Affiliate links on Android Authority may earn us a commission. Learn more.
An introduction to Java syntax for Android development
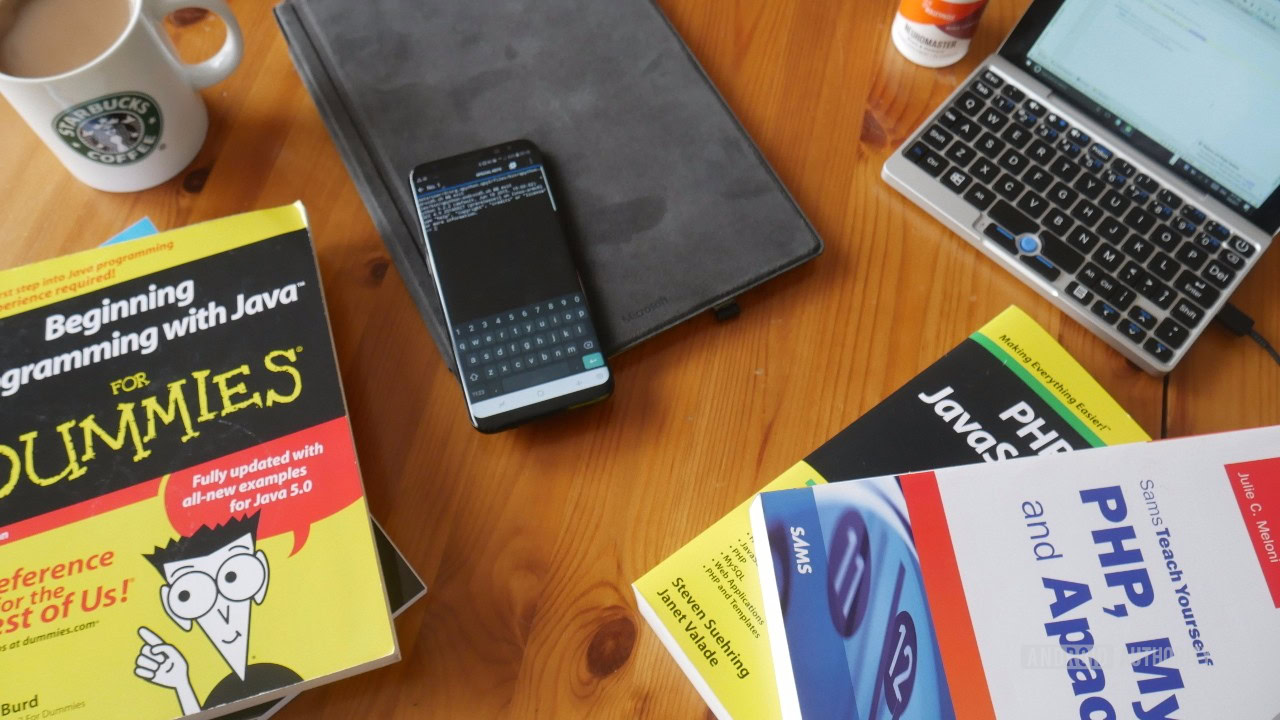
Java is one of the “official” languages that Google supports for Android development — the other being Kotlin. While the latter is increasingly favored by Google however, Java remains popular due to its wide use outside of Android development. This is an in-demand language and learning it can be extremely beneficial for anyone looking to begin a career in development.
Fortunately, there are plenty of tutorials available to walk you through common statements and what they all do. However, before you start decoding Java, it’s important to get a grasp of Java syntax.
Java needs to be written in a certain way — it includes a fair amount of “decoration” and some strange punctuation. This can be a little confusing for newbies and can be a roadblock when trying to follow-along with tutorials. When you figure out these basics though, you’ll be able to read and write new Java commands much more easily. And while it might all seem a little arbitrary, all of these rules and best-practices are here for a reason. Understanding Java syntax can thus help you to prevent issues later down the line!
