Affiliate links on Android Authority may earn us a commission. Learn more.
Sending Android push notifications with Firebase Cloud Messaging
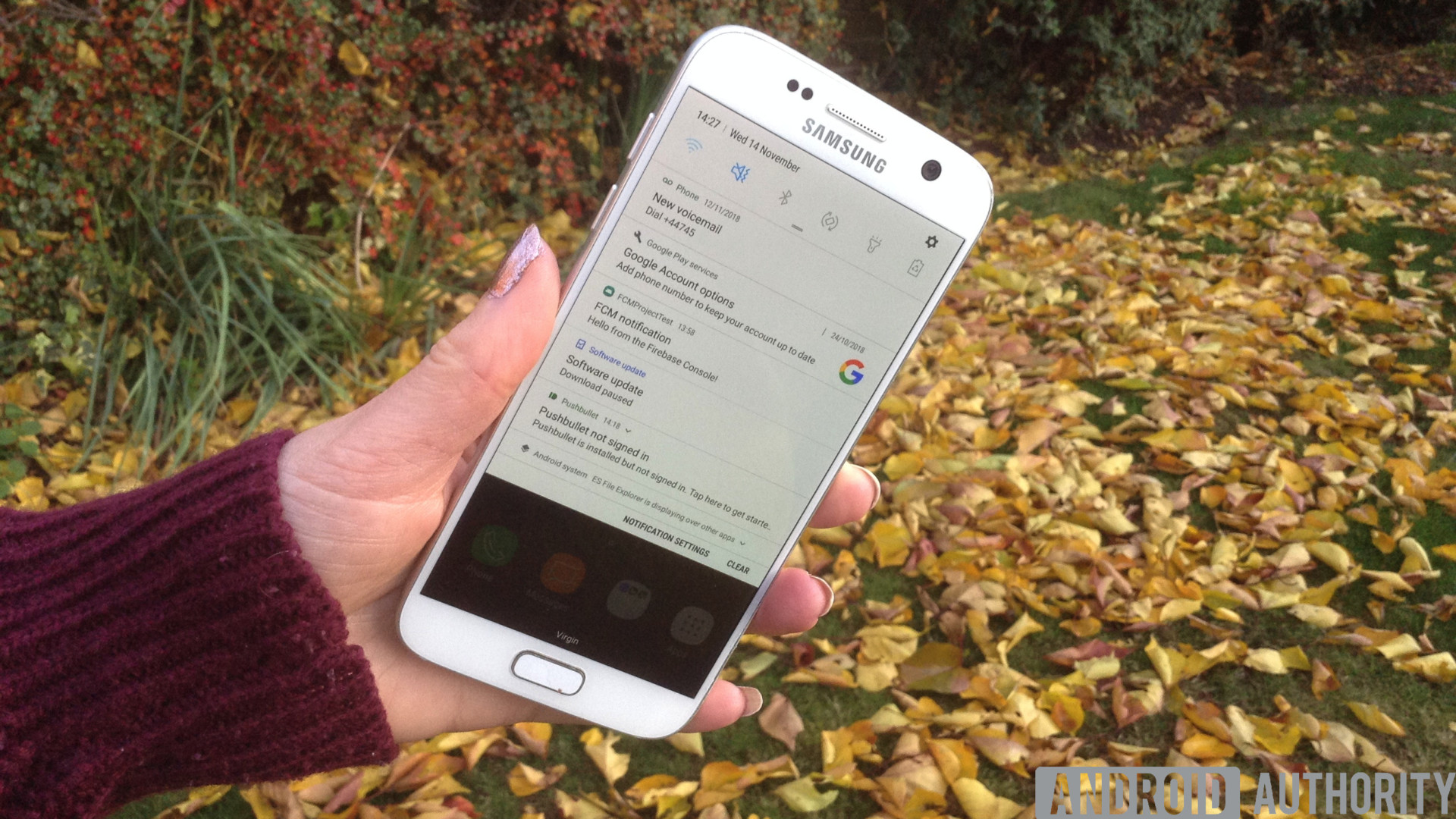
If your app is going to be a success, then you need to hold the user’s interest over time, and notifications are an important way to keep your audience engaged.
