Affiliate links on Android Authority may earn us a commission. Learn more.
Java tutorial for beginners: Write a simple app with no previous experience
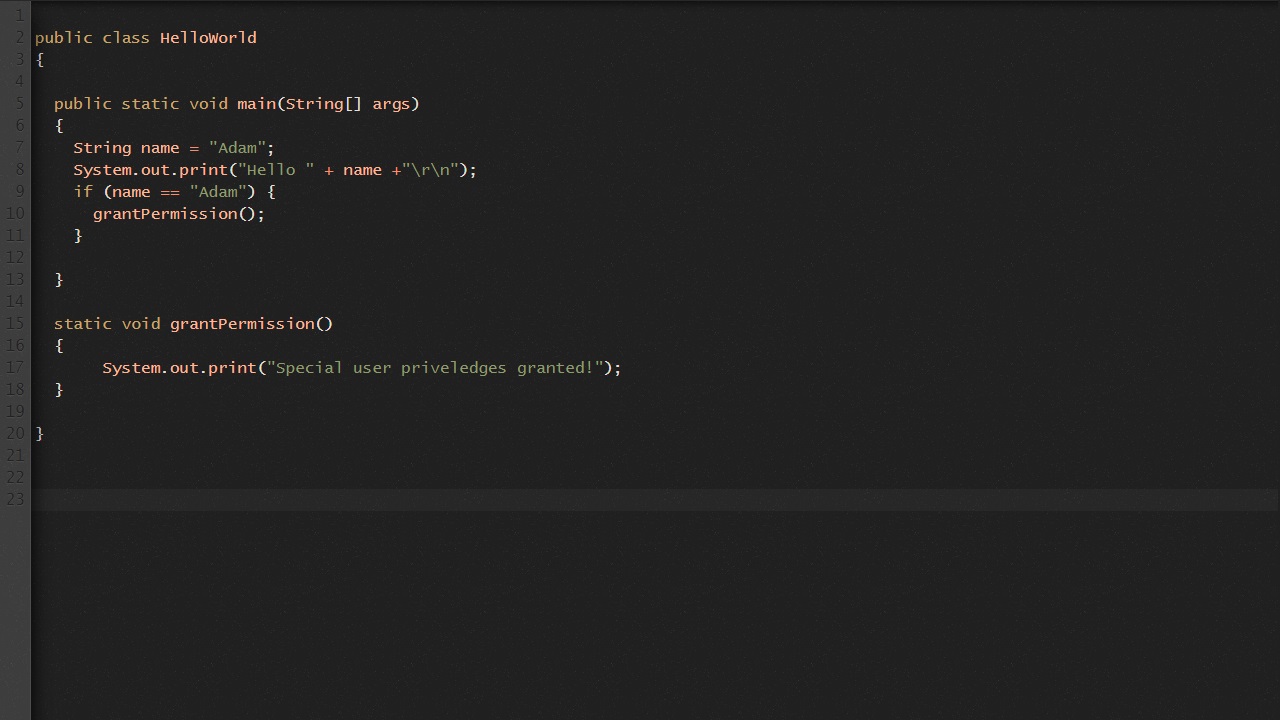
Java is one of the most in-demand programming languages in the world and one of the two official programming languages used in Android development (the other being Kotlin). Developers familiar with Java are highly employable and capable of building a wide range of different apps, games, and tools. In this Java tutorial for beginners, you will take your first steps to become one such developer! We’ll go through everything you need to know to get started, and help you build your first basic app.
What is Java?
Java is an object-oriented programming language developed by Sun Microsystems in the 1990s (later purchased by Oracle).
“Object oriented” refers to the way that Java code is structured: in modular sections called “classes” that work together to deliver a cohesive experience. We’ll discuss this more later, but suffice to say that it results in versatile and organized code that is easy to edit and repurpose.