Let’s look at the grammar of Java and why certain things are laid out the way they are.
Java syntax basics
For now, we’re not necessarily interested in how Java works as such, so much as its basic rules for writing a line of code.
Java code is case sensitive. Later on, you’ll learn how to name your own variables. You need to ensure consistent capitalization use, otherwise you’ll run into an error.
The most important rule of Java syntax: lines end with a semicolon. This punctuation tells Java (and you) that the line of code is over and isn’t intended to run on to the next line. Kind of like a full stop! Some languages (like Kotlin) make this optional, but leaving off the “;” in Java will get a red underline — the code won’t run!
![Java Syntax ]Code Example Java syntax main activity code](https://www.androidauthority.com/wp-content/uploads/2018/07/Java-Syntax-Code-Example.png)
The exception to this rule is when you open up new code blocks. If you end a line with an open curly bracket ({) you are grouping the following lines of code in some way. These will follow on from the preceding line, until the closing }.
Lines ending with curly brackets don’t need to be followed by semi-colons, but the code inside them does and is formatted as normal. You might use this when writing methods — chunks of code you can call upon at any point during your program — and “conditional statements,” which only execute if certain conditions are met.
Code blocks should also be indented. You can have blocks within blocks within blocks (!), and indentations will quickly show us the logical grouping of our code at a glance. Some other programming languages (like Python) require these indents to run code at all, doing away with the curly brackets altogether.
Another exception (sorry) is a comment, which begins with two forward strokes and allows you to write messages for your future self or a colleague, explaining the purpose of the code segment
If some of that went over your head, don’t worry. Just keep it in mind when reading and writing future code. It can look pretty alien at first, but everything is there for a reason!
