. This is a game engine, meaning that all the physics and many of the other features you might want to use are already taken care of. It’s cross platform and it’s designed to be very beginner-friendly for hobbyists and indie developers.
At the same time, Unity is a highly professional tool that powers the vast majority of the biggest selling titles on the Play Store. There are no limitations here and no good reason to make life harder for yourself. It’s free, too!
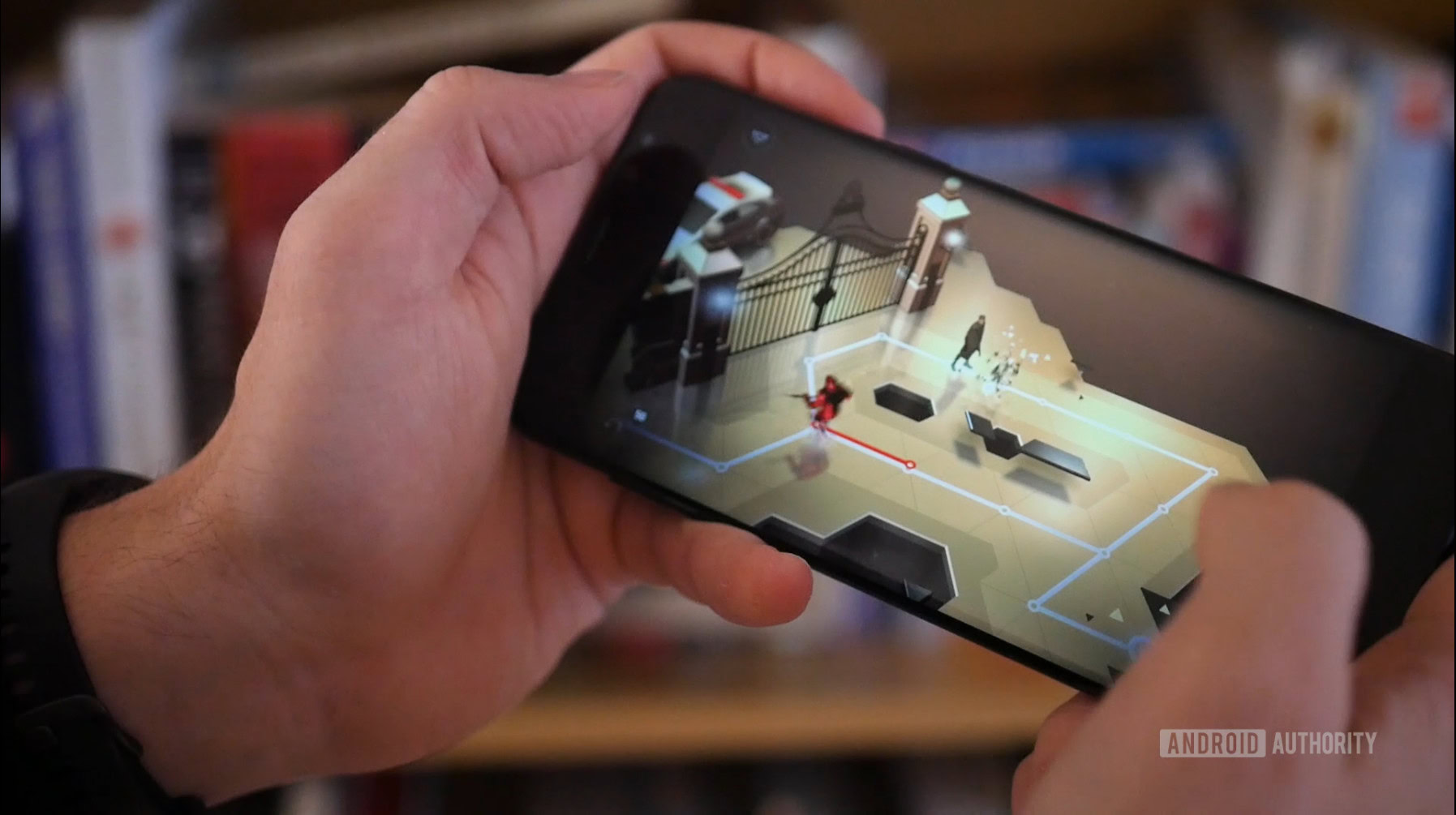
To demonstrate just how easy game development with Unity is, I’m going to show you how to make your first Android game in just 7 minutes.
