Affiliate links on Android Authority may earn us a commission. Learn more.
Getting started with the Motion Editor in Android Studio 4.0
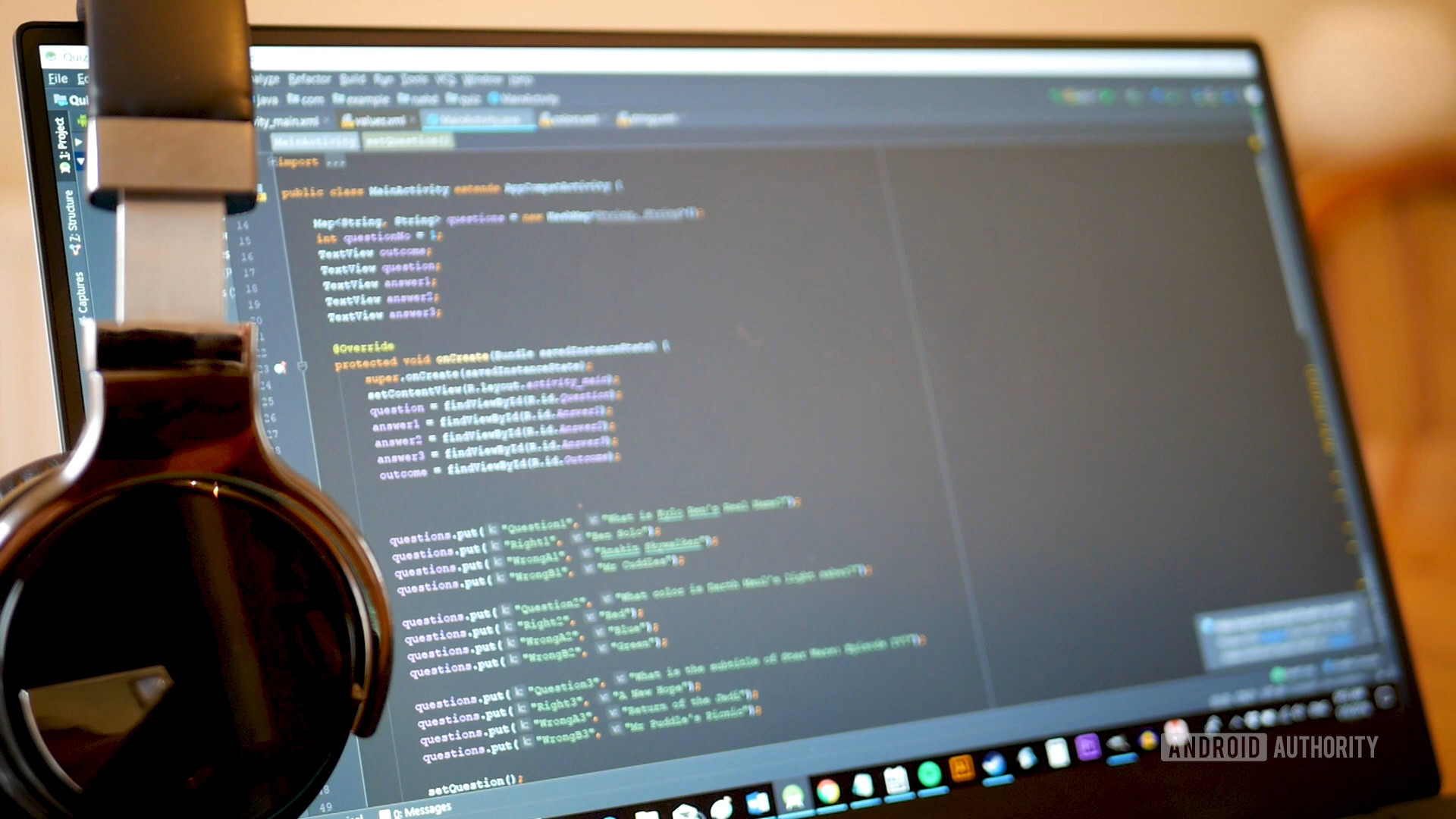
Android Studio 4.0 represents a rather large update for the IDE and offers a lot for devs to get stuck into. Perhaps the most exciting new feature is the “Motion Editor.” This feature is designed to help developers create more attractive, animated layouts. This can significantly improve the UI of any app, and now it’s considerably less fiddly to do!