While it can look pretty alien at first, everything is there for a reason
For now, remember every line must end with a semi-colon, unless it ends with a curly bracket. Code blocks contained within curly brackets are indented, and comments are preceded by two forward slashes.
camelCase
One more thing to keep in mind is the naming convention for your variables and methods. When you create a value to represent something like “Player Health,” you need to give it a name. Variable names can’t include spaces, which means you risk having run-on phrases like “playerhealth” or longer. If you had a whole page of text like that, it would get pretty hard to decipher pretty quickly! Legible code is always the best code.
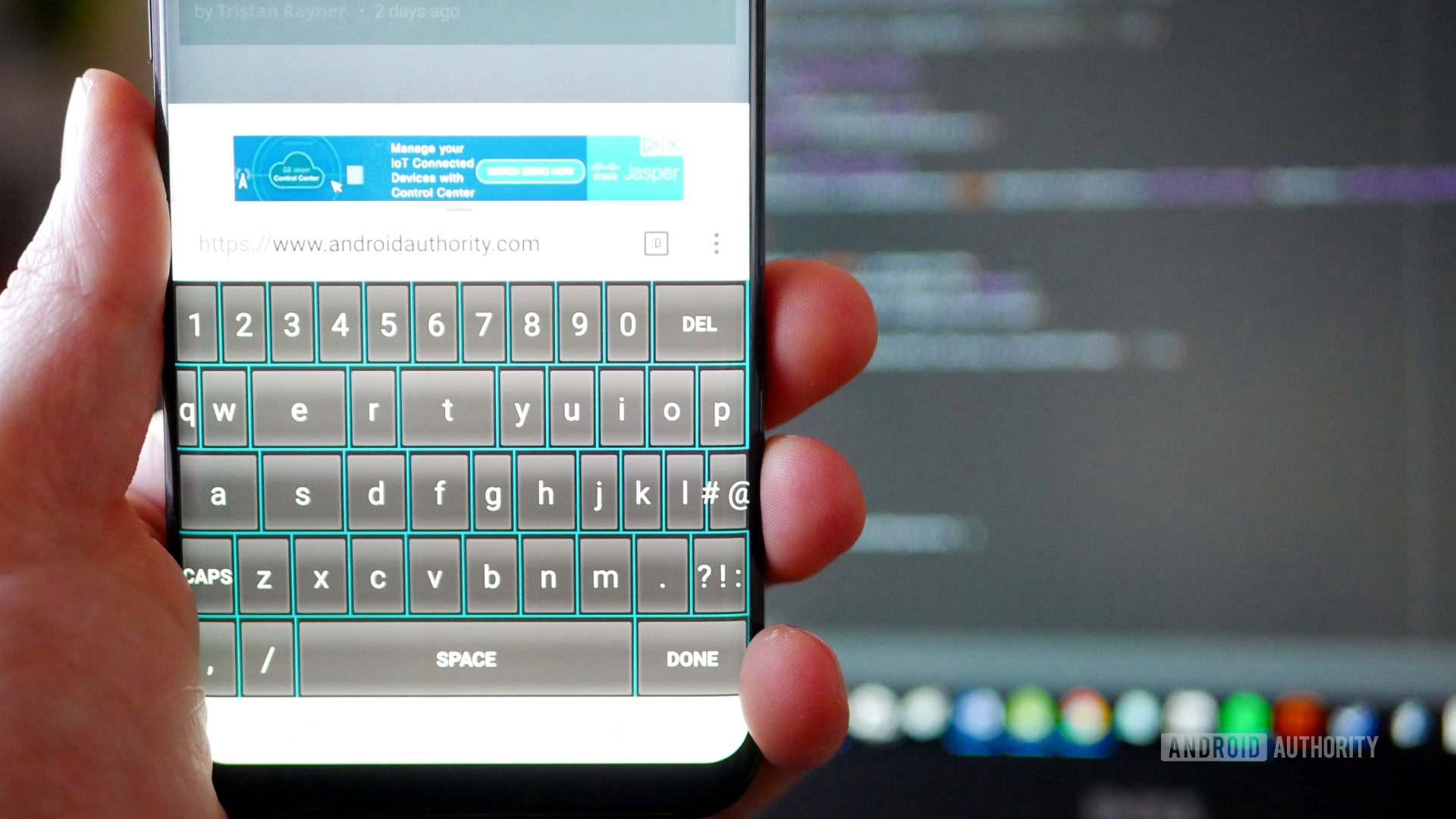
Instead, we use a convention known as “camel case” where each new word begins with a capital letter in order to make it easier to understand. So “Player Health” becomes either “playerHealth'” or “PlayerHealth.” The former (playerHealth) is “lower camelCase'” and the latter (PlayerHealth) is “upper camelCase.”
How you go about using this is up to you (in a way, creating methods and variables lets you define your own Java syntax), but there are some best practices worth looking at. It’s good to follow guidelines like those, as it will keep you from getting confused between Java statements and your own variables, classes, and methods (that will all make sense later).
A simple rule of thumb, is to use lower camelCase for variables, and upper camel case for your methods. This ensures you can distinguish between the two, and knowing that this is how most other people code also makes it easier for you to stop variables in sample code.
It’s very important to name things in a logical manner so everything’s function is easy to understand at a glance. Avoid using acronyms or random words — they’ll only make your code harder to understand if you take a break from it. If it’s ever unclear what something does, write a comment to explain it! Don’t trust your memory to be there for you months later.