No – I’m not going to explain how to do it in 7 minutes. I’m going to do it in 7 minutes. If you follow along too, you’ll be able to do the precise same thing!
Disclaimer: before we get started, I just want to point out that I’m slightly cheating. While the process of making the game will take 7 minutes, that presumes you’ve already installed Unity and gotten everything set up. But I won’t leave you hanging: you can find a full tutorial on how to do that over at Android Authority.
Adding sprites and physics
Start by double clicking on Unity to launch it. Even the longest journey starts with a single step.
Now create a new project and make sure you choose ‘2D’. Once you’re in, you’ll be greeted with a few different windows. These do stuff. We don’t have time to explain, so just follow my directions and you’ll pick it up as we go.
The first thing you’ll want to do is to create a sprite to be your character. The easiest way to do that is to draw a square. We’re going to give it a couple of eyes. If you want to be even faster still, you can just grab a sprite you like from somewhere.
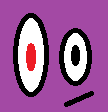
Save this sprite and then just drag and drop it into your ‘scene’ by placing it in the biggest window. You’ll notice that it also pops up on the left in the ‘hierarchy’.
Now we want to create some platforms. Again, we’re going to make do with a simple square and we’ll be able to resize this freehand to make walls, platforms and what have you.

There we go, beautiful. Drop it in the same way you just did.
We already have something that looks like a ‘game’. Click play and you should see a static scene for now.
We can change that by clicking on our player sprite and looking over to the right to the window called the ‘inspector’. This is where we change properties for our GameObjects.
Choose ‘Add Component’ and then choose ‘Physics 2D > RigidBody2D’. You’ve just added physics to your player! This would be incredibly difficult for us to do on our own and really highlights the usefulness of Unity.
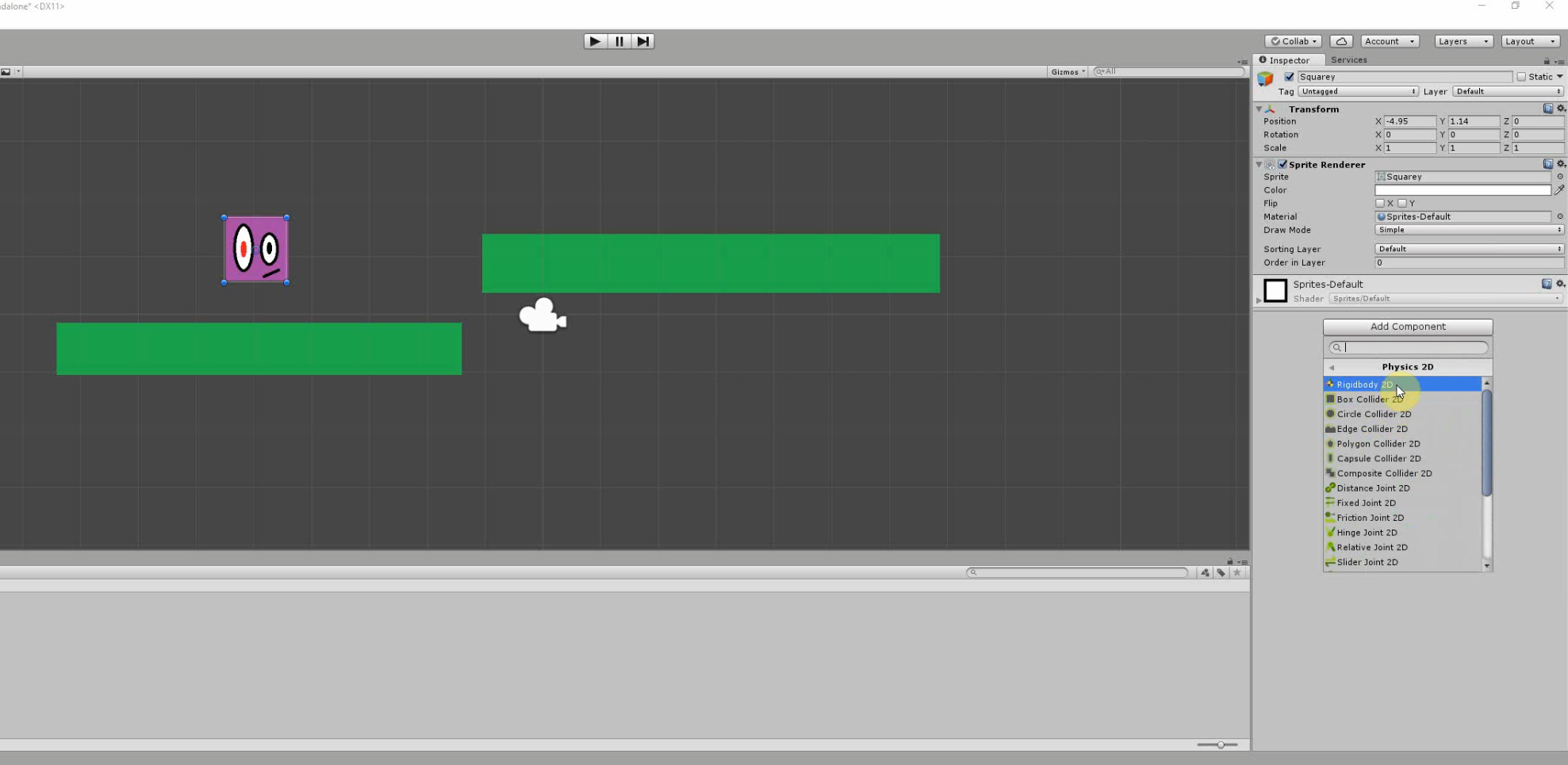
We also want to fix our orientation to prevent the character spinning and freewheeling around. Find ‘constraints’ in the inspector with the player selected and tick the box to freeze rotation Z. Now click play again and you should find your player now drops from the sky to his infinite doom.