Also read: An introduction to Jetpack Compose for quick Android UI designs
The basics
Previously, in order to animate a layout, you had to manually modify XML. This new editor makes the process a lot easier by generating that code for you and letting you handle the actual design using a visual editor. In theory at least!
This being Google, the implementation isn’t quite intuitive
Essentially, you will be creating different versions of your layouts by simply dragging and dropping elements that you have defined in a “base” layout. You’ll then create transitions that will move those versions from the first arrangement to the second, and so on.
Also read: All the latest Android developer news and feature you need to know about!
This certainly makes life easier and is a welcome addition. But this being Google, the implementation isn’t quite intuitive out-of-the-box and some key features are currently missing. This guide will hopefully get you started and help you to make sense of the new tool.
Setting up
To get started, you first need to ensure that you have Android Studio 4.0, which is now available on the stable channel. You also need to ensure that you are using the following ConstraintLayout dependency, as MotionLayout is part of the constraint layout beta.
implementation 'com.android.support.constraint:constraint-layout:2.0.0-beta1'
Or:
com.android.support.constraint:constraint-layout:2.0.0-beta1
Next, you’ll need to set up a new Layout Resource File. Make sure that the Root element is set to: androidx.constraintlayout.motion.widget.MotionLayout.
Also read: Android Studio tutorial for beginners
Once that’s built, you’ll be taken straight to the shiny new Motion Editor!
At the moment, you’ll see a message telling you that the Motion Editor cannot be used and that you have a MotionScene Syntax error. Great start!
Creating your first MotionScene
First then, we need to create a motion scene.
The MotionScene object describes how elements are going to be animated in the MotionLayout. To define this object, we need to create another XML file in the XML folder. This will then list layout states that can be used and how to move between them.
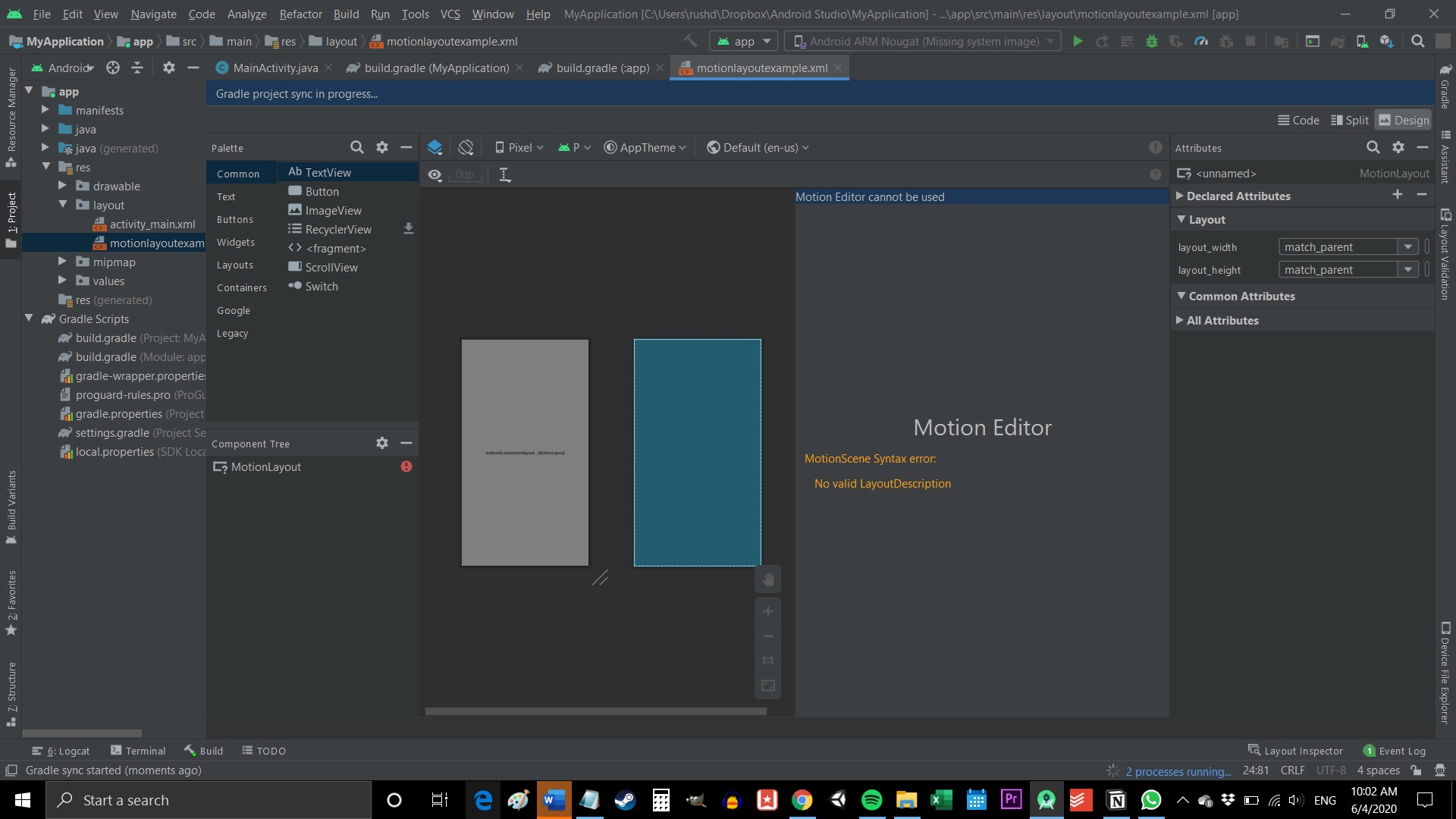
On a side note, some other IDEs would have done this automatically when you first created the new MotionLayout. But I digress!