By presenting the user with a timely, relevant notification at exactly the right moment, you can recapture their wandering interest, and pull them back into your app.
Android has various classes for creating notifications on-device, but often the most compelling notifications are triggered externally. If you’ve developed a mobile game, then you could spark the user’s interest by notifying them about a new themed event that’s just about to start, or congratulating them on being hand-picked to participate in an exclusive in-game challenge.
In this article, I’ll show you how to quickly and easily send notifications from an external server, using Firebase Cloud Messaging (FCM). Once we’ve added FCM support to a project and sent a few test notifications, I’ll show you how to create more engaging notifications, by using the Firebase Console to target specific sections of your audience, including sending a notification to a single device, using their unique token ID.
What is Firebase Cloud Messaging?
FCM is a free, cross-platform messaging solution that lets you send push notifications to your audience, without having to worry about the server code. By using FCM alongside Firebase’s Notifications Composer (as seen in the following screenshot), you can create notifications that target very specific sections of your user base, often without having to write any special code.
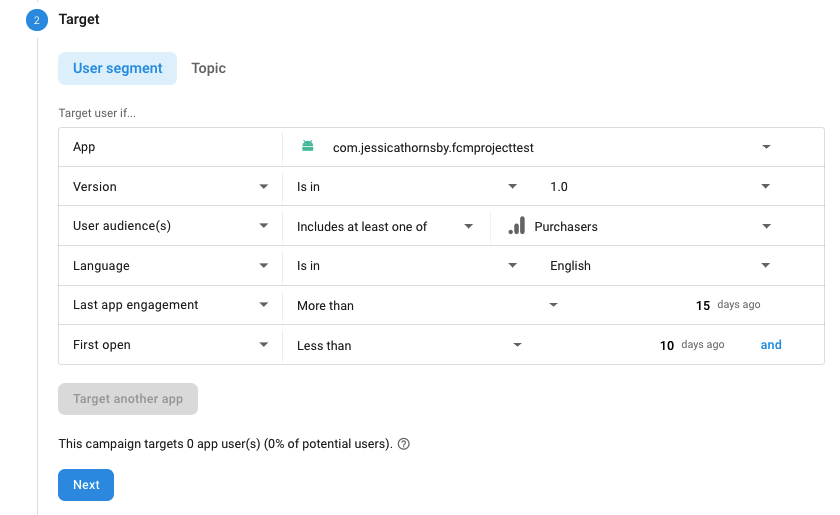
Although it’s beyond the scope of this tutorial, you can also use FCM for upstream notifications, where FCM receives a message from the client application, or to notify your app when there’s new data available for it to download. In this way, you can ensure communication between your app server and client app occurs only when necessary, which is much more efficient than the client app contacting the server at regular intervals, on the off-chance there might be some new data available.
Since FCM is part of Firebase, it also plays nicely with other Firebase services. Once you’ve mastered the FCM essentials, you may want to use A/B Testing to identify which notifications are the most effective, or use Firebase Predictions to apply powerful machine learning to all of the analytics data generated from your various FCM campaigns.
FCM supports two types of messages:
- Notification messages. The client application will behave differently depending on whether it’s in the background or the foreground when it receives the FCM message. If your app is in the background, then the Firebase SDK will automatically process the message and display it as a notification in the device’s system tray. Since the Android system builds the notification for you, this is one of the easiest ways to send push notifications to your users. If your app receives an FCM message while it’s in the foreground, then the system won’t handle this notification automatically, leaving you to process the message in your app’s onMessageReceived() callback. We’ll be exploring onMessageReceived() later in this tutorial, but for now just be aware that if your app receives a message while it’s in the foreground, then by default this message won’t be displayed to the user.