Take a moment to reflect on just how easy this was: simply by applying this script called ‘RigidBody2D’ we have fully functional physics. Were we to apply the same script to a round shape, it would also roll and even bounce. Imagine coding that yourself and how involved that would be!
To stop our character falling through the floor, you’ll need to add a collider. This is basically the solid outline of a shape. To apply that, choose your player, click ‘Add Component’ and this time select ‘Physics 2D > BoxCollider2D’.
Take a moment to reflect on just how easy this was: simply by applying this script called ‘RigidBody2D’ we have fully functional physics.
Do the precise same thing with the platform, click play and then your character should drop onto the solid ground. Easy!
One more thing: to make sure that the camera follows our player whether they’re falling or moving, we want to drag the camera object that’s in the scene (this was created when you started the new project) on top of the player. Now in the hierarchy (the list of GameObjects on the left) you’re going to drag the camera so that it is indented underneath the player. The camera is now a ‘child’ of the Player GameObject, meaning that when the player moves, so too will the camera.
Your first script
We’re going to make a basic infinite runner and that means our character should move right across the screen until they hit an obstacle. For that, we need a script. So right click in the Assets folder down the bottom and create a new folder called ‘Scripts’. Now right click again and choose ‘Create > C# Script’. Call it ‘PlayerControls’.
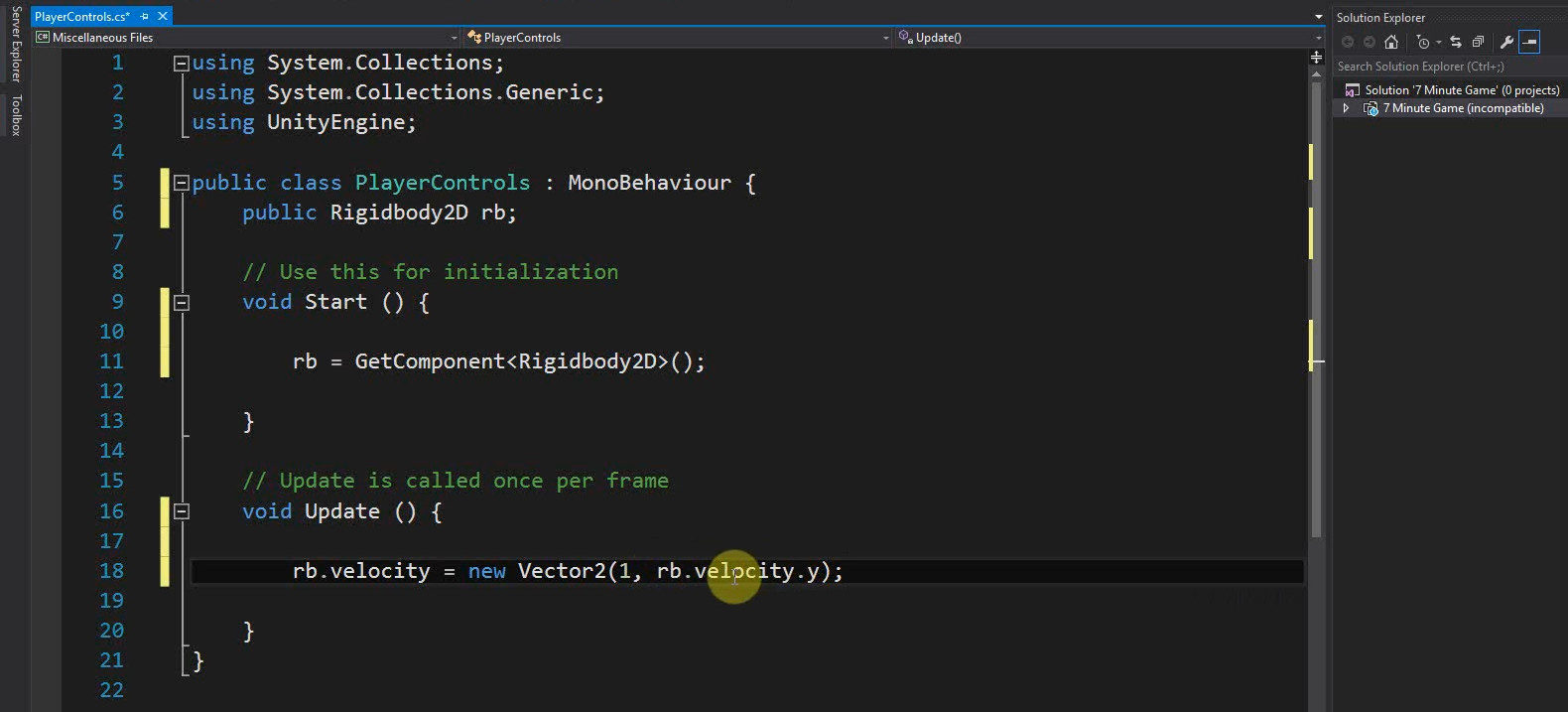
For the most part the scripts we create will define specific behaviors for our GameObjects.
Now double click on your new script and it will open up in Visual Studio if you set everything up correctly.
There’s already some code here, which is ‘boiler plate code’. That means that it’s code that you will need to use in nearly every script, so its ready-populated for you to save time. Now we’ll add a new object with this line above void Start():
public Rigidbody2D rb;