Fortunately, Android Studio does make this a little easier for us. Just click the red exclamation next to where it says “MotionLayout” in the component tree, and you will be prompted to create a new MotionScene file. Click “Fix” and it will generate that on your behalf and put it in the right place!
The automatically generated file will be given the name of your layout file with “_scene.xml” affixed. My layout file is called “motionlayoutexample” and my scene is called “motionlayoutexample_scene.xml.”
Your scene should contain the following XML:
<MotionScene xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<ConstraintSet android:id="@+id/start">
<Constraint android:id="@+id/widget" />
</ConstraintSet>
<ConstraintSet android:id="@+id/end">
<Constraint android:id="@id/widget" />
</ConstraintSet>
<Transition
app:constraintSetEnd="@id/end"
app:constraintSetStart="@+id/start" />
</MotionScene>
At the moment, the widget that this refers to does not exist, but we’ll remedy that next.
Switch back to the motion layout, and then choose code view. I’m going to drop Google’s own example in here:
<android.support.constraint.motion.MotionLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/motionLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layoutDescription="@xml/motionlayoutexample_scene"
tools:showPaths="true">
<View
android:id="@+id/button"
android:layout_width="64dp"
android:layout_height="64dp"
android:background="@color/colorAccent"
android:text="Button" />
</android.support.constraint.motion.MotionLayout>
Note that I changed the MotionScene file to my own motionlayoutexample_scene. This layout simply shows a button on the screen with the ID “button”.