Java is influenced by C and C++, so it has many similarities with those languages (and C#). One of the big advantages of Java is that it is “platform independent.” This means that code you write on one machine can easily be run on a different one. This is referred to as the “write once, run anywhere” principle (although it is not always that simple in practice!).
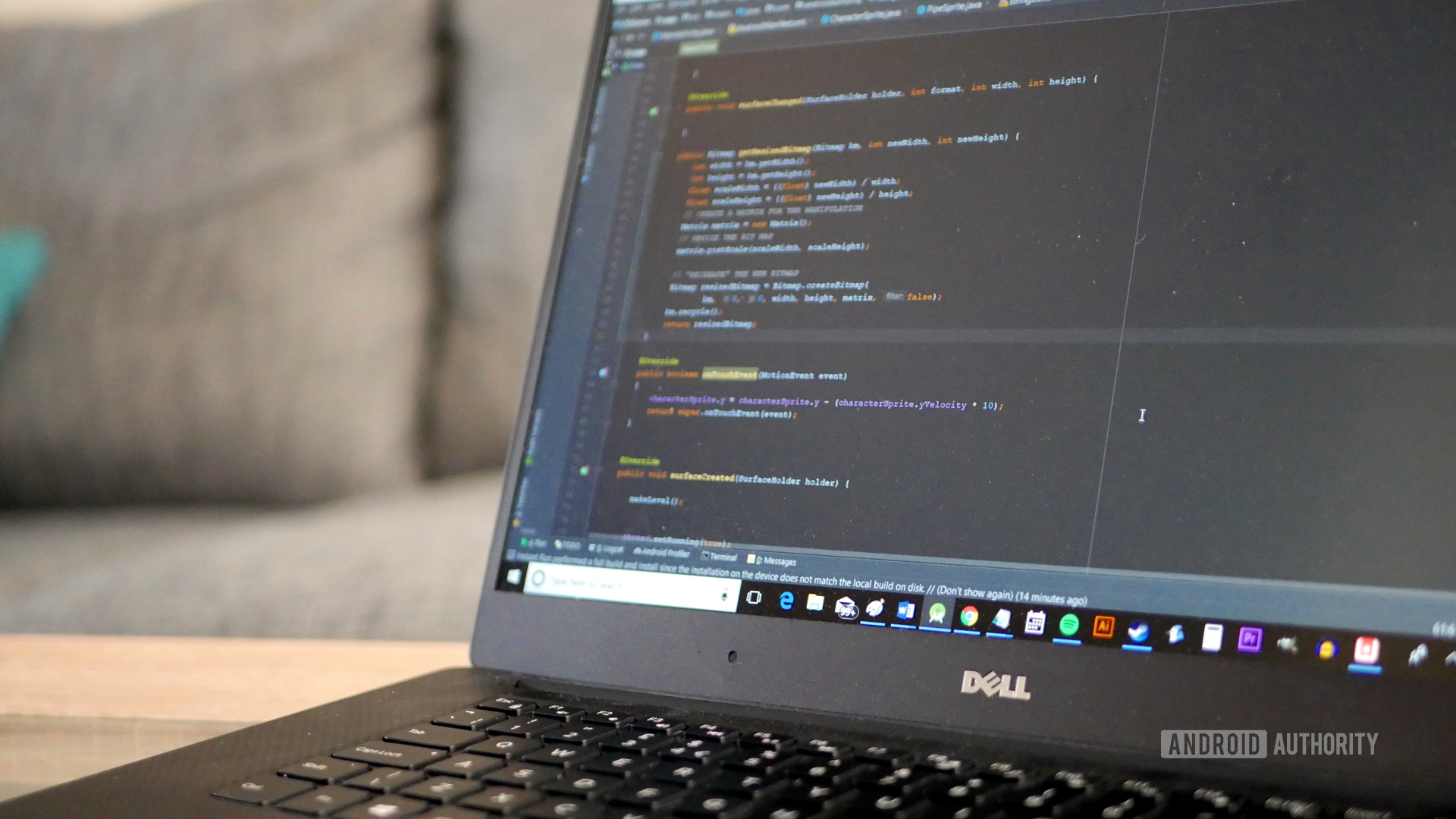
To run and use Java, you need three things:
- The JDK – Java Development Kit
- The JRE – The Java Runtime Environment
- The JVM – The Java Virtual Machine
The Java Virtual Machine ensures that your Java applications have access to the minimum resources they need to run. It is thanks to the JVM that Java code is so easily run across platforms.