Then place this next line of code within the Start() method to find the rigidbody. This basically tells Unity to locate the physics attached to the GameObject that this script will be associated with (our player of course). Start() is a method that is executed as soon as a new object or script is created. Locate the physics object:
rb = GetComponent<Rigidbody2D>();
Add this inside Update():
rb.velocity = new Vector2(3, rb.velocity.y);
Update() refreshes repeatedly and so any code in here will run over and over again until the object is destroyed. This all says that we want our rigidbody to have a new vector with the same speed on the y axis (rb.velocity.y) but with the speed of ‘3’ on the horizontal axis. As you progress, you’ll probably use ‘FixedUpdate()’ in future.
Save that and go back to Unity. Click your player character and then in the inspector select Add Component > Scripts and then your new script. Click play, and boom! Your character should now move towards the edge of the ledge like a lemming.
Note: If any of this sounds confusing, just watch the video to see it all being done – it’ll help!
Very basic player input
If we want to add a jump feature, we can do this very simply with just one additional bit of code:
if (Input.GetMouseButtonDown(0)) {
rb.velocity = new Vector2(rb.velocity.x, 5);
}
This goes inside the Update method and it says that ‘if the player clicks’ then add velocity on the y axis (with the value 5). When we use if, anything that follows inside the brackets is used as a kind of true or false test. If the logic inside said brackets is true, then the code in the following curly brackets will run. In this case, if the player clicks the mouse, the velocity is added.
Android reads the left mouse click as tapping anywhere on the screen! So now your game has basic tap controls.
Finding your footing
This is basically enough to make a Flappy Birds clone. Throw in some obstacles and learn how to destroy the player when it touches them. Add a score on top of that.
If you get this down, no challenge will be too great in future
But we have a little more time so we can get more ambitious and make an infinite runner type game instead. The only thing wrong with what we have at the moment is that tapping jump will jump even when the player isn’t touching the floor, so it can essentially fly.