- Data messages. Unlike notification messages, you can use data messages to send custom data elements to the client application. However, FCM does place a 4KB limit on these data messages, so if your payload exceeds 4KB then you’ll need to fetch the additional data using WorkManager or the JobScheduler API.
In this tutorial, we’ll be focusing on notification messages.
What about Google Cloud Messaging?
If you’re using the Google Cloud Messaging (GCM) server and client APIs, then there’s some bad news: this service has already been deprecated and Google are planning to turn off “most” GCM services in April 2019. If you’re still using GCM, then you should start migrating your projects to FCM now, and must have completed your migration by April 2019.
Adding Firebase to your Android project
Let’s see how easy it is to add basic FCM support to your app, and then use it to send push notifications to your users.
Since FCM is a Firebase service, you’ll need to add Firebase to your app:
- Head over to the Firebase Console.
- Select “Add project,” and give your project a name.
- Read the terms and conditions. If you’re happy to proceed, then select “I accept…” followed by “Create project.”
- Select “Add Firebase to your Android app.”
- Enter your project’s package name, and then click “Register app.”
- Select “Download google-services.json.”
- In Android Studio, drag and drop the google-services.json file into your project’s “app” directory.
- Open your project-level build.gradle file and add the following:
classpath 'com.google.gms:google-services:4.0.1'
- Open your app-level build.gradle file, and add the Google services plugin, plus the dependencies for Firebase Core and FCM:
//Add the Google services plugin//
apply plugin: 'com.google.gms.google-services'
…
…
…
dependencies {
implementation fileTree(dir: 'libs', include: ['*.jar'])
//Add Firebase Core//
implementation 'com.google.firebase:firebase-core:16.0.1'
//Add FCM//
implementation 'com.google.firebase:firebase-messaging:17.3.4'
- When prompted, sync your changes.
- Next, you need to let the Firebase Console know that you’ve successfully added Firebase to your project. Install your app on either a physical Android smartphone or tablet, or an Android Virtual Device (AVD).
- Back in the Firebase Console, select “Run app to verify installation.”
- Once Firebase has detected your app, you’ll see a “Congratulations” message. Select “Continue to the console.”
Sending your first push notification with Firebase
And that’s it! You can now send a push notification to your users, and that notification will appear in the device’s system tray (for now, let’s assume your app isn’t in the foreground when the message is delivered).
You create FCM notifications using the Notifications Composer, which is available via the Firebase Console:
- Make sure your app is installed and running in the background, and that your device has an active Internet connection.
- In the Firebase Console, select “Cloud Messaging” from the left-hand menu.

- Select “Send your first message.”
- Give your message a title and some body text, and then click “Next.”
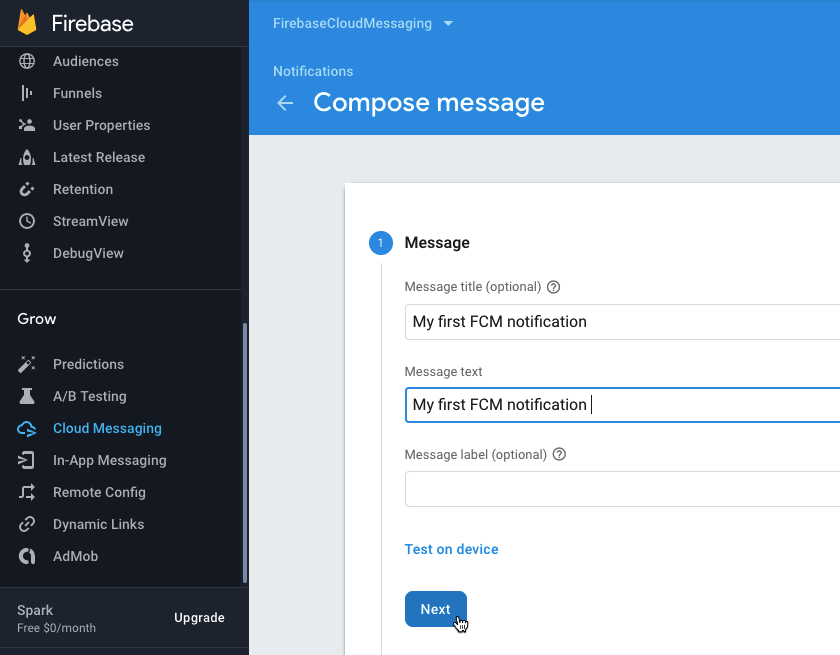
- Open the “Select app” dropdown, and choose your application from the list. This section also includes some advanced options that you can use to create targeted notifications, based on factors such as app version, the device’s locale, and the last time the user engaged with your app. We won’t be using any of these options in our test notification, but if you want to see what’s available, then select “and…” and explore the subsequent dropdown.