Annoyingly, I needed to restart Android Studio before it would acknowledge that I had added the layoutDescription correctly. Try that if you have problems!
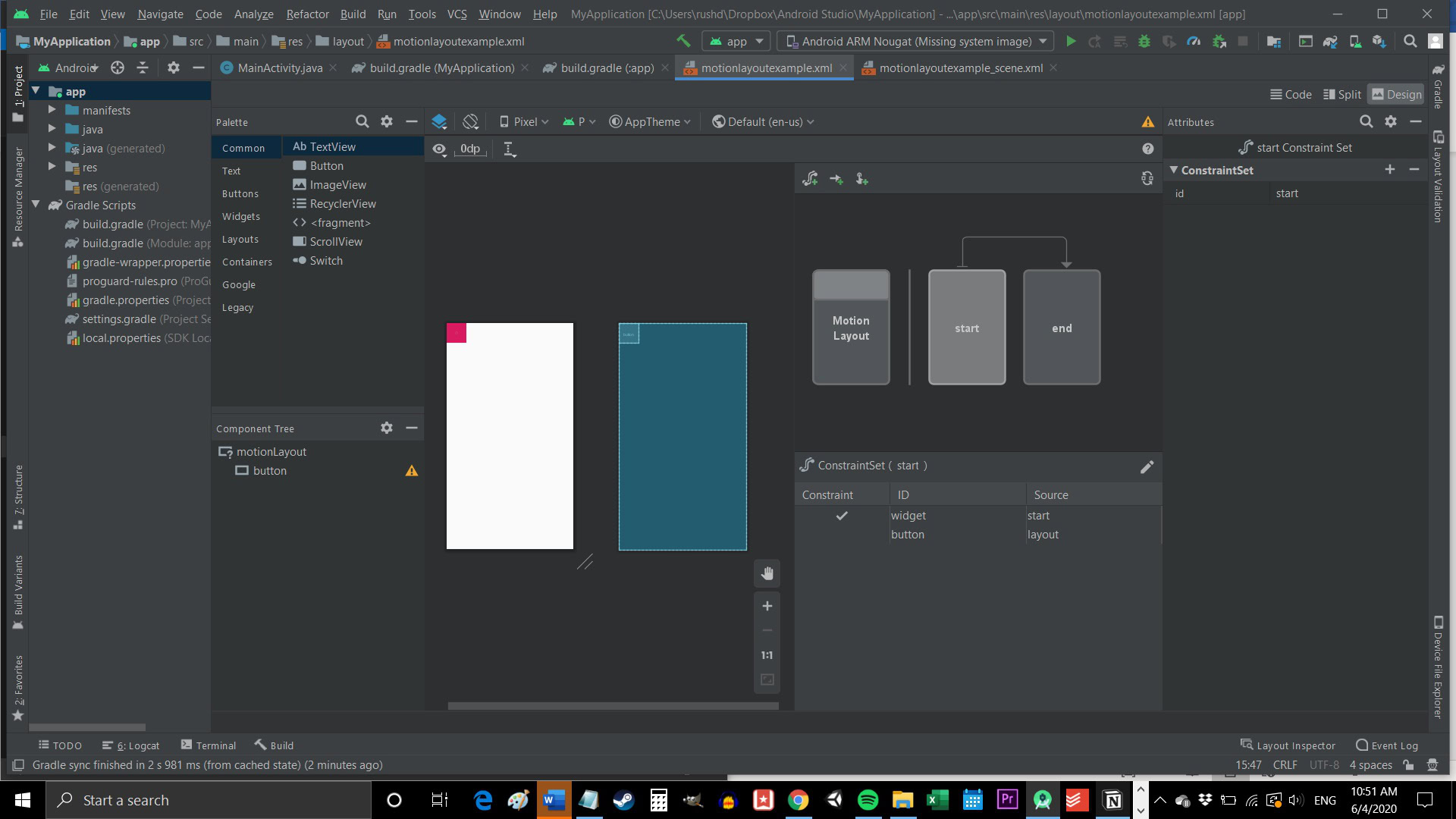
Once that’s done, you should be able to switch to the design view and see a bunch of new controls for you to play around with. You’ll also notice that there is a button in the top left of the screen!
How to animate
The controls on the right allow you to see two states that the layout can adopt: a “start” state and an “end” state. You’ll also see the “base state” which is what you’re looking at now, just the way it is defined in your layout folder.
Android Studio actually refers to these as “ConstraintSets.” The icon in the top left of this window (that looks like two nodes with a small green plus underneath) will allow you to create a new state. The next tool along (the arrow) defines a new transition between those states. The third finger icon allows you to define the actions that trigger the transitions and state changes. This is called a click or swipe handler.
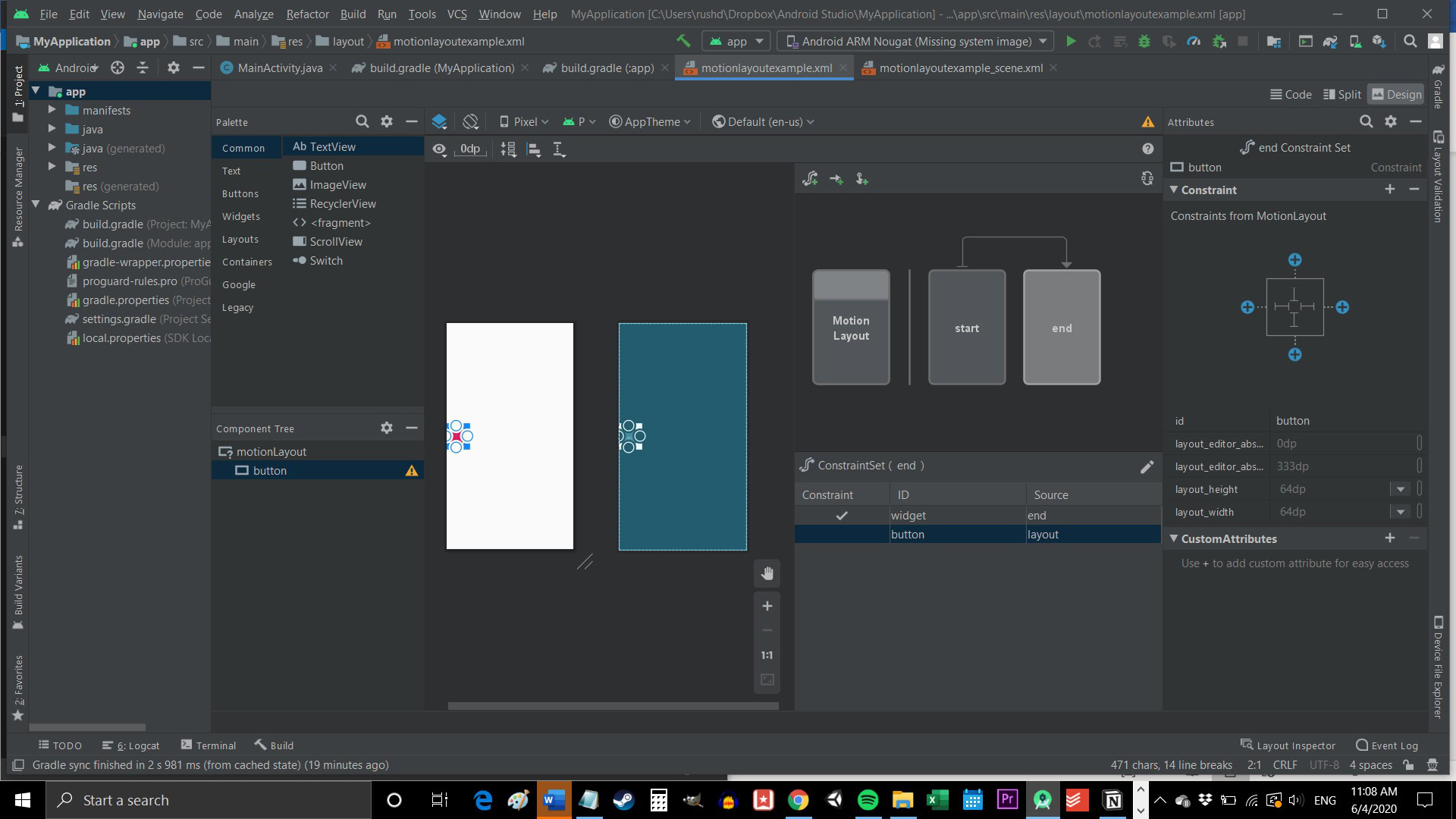
Check the motionlayoutexample_scene XML and you’ll see the “Start” and “End” constraint tags that define these two layouts. You’ll also find the transition tag that tells Android there is some kind of transition between the two.
You can choose any of the states in order to view them in the editor to the left.
Let’s try switching to the “end” state. With that selected, you’re going to edit the constraints to place it at the bottom of the screen.