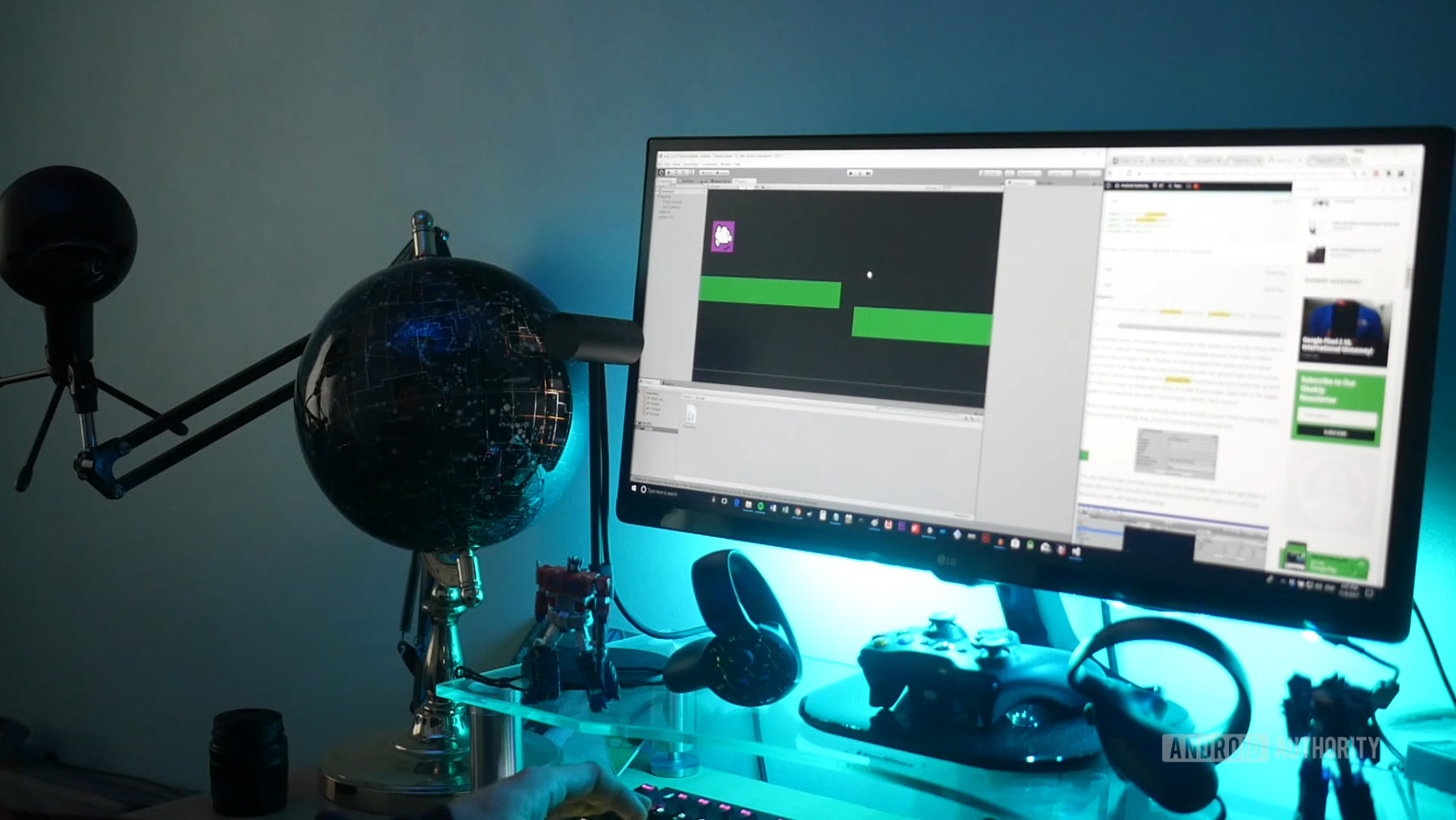
Remedying this gets a little more complex but this is about as hard as Unity gets. If you get this down, no challenge will be too great in future.