- Once you’ve finished editing this section, click “Next.”
- Assuming you want to send this message immediately, open the “Send to eligible users” dropdown and select “Now.”
- In the bottom-right of the screen, click “Publish.”
- Check all the information in the subsequent popup, and if you’re happy to proceed then select “Publish.”
After a few moments, all the client devices that you targeted should receive this notification in their system tray.
Most of the time, FCM notifications will be delivered immediately, but occasionally it may take a few minutes for a message to arrive, so don’t panic if your notification is delayed.
Setting some goals: Notification conversion events
When creating a notification, you’ll usually have a goal in mind – whether that’s driving users back to your app, convincing them to splash out on an in-app purchase, or simply opening your notification.
You can assign a goal to your notification, using the Notification Composer, and then track that notification’s performance in the FCM reporting dashboard.
To set a goal, click to expand the Navigation Composer’s “Conversion events” section, then open the accompanying dropdown and choose from the available conversion events.
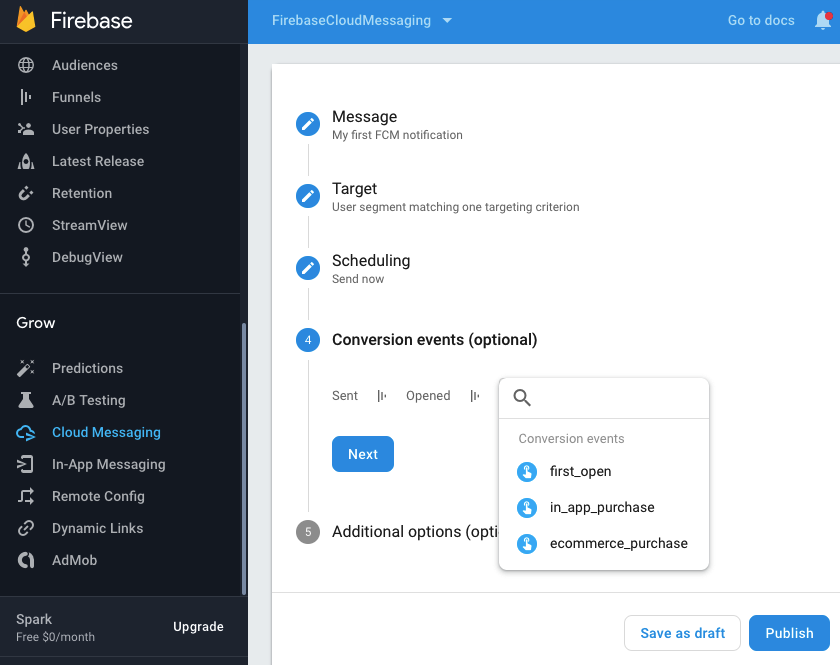
Was your notification a success?
After sending a notification message, you can analyze its performance in the FCM reporting dashboard, which should load automatically every time you send a new message, or you can access the dashboard directly.
Even if you didn’t set any explicit conversion goals, you can still gauge whether users are acting on your notifications, by comparing the number of messages delivered, to the number of messages opened.
You can also select any message in this list, to see the send, open and conversion data as a graph. If you set any conversion goals, then this is also where you’ll find the statistics relating to those goals.