Switch back and the button should magically reappear at the top! Again, it took a little bit of time before Android Studio would play ball for me. But you can also achieve the same effect by editing the XML in your scene with the starting position set in the first constraint and the ending position in the second.
This is how Google did it:
<ConstraintSet android:id="@+id/start">
<Constraint
android:id="@+id/button"
android:layout_width="64dp"
android:layout_height="64dp"
android:layout_marginStart="8dp"
motion:layout_constraintBottom_toBottomOf="parent"
motion:layout_constraintStart_toStartOf="parent"
motion:layout_constraintTop_toTopOf="parent" />
</ConstraintSet>
<ConstraintSet android:id="@+id/end">
<Constraint
android:id="@+id/button"
android:layout_width="64dp"
android:layout_height="64dp"
android:layout_marginEnd="8dp"
motion:layout_constraintBottom_toBottomOf="parent"
motion:layout_constraintEnd_toEndOf="parent"
motion:layout_constraintTop_toTopOf="parent" />
</ConstraintSet>
To view the animation in action, simply click on the transition itself (the arrow above the two states) then click play. You should now see the button repeatedly slide down the screen! You can also set keyframes this way for more advanced animations.
Finally, decide what you want to trigger this animation by using the click or swipe handler. Simply choose the transition to deploy from the first drop-down box, and then the view that you want to register the action.
Where to go from here
While the tool is a little fiddly and buggy right now, it definitely has a lot of potential. And there’s more you can do with it too!