Add the following code to your script above the Update() method:
public Transform groundCheck;
public Transform startPosition;
public float groundCheckRadius;
public LayerMask whatIsGround;
private bool onGround;
Add this line to the Update method above the if statement:
onGround = Physics2D.OverlapCircle(groundCheck.position, groundCheckRadius, whatIsGround);
Finally, change the following line so that it includes && onGround:
if (Input.GetMouseButtonDown(0) && onGround) {
The entire thing should look like this:
public class PlayerControls : MonoBehaviour
{
public Rigidbody2D rb;
public Transform groundCheck;
public Transform startPosition;
public float groundCheckRadius;
public LayerMask whatIsGround;
private bool onGround;
void Start() {
rb = GetComponent<Rigidbody2D>();
}
void Update() {
rb.velocity = new Vector2(3, rb.velocity.y);
onGround = Physics2D.OverlapCircle(groundCheck.position, groundCheckRadius, whatIsGround);
if (Input.GetMouseButtonDown(0) && onGround) {
rb.velocity = new Vector2(rb.velocity.x, 5);
}
}
}
What we’re doing here is creating a new transform – a position in space – then we’re setting its radius and asking if it is overlapping a layer called ground. We’re then changing the value of the Boolean (which can be true or false) depending on whether or not that’s the case.
So, onGround is true if the transform called groundCheck is overlapping the layer ground.
If you click save and then head back to Unity, you should now see that you have more options available in your inspector when you select the player. These public variables can be seen from within Unity itself and that means that we can set them however we like.
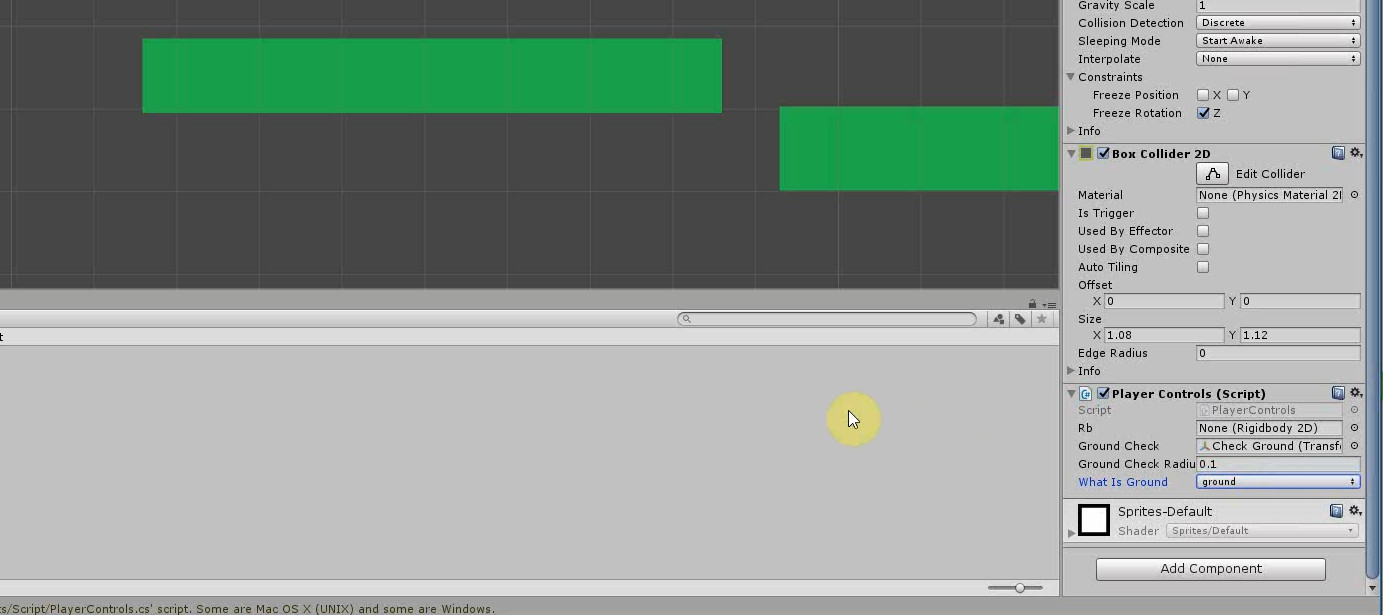
Right-click in the hierarchy over on the left to create a new empty object and then drag it so that it’s just underneath the player in the Scene window where you want to detect the floor. Rename the object ‘Check Ground’ and then make it a child of the player just as you did with the camera. Now it should follow the player, checking the floor underneath as it does.
Select the player again and, in the inspector, drag the new Check Ground object into the space where it says ‘groundCheck’. The ‘transform’ (position) is now going to be equal to the position of the new object. While you’re here, enter 0.1 where it says radius.