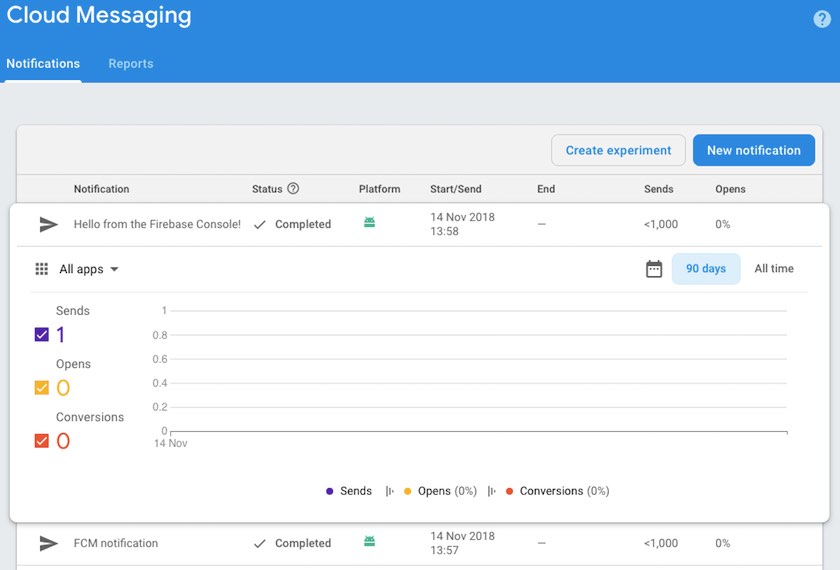
What if my app is in the foreground?
FCM notifications behave differently depending on the state of the client application.
By default, your app won’t display any FCM messages it receives while it’s in the foreground, so when you send a message there’s no guarantee your users will actually see that message.
To act on the messages your app receives while it’s in the foreground, you’ll need to extend the FirebaseMessagingService, override the onMessageReceived method, and then retrieve the message’s content using either getNotification or getData, depending on whether you’re working with data or notification messages, or both.
Create a new Java class named “MyFirebaseMessagingService” and then add the following:
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onMessageReceived(RemoteMessage message) {
super.onMessageReceived(remoteMessage);
You’ll also need to create a notification object. This is your chance to customize your notification, for example choosing the sound that should play whenever the user receives this notification, or applying a custom notification icon. You’ll also need to retrieve the content from the data or notification message, for example:
NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(this, "channel_id")
.setContentTitle(remoteMessage.getNotification().getTitle())
.setContentText(remoteMessage.getNotification().getBody())
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.setStyle(new NotificationCompat.BigTextStyle())
.setSound(RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION))
.setSmallIcon(R.mipmap.ic_launcher)
.setAutoCancel(true);
NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
notificationManager.notify(0, notificationBuilder.build());
}
}
Once you’ve created your service, don’t forget to add it to your Manifest:
<service android:name=".MyFirebaseMessagingService">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
Now, everytime your app receives an FCM message while it’s in the foreground, it’ll be delivered to the onMessageReceived() handler and your app will then take the action defined by you, such as posting the notification or updating your app’s content.
More engaging notifications: Targeting your users
Up until now, we’ve been sending the same notification to our entire user base, but notifications are far more engaging when they’re targeted at specific users.
You can use the Notification Composer to send different notifications, to different parts of your user base. Head over to the Notification Composer and create your notification as normal, but in the “Target” section, click “and.” This gives you access to a new dropdown, containing the following options:
- Version. This allows you to target, or exclude, devices running specific versions of your application. For example, you might send notifications to people who are running the free version, encouraging them to upgrade to your app’s Premium version.
- Language. You can use this setting to target or exclude the different languages and locales that your application supports, such as creating notifications that are tailored for different time zones or languages.
- User audience(s). This lets you target, or exclude different sections of your audience. For example, you could use this setting to tempt people who have a history of making in-app purchases, by offering them a discount or drawing their attention to all the amazing new in-app products you’ve just released.