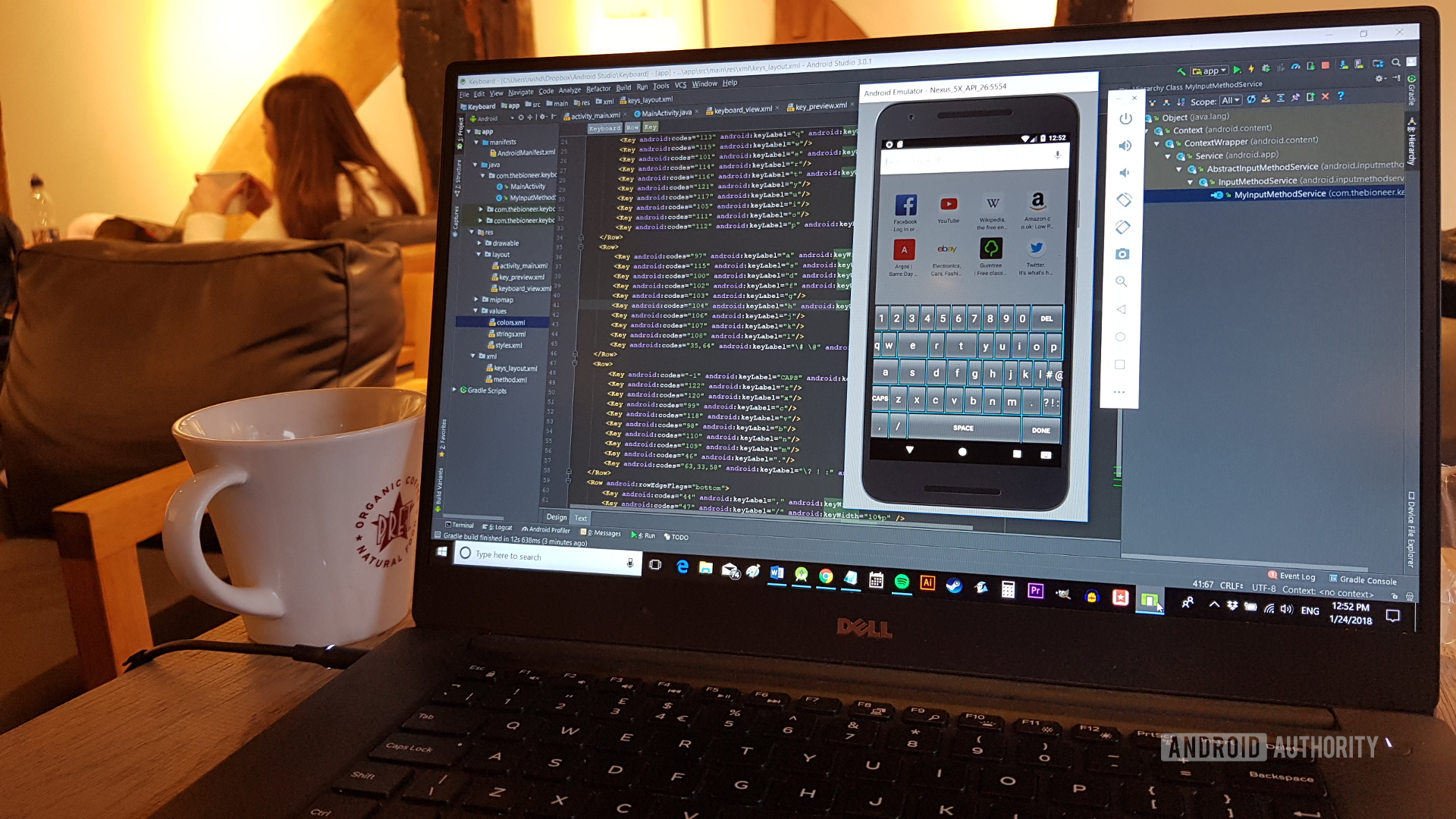
Even the main Java file in our program, MainActivity, is written in camelCase! You can’t have spaces in file names either. Meanwhile, activity_main.xml uses a different naming convention, as files in the resources folder aren’t allowed to have capitalization (I don’t make the rules!). Thus we use an underscore in order to separate out those words.
All this might seem a little arbitrary, but the goal of Java syntax is to make code as readable as possible while avoiding preventable errors. Very smart people tested and experimented for decades before settling on this, so it’s probably pretty good!
Get into good habits now and you’ll save yourself countless hours down the line.