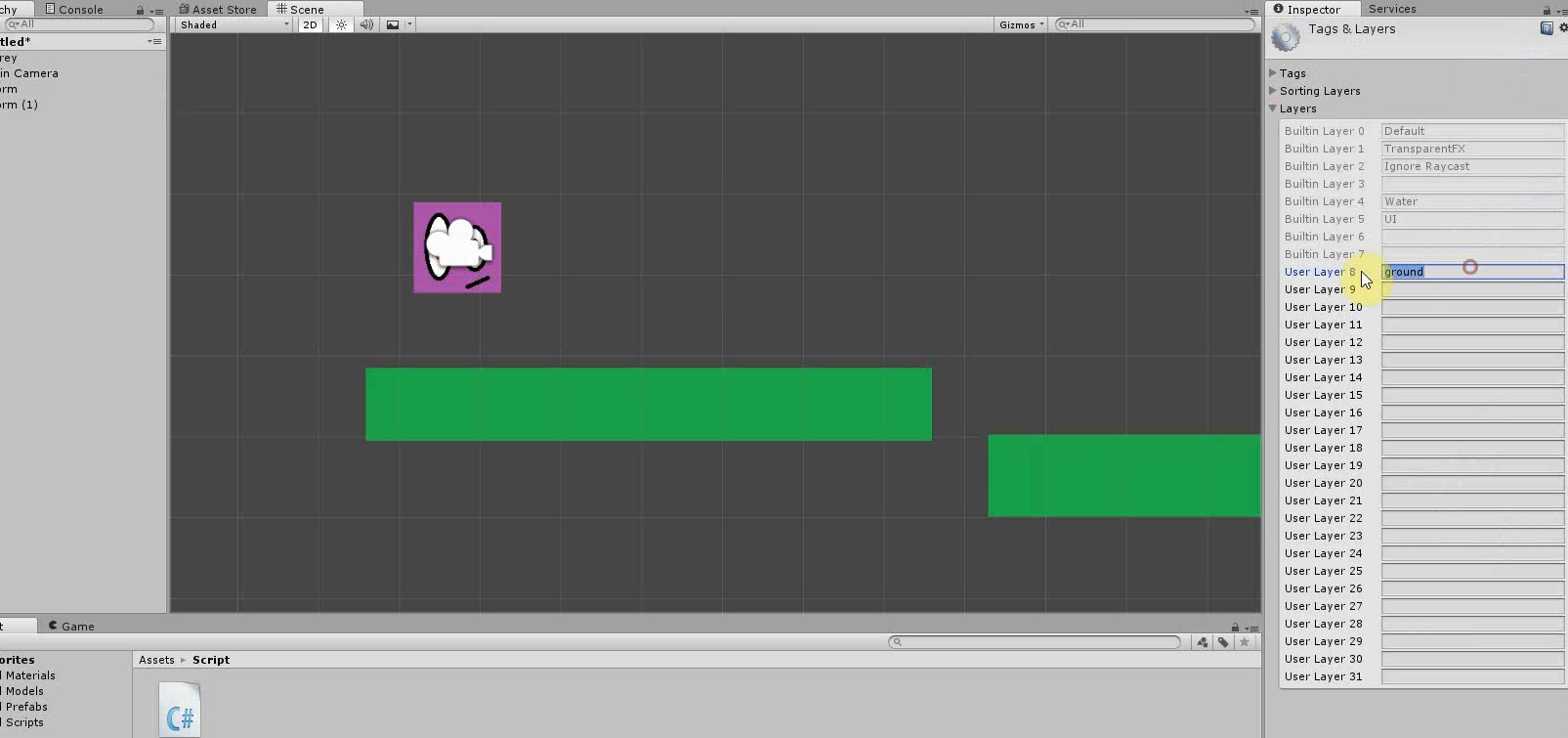
Finally, we need to define our ‘ground’ layer. To do this, select the terrain you created earlier, then up in the top right in the inspector, find where it says ‘Layer: Default’. Click this drop down box and choose ‘Add Layer’.
Now click back and this time select ‘ground’ as the layer for your platform (repeat this for any other platforms you have floating around). Finally, where it says ‘What is Ground’ on your player, select the ground layer as well.
You’re now telling your player script to check if the small point on the screen is overlapping anything matching that layer. Thanks to that line we added earlier, the character will now only jump when that is the case.
And with that, if you hit play, you can enjoy a pretty basic game requiring you to click to jump at the right time.
With that, if you hit play you can enjoy a pretty basic game requiring you to click to jump at the right time. If you set your Unity up properly with the Android SDK, then you should be able to build and run this and then play on your smartphone by tapping the screen to jump.