The Java Runtime Environment provides a “container” for those elements and your code to run in. The JDK is the “compiler” that interprets the code itself and executes it. The JDK also contains the developer tools you need to write Java code (as the name suggests!).
The good news is that developers need only concern themselves with downloading the JDK – as this comes packed with the other two components.
How to get started with Java programming
If you plan on developing Java apps on your desktop computer, then you will need to download and install the JDK.
You can get the latest version of the JDK directly from Oracle. Once you’ve installed this, your computer will have the ability to understand and run Java code. However, you will still need an additional piece of software in order to actually write the code. This is the “Integrated Development Environment” or IDE: the interface used by developers to enter their code and call upon the JDK.
When developing for Android, you will use the Android Studio IDE. This not only serves as an interface for your Java (or Kotlin) code, but also acts as a bridge for accessing Android-specific code from the SDK. For more on that, check out our guide to Android development for beginners.
For the purposes of this Java tutorial, it may be easier to write your code directly into a Java compiler app. You can download these for Android and iOS, or even find web apps that run in your browser. These tools provide everything you need in one place and let you start testing code.
I recommend compilejava.net.
How easy is it to learn Java programming?
If you’re new to Java development, then you may understandably be a little apprehensive. How easy is Java to learn?
This question is somewhat subjective, but I would personally rate Java as being on the slightly harder end of the spectrum. While easier than C++ and is often described as more user-friendly, it certainly isn’t quite as straightforward as options like Python or BASIC which sit at the very beginner-friendly end of the spectrum. For absolute beginners who want the smoothest ride possible, I would recommend Python as an easier starting point.
C# is also a little easier as compared with Java, although they are very similar.
Of course, if you have a specific goal in mind – such as developing apps for Android – it is probably easiest to start with a language that is already supported by that platform.
Java has its quirks, but it’s certainly not impossible to learn and will open up a wealth of opportunities once you crack it. And because Java has so many similarities with C and C#, you’ll be able to transition to those languages without too much effort.
What is Java syntax?
Before we dive into the meat of this Java for beginners tutorial, it’s worth taking a moment to examine Java syntax.
Java syntax refers to the way that things are written. Java is very particular about this, and if you don’t write things in a certain way, then your code won’t run!
I actually wrote a whole article on Java syntax for Android development, but to recap on the basics:
- Most lines should end with a semicolon “;”
- The exception is a line that opens up a new code block. This should end with an open curly bracket “{“. Alternatively, this open bracket can be placed on a new line beneath the statement. Code blocks are chunks of code that perform specific, separate tasks.
- Code inside the code block should then be indented to set it apart from the rest.
- Open code blocks should be closed with a closing curly bracket “}”.
- Comments are lines preceded by “//”
If you hit “run” or “compile” and you get an error, there is a high chance it’s because you missed off a semi-colon somewhere!
You will never stop doing this and it will never stop being annoying. Joy!
With that out of the way, we can dive into the Java tutorial proper!
Java basics: your first program
Head over to compilejava.net and you will be greeted by an editor with a bunch of code already in it.