- User property. If you’ve setup Firebase Analytics, then you’ll have access to a range of information about your audience, via user properties. You can use these properties in combination with FCM, to send targeted notifications to very specific sections of your user base, such as people within the 25-34 age range who are interested in sports.
- Prediction. If you’ve setup Firebase Predictions, then you can target users based on how likely they are to engage in a particular behavior over the next 7 days. For example, if Predictions warns that someone is likely to disengage from your mobile game, then you could use FCM to invite them to take part in a new quest, or to send them some in-game currency.
- Last app engagement. If a user hasn’t launched your app in a while, then you can use this setting to send them a few notifications, just to remind them about all the great content that your app has to offer.
- First open. This lets you send notifications based on the first time the user opened your app, for example you might help new users get up to speed by sending them notifications containing useful tips and advice.
Targeting a single device with registration tokens
We’ve already seen how to send targeted notifications based on factors such as the user’s age, interests, and the last time they engaged with your app, but you can get even more specific. In this final section, I’ll show you how to send an FCM notification to a single device.
When the user launches your app for the first time, the FCM SDK generates a registration token for that client app instance. You can use FirebaseInstanceId.getInstance().getInstanceId() to capture this registration token, and then send a notification to this specific token.
Note that in a real-world project, you’d typically capture a token by sending it to your app server and storing it using your preferred method, but to help keep things straightforward I’ll simply be printing this token to Android Studio’s Logcat.
Here’s my completed MainActivity:
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.annotation.NonNull;
import android.util.Log;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.iid.FirebaseInstanceId;
import com.google.firebase.iid.InstanceIdResult;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
FirebaseInstanceId.getInstance().getInstanceId()
.addOnCompleteListener(new OnCompleteListener<InstanceIdResult>() {
@Override
public void onComplete(@NonNull Task<InstanceIdResult> task) {
if (!task.isSuccessful()) {
//To do//
return;
}
// Get the Instance ID token//
String token = task.getResult().getToken();
String msg = getString(R.string.fcm_token, token);
Log.d(TAG, msg);
}
});
}
}
Open your strings.xml file and create the “fcm_token” string resource that we’re referencing in our MainActivity:
<string name="fcm_token">FCM Token: %s</string>
You can now retrieve your device’s unique token:
- Install your project on the connected Android device, or AVD.
- Open Android Studio’s Logcat, by selecting the “Logcat” tab (where the cursor is positioned in the following screenshot).
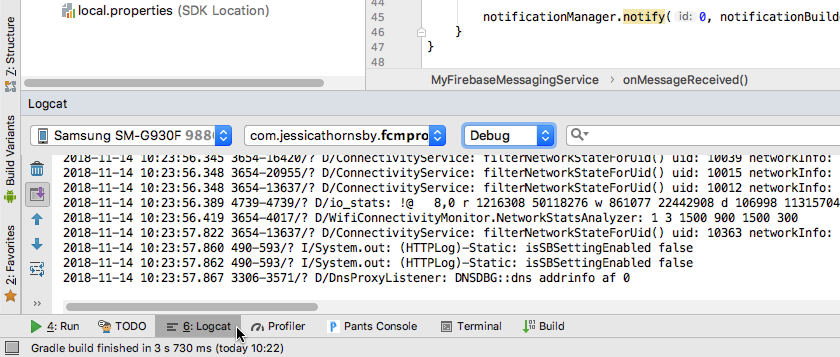
- Your device’s token will be printed to the “Debug” section of Logcat, so open the dropdown and select “Debug.”
Depending on the amount of information in your Logcat, it may be difficult to spot the line you’re looking for. If you’re struggling, then run a search for the word “token,” or try closing and then relaunching the app.
Once you’ve retrieved the token, you can use it to send a push notification to this particular device:
- Head over to the Firebase Console and select your project from the dropdown menu, if you haven’t already.
- Choose “Cloud Messaging” from the left-hand menu.
- Click the “New notification” button.