Of course, you can add new views just as you normally would via the editor (make sure the default Motion Layout is selected). You can also add new states and transitions between them. If you want to add custom elements to your animations (like color changes), you can do so by using Custom Attributes. Hopefully, this will be built into the editor proper in future.
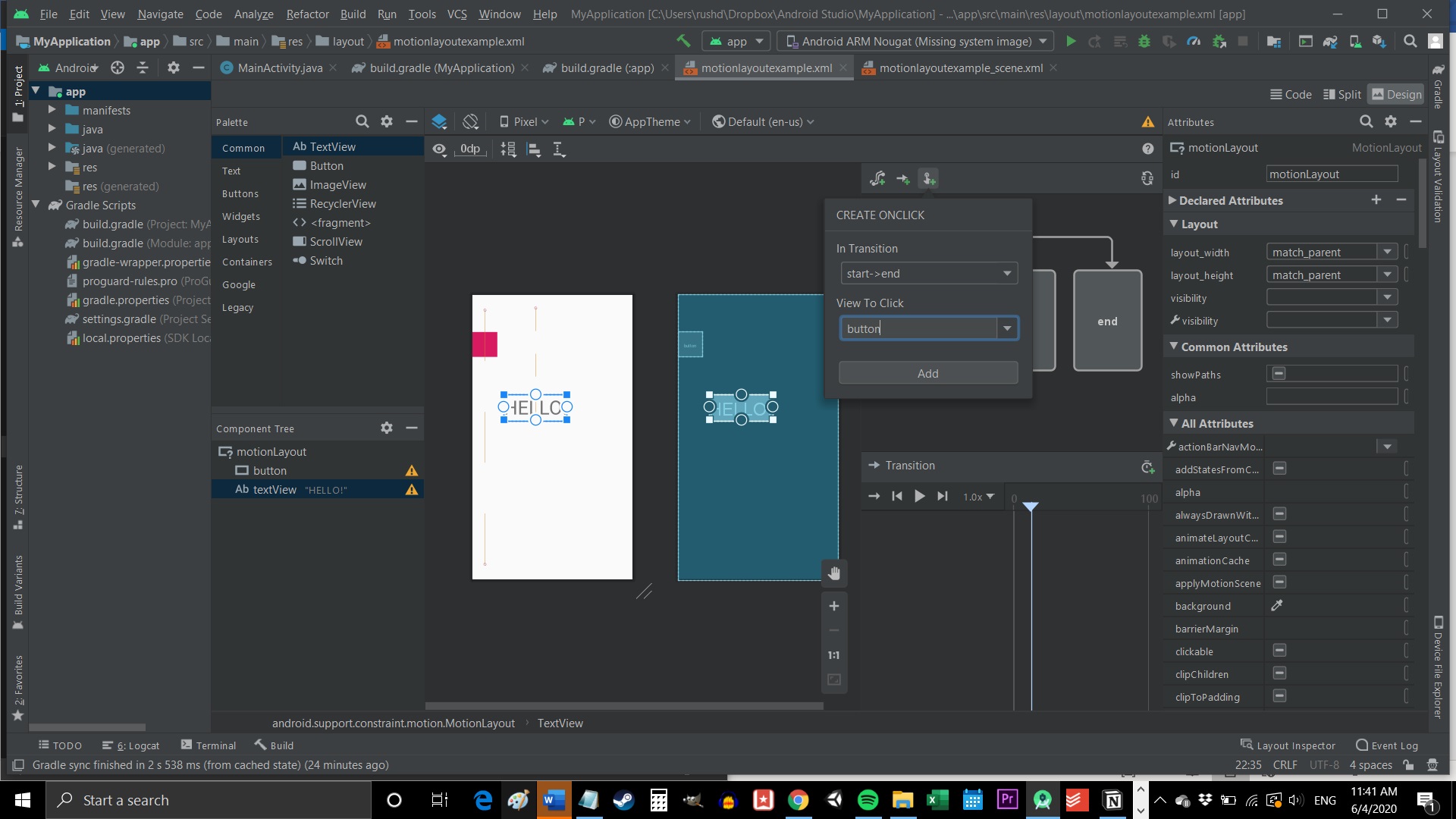
Check out the official documentation from Google for more details. Hopefully, this introduction has filled you in on the basics and you now have a good idea of what can be done with the new Motion Editor and how to get started. Let us know how you get on in the comments below!
Happy animating!