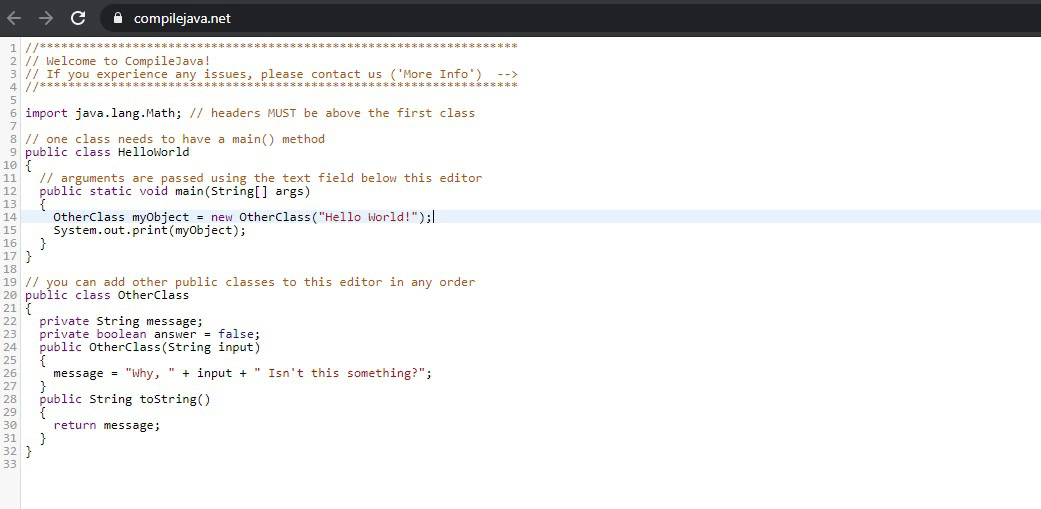
(If you would rather use a different IDE or app that’s fine too! Chances are your new project will be populated by similar code.)
Delete everything except the following:
public class HelloWorld
{
public static void main(String[] args)
{
}
}
This is what we refer to “in the biz” (this Java tutorial is brought to you by Phil Dunphy) as “boilerplate code.” Boilerplate is any code that is required for practically any program to run.
The first line here defines the “class” which is essentially a module of code. We then need a method within that class, which is a little block of code that performs a task. In every Java program, there needs to be a method called main, as this tells Java where the program starts.
You won’t need to worry about the rest until later. All we need to know for this Java tutorial right now is that the code we actually want to run should be placed within the curly brackets beneath the word “main.”
Place the following statement here:
System.out.print("Hello world!");
This statement will write the words “Hello world!” on the screen. Hit “Compile & Execute” and you’ll be able to see it in action! (It’s a programming tradition to make your first program in any new language say “Hello world!” Programmers are a weird bunch.)
Congratulations! You just wrote your first Java app!
Introducing variables in Java
Now it’s time to cover some more important Java basics. Few things are more fundamental to programming than learning how to use variables!
A variable is essentially a “container” for some data. That means you’ll choose a word that is going to represent a value of some sort. We also need to define variables based on the type of data that they are going to reference.
Three basic types of variable that we are going to introduce in this Java tutorial are:
- Integers – Whole numbers.
- Floats – Or “floating point variables.” These contain full numbers that can include decimals. The “floating point” refers to the decimal place.
- Strings – Strings contain alphanumeric characters and symbols. A typical use for a string would be to store someone’s name, or perhaps a sentence.
Once we define a variable, we can then insert it into our code in order to alter the output. For example:
public class HelloWorld
{
public static void main(String[] args)
{
String name = "Adam";
System.out.print("Hello " + name);
}
}
In this example code, we have defined a string variable called “name.” We did this by using the data type “String”, followed by the name of our variable, followed by the data. When you place something in inverted commas in Java, it will be interpreted verbatim as a string.
Now we print to the screen as before, but this time have replaced “Hello world!” With “Hello ” + name. This shows the string “Hello “, followed by whatever value is contained within the following String variable!
The great thing about using variables is that they let us manipulate data so that our code can behave dynamically. By changing the value of name you can change the way the program behaves without altering any actual code!
Conditional statements in Java tutorial
Another of the most important Java basics, is getting to grips with conditional statements.
Conditional statements use code blocks that only run under certain conditions. For example, we might want to grant special user privileges to the main user of our app. That’s me by the way.