- Enter you message title and text, as normal, but then click “Test on device.”
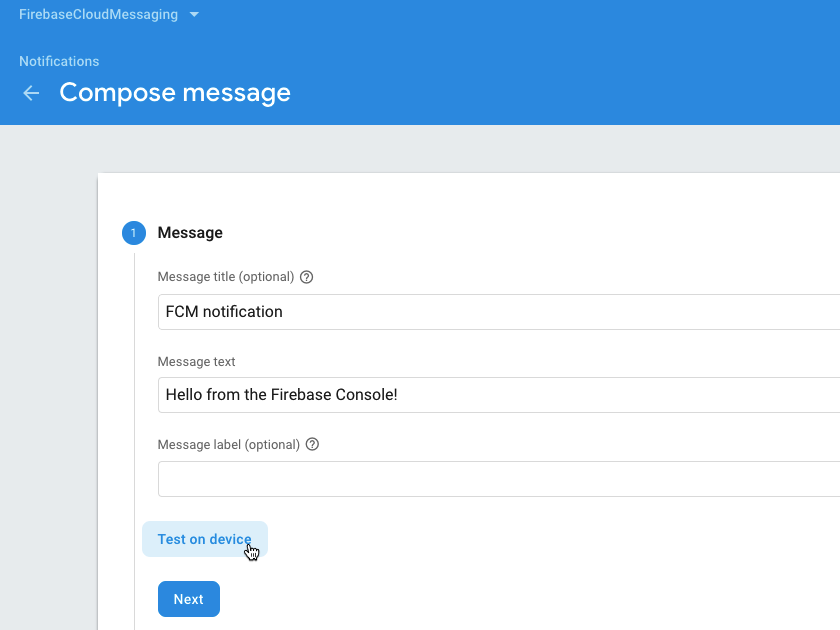
- Copy/paste your token into the “Add an instance…” field, and then click the little blue “+’ icon that appears.
- Select the token’s accompanying checkbox.
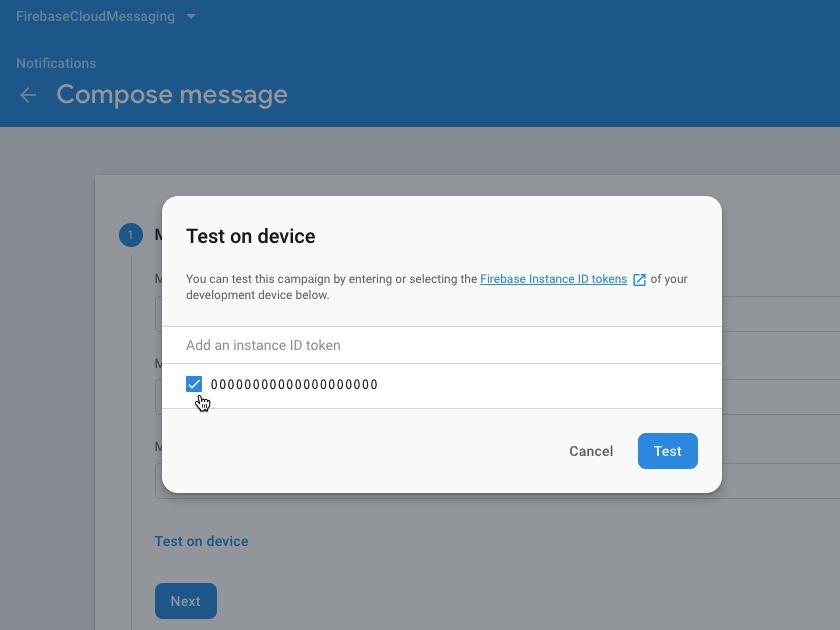
- Click “Test.”
This notification will now appear on the targeted client device only.
Wrapping up
In this article, I showed you how to send Android push notifications, using Firebase Cloud Messaging, and how to create notifications that target different sections of your user base.
Are you going to use FCM in your own Android projects? Let us know in the comments below!