The road ahead
Obviously there’s a lot more to add to make this a full game. The player should to be able to die and respawn. We’d want to add extra levels and more.
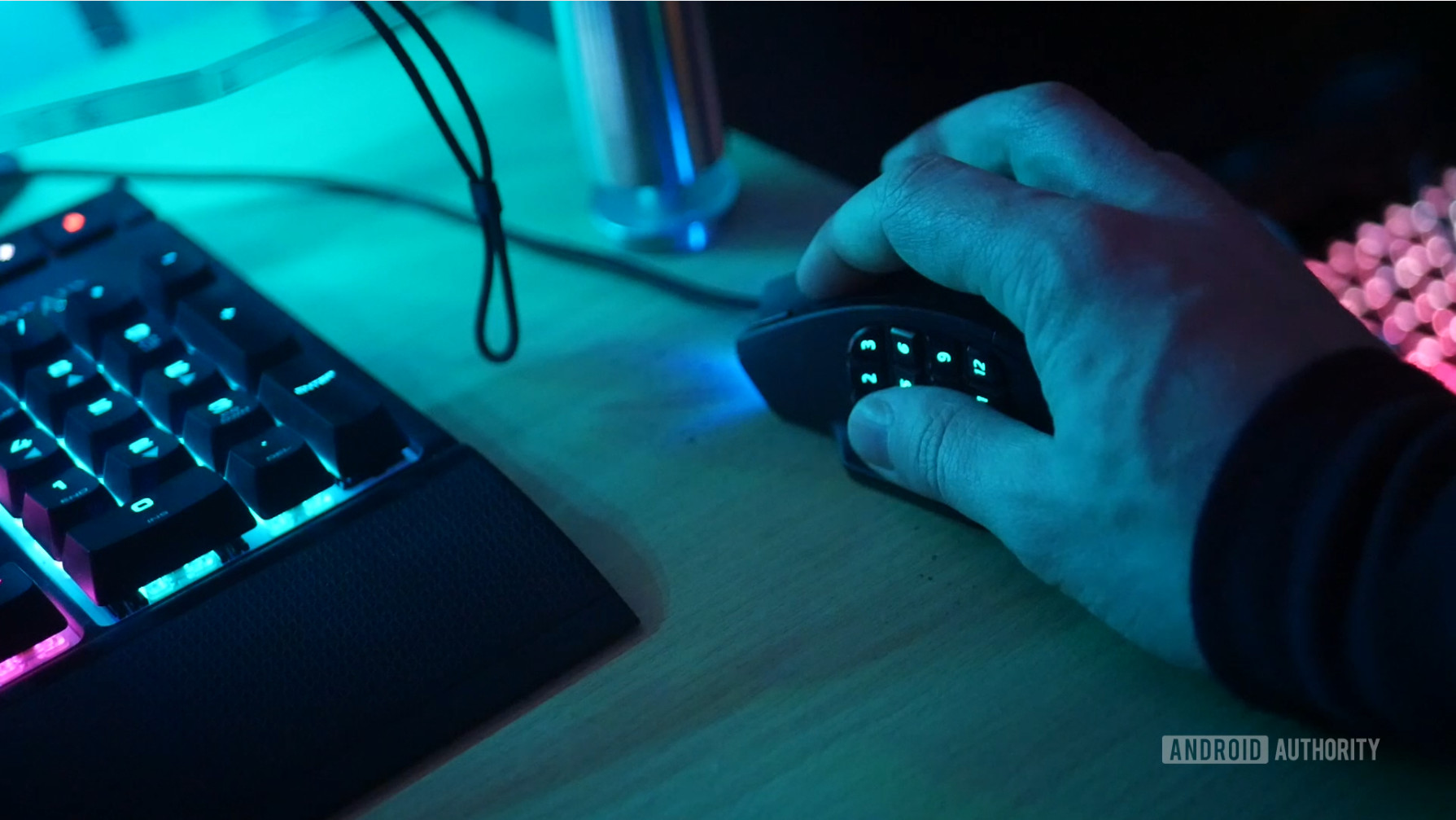
My aim here was to show you how quickly you can get something basic up and running. Following these instructions, you should have been able to build your infinite runner in no time simply by letting Unity handle the hard stuff, like physics.
If you know what you want to build and do your research, you don’t need to be a coding wizard to create a decent game!