So to do this, we could use the following code:
public class HelloWorld
{
public static void main(String[] args)
{
String name = "Adam";
System.out.print("Hello " + name +"\r\n");
if (name == "Adam")
{
System.out.print("Special user priveledges granted!");
}
}
}
Run this code and you’ll see that the special permissions are granted. But if you change the value of name to something else, then the code won’t run!
This code uses an “if” statement. This checks to see if the statement contained within the brackets is true. If it is, then the following code block will run. Remember to indent your code and then close the block at the end! If the statement in the brackets is false, then the code will simply skip over that section and continue from the closed brackets onward.
Notice that we use two “=” signs when we check data. You use just one when you assign data.
Methods in Java tutorial
One more easy concept we can introduce in this Java tutorial is how to use methods. This will give you a bit more idea regarding the way that Java code is structured and what can be done with it.
All we’re going to do, is take some of the code we’ve already written and then place it inside another method outside of the main method:
public class HelloWorld
{
public static void main(String[] args)
{
String name = "Adam";
System.out.print("Hello " + name +"\r\n");
if (name == "Adam") {
grantPermission();
}
}
static void grantPermission()
{
System.out.print("Special user priveledges granted!");
}
}
We created the new method on the line that starts “static void.” This states that the method defines a function rather than a property of an object and that it doesn’t return any data. You can worry about that later!
But anything we insert inside the following code block will now run any time that we “call” the method by writing its name in our code: grantPermission(). The program will then execute that code block and return to the point it left from.
Were we to write grantPermission() multiple times, the “Special user privileges granted!” message would be displayed multiple times! This is what makes methods such fundamental Java basics: they allow you to perform repetitive tasks without writing out code over and over!
Passing arguments in Java
What’s even better about methods though, is that they can receive and manipulate variables. We do this by passing variables into our methods as “Strings.” This is what the brackets following the method name are for.
In the following example, I have created a method that receives a string variable, and I have called that nameCheck. I can then refer to nameCheck from within that code block, and its value will be equal to whatever I placed inside the curly brackets when I called the method.
For this Java tutorial, I’ve passed the “name” value to a method and placed the if statement inside there. This way, we could check multiple names in succession, without having to type out the same code over and over!
Hopefully, this gives you an idea of just how powerful methods can be!
public class HelloWorld
{
public static void main(String[] args)
{
String name = "Adam";
System.out.print("Hello " + name +"\r\n");
checkUser(name);
}
static void checkUser(String nameCheck)
{
if (nameCheck == "Adam")
{
System.out.print("Special user priveledges granted!");
}
}
}
That’s all for now!
That brings us to the end of this Java tutorial. Hopefully, you now have a good idea of how to learn Java. You can even write some simple code yourself: using variables and conditional statements, you can actually get Java to do some interesting things already!
The next stage is to understand object-oriented programming and classes. This understanding is what really gives Java and languages like it their power, but it can be a little tricky to wrap your head around at first!
Read also: What is Object Oriented Programming?
Of course, there is much more to learn! Stay tuned for the next Java tutorial, and let us know how you get on in the comments below.
Other frequently asked questions
Q: Are Java and Python similar?
A: While these programming languages have their similarities, Java is quite different from Python. Python is structure agnostic, meaning it can be written in a functional manner or object-oriented manner. Java is statically typed whereas Python is dynamically typed. There are also many syntax differences.
Q: Should I learn Swift or Java?
A: That depends very much on your intended use-case. Swift is for iOS and MacOS development.
Q: Which Java framework should I learn?
A: A Java framework is a body of pre-written code that lets you do certain things with your own code, such as building web apps. The answer once again depends on what your intended goals are. You can find a useful list of Java frameworks here.
Q: Can I learn Java without any programming experience?
A: If you followed this Java tutorial without too much trouble, then the answer is a resounding yes! It may take a bit of head-scratching, but it is well worth the effort.