Affiliate links on Android Authority may earn us a commission. Learn more.
Let's build a custom keyboard for Android
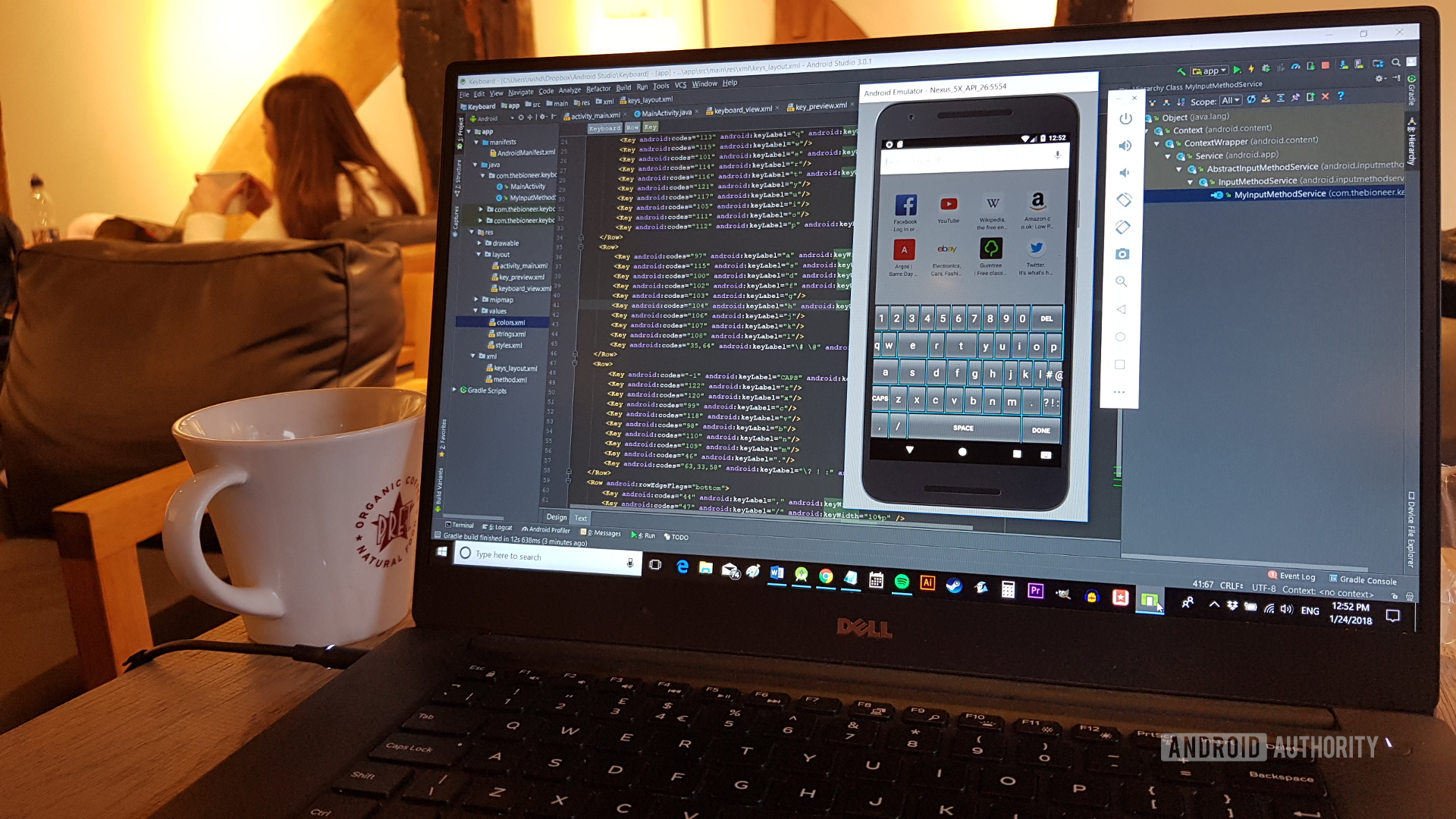
When thinking of building an Android app, we often think of something with a screen and a contained function. It could be a game, or a tool to perform a common task.
But apps can come in a variety of shapes and sizes. You could build a service that runs in the background and quietly makes life easier for the user. You could create a widget, or a launcher. How about a keyboard?
A keyboard can make every interaction quicker, easier and less prone to errors.
Upgrading the software keyboard on your device is one of the most profound ways to customize a device. Most of us use the keyboard as our primary input method. It’s integral to nearly every interaction with your phone. In the best-case scenario, it can make everything quicker, easier, and less error-prone.
Keyboard apps can be highly successful for this reason too; just look at the ubiquity of Swype and SwiftKey.
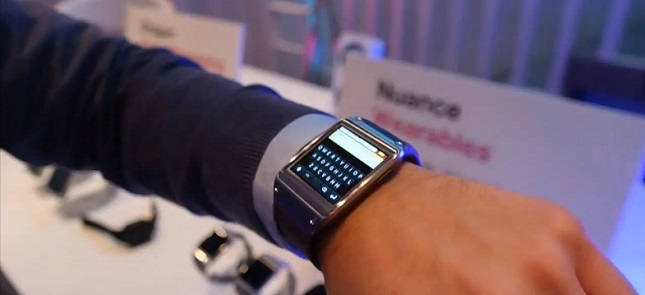
Whether you just want to take your Android customization to the next level, or you’d like to sell a whole new way to interact with a smart device, read on and let’s explore how to create an Android keyboard.
Note: This project is relatively simple and requires mostly copying and pating XML script. However, it does include some more advanced concepts like services and inheritance. If you’re happy to follow along to get a keyboard running, then anyone should be able to reproduce the app. If you want to understand what everything does, this is a good intermediate project to wrap your head around. You will of course need Android Studio and the Android SDK already set-up.
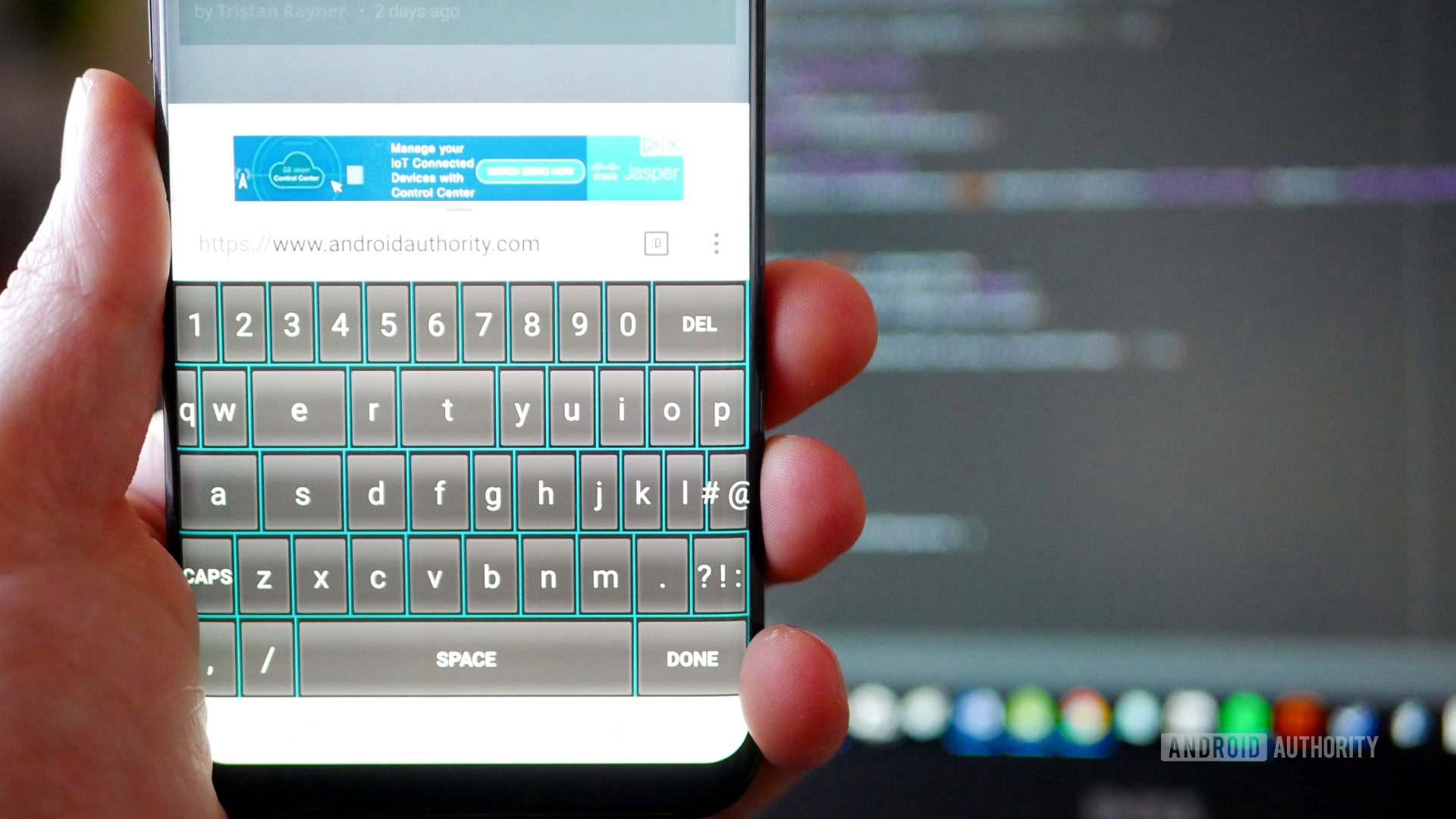
Layout files. LOTS of layout files
To build our custom keyboard, we’re first going to need to create a new xml file, which will define the layout and appearance of our keyboard. That file will be called keyboard_view.xml. To create this, right click on the “layout” folder in your “res” directory and choose “layout resource file.” In the dialog box that pops up, clear the text where it says “Root element” and start typing “keyboard.” Select the first option which comes up, which should be: android.inputmethodservice.KeyboardView. Call the file keyboard_view.xml (remember, no capitals for resources!).
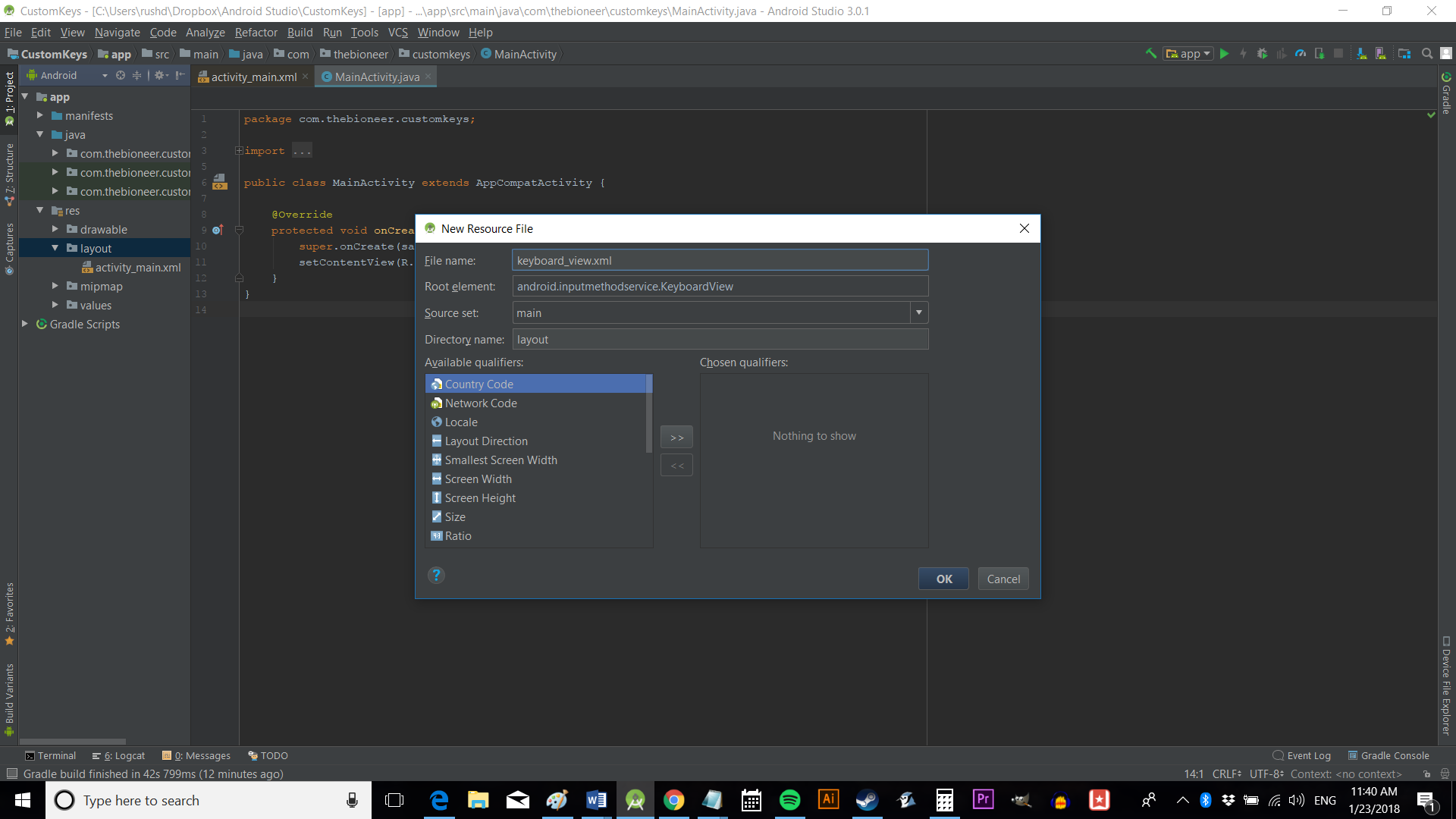
You will be greeted by a file looking like this:
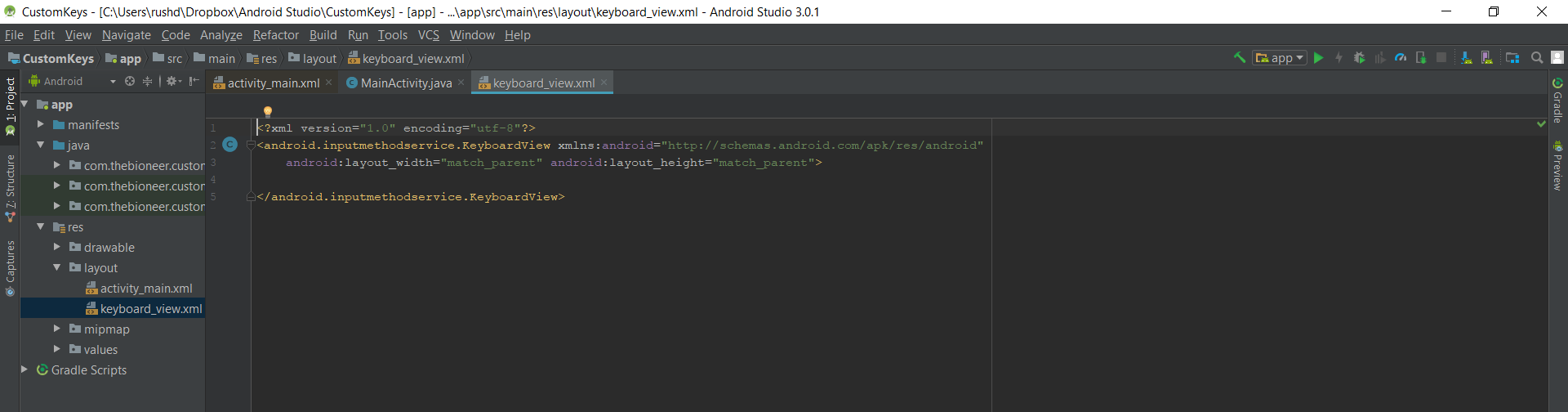
We’re going to add a few elements now:
android:id="@+id/keyboard_view"
android:keyPreviewLayout="@Layout/key_preview"
android:layout_alignParentBottom="true"
android:background="@color/colorPrimary">
We’ve assigned an ID here so we can refer to the keyboard later in our code. The code aligns our keyboard to the bottom of the screen and the background color is set to colorPrimary. This color is the one set in our values > colors.xml file — it’s easy to change later on. So just hop in there and change the respective color code to change the look a little.
We’ve also referred to another layout for “keyboard preview.” In case you’re scratching your head, that’s the image of the key that flashes up in a large font when you make contact. This assures the user they hit the right key.
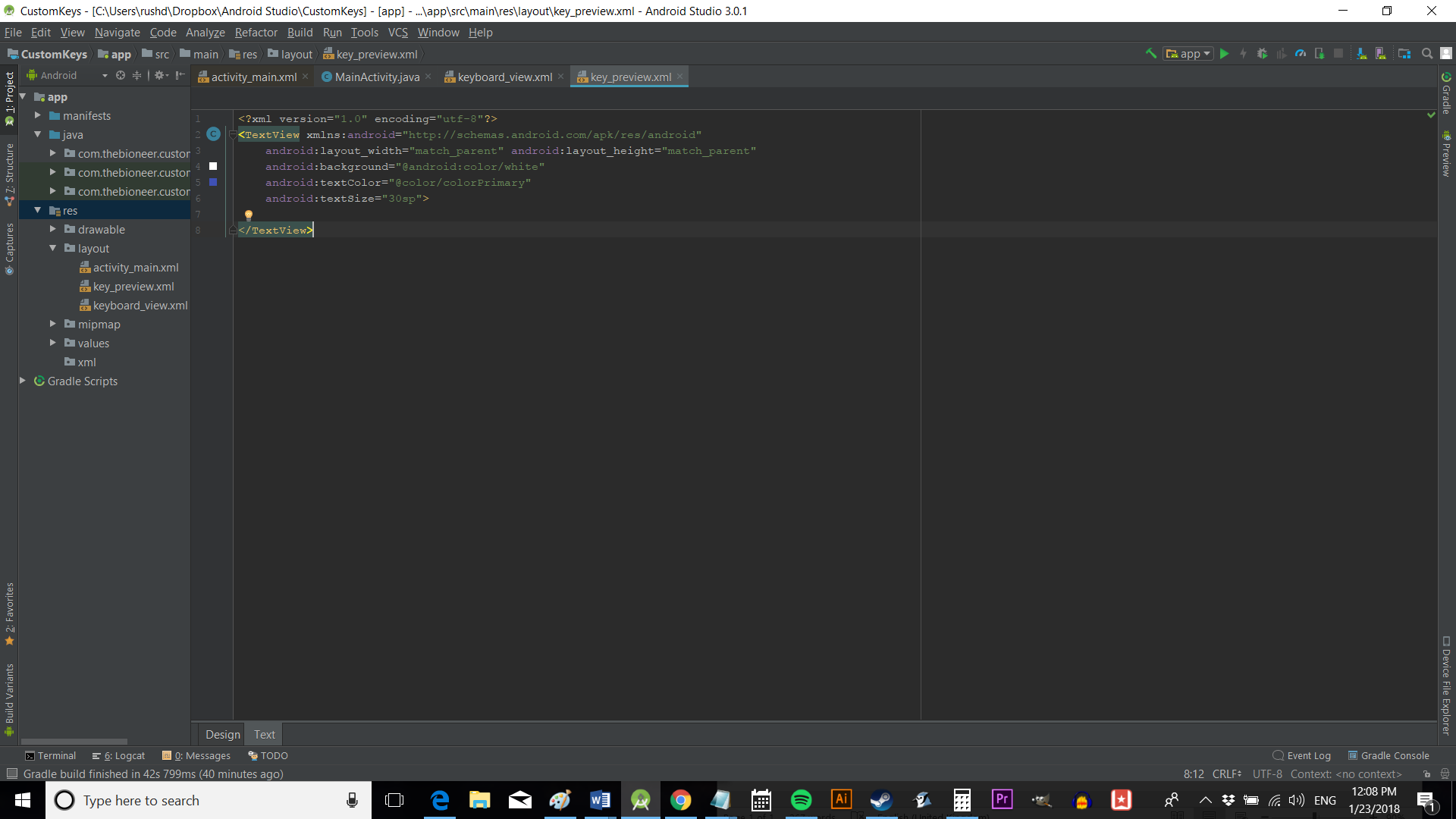
As you’ve possibly guessed, this means we need another new layout file, the aforementioned keyboard_preview.xml. Create it in just the same way, though the root element this time is TextView.
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent"
android:background="@color/colorPrimaryDark"
android:textColor="@color/colorPrimary"
android:textAlignment="center"
android:textSize="30sp">
</TextView>
Add this code and you’ll define the color of the square and the color of the letter appearing in the square. I also set the alignment to center, which ensures it looks the way it should.
The next new XML file is called method.xml. This will go in your resources folder and have the root element input-method. This file will tell Android what type of input is available through your app. Again, you want to replace the boilerplate code that’s there so that it reads like this:
<input-method xmlns:android="http://schemas.android.com/apk/res/android">
<subtype android:imeSubtypeMode="keyboard" />
</input-method>
You can also put information such as language in here later on.
This is where we will create the layout for our keyboard — it’s nearly the fun part!
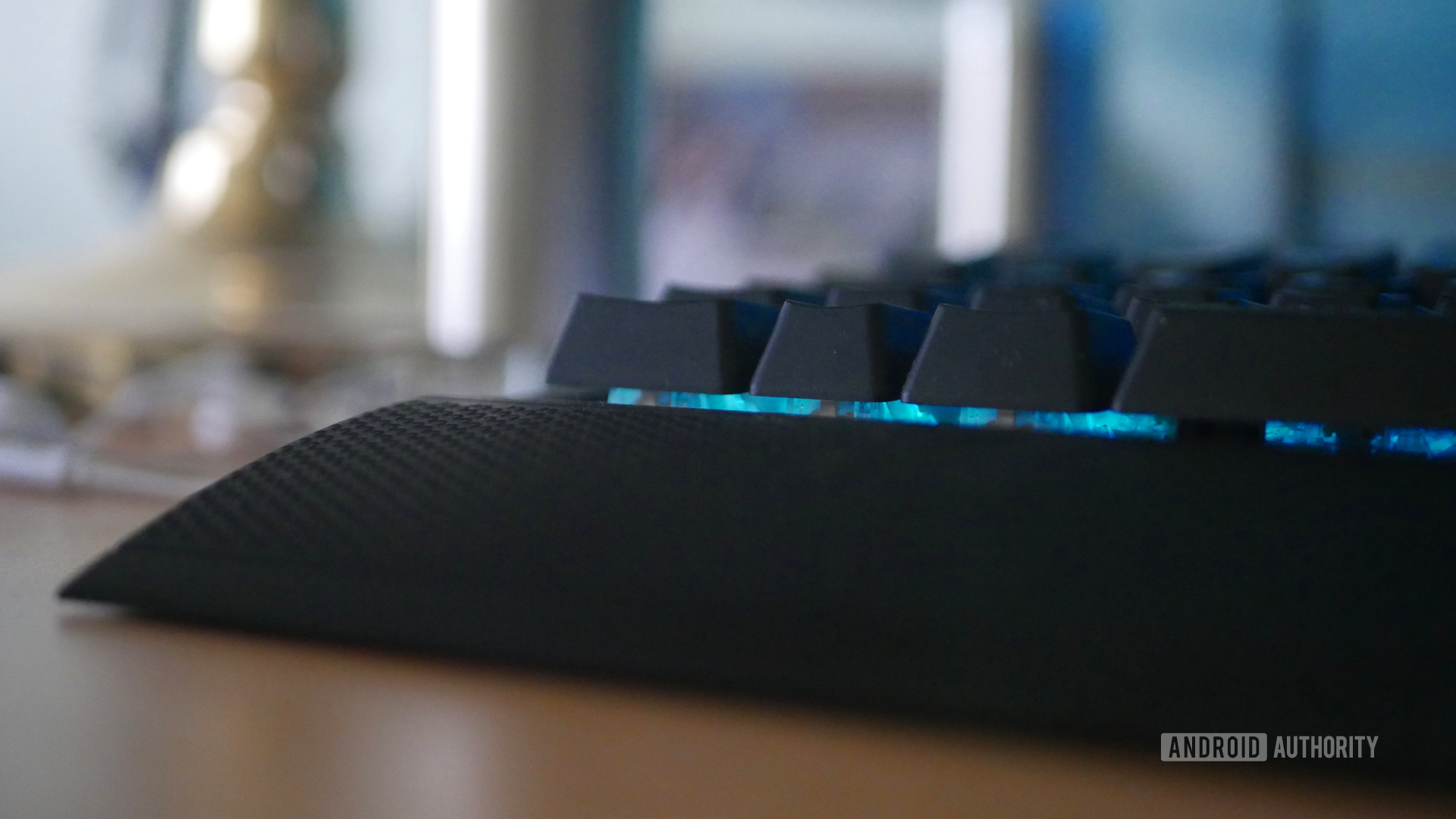
That will go in a new directory you’ll create (res — xml) and I’m calling mine keys_layout.xml. Replace the code that’s there with this:
<?xml version="1.0" encoding="utf-8"?>
<Keyboard xmlns:android="http://schemas.android.com/apk/res/android">
</Keyboard>
This is what we’ll be populating with the keys and their behaviors.
Designing your keyboard
We’ve built a bunch of XML files and now we’re ready to start having some fun. It’s time to create the layout of the keys!
This is what I used. It’s basically a slightly tweaked version of a keyboard layout I found online, with the keys all in standard rows. It’s not exactly beautiful, but it’ll do.
<?xml version="1.0" encoding="utf-8"?>
<Keyboard xmlns:android="http://schemas.android.com/apk/res/android"
android:keyWidth="10%p"
android:horizontalGap="3px"
android:verticalGap="3px"
android:keyHeight="60dp"
android:keyTextColor="@color/colorAccent"
android:keyBackground="@color/colorPrimaryDark"
>
<Row>
<Key android:codes="49" android:keyLabel="1" android:keyEdgeFlags="left"/>
<Key android:codes="50" android:keyLabel="2"/>
<Key android:codes="51" android:keyLabel="3"/>
<Key android:codes="52" android:keyLabel="4"/>
<Key android:codes="53" android:keyLabel="5"/>
<Key android:codes="54" android:keyLabel="6"/>
<Key android:codes="55" android:keyLabel="7"/>
<Key android:codes="56" android:keyLabel="8"/>
<Key android:codes="57" android:keyLabel="9"/>
<Key android:codes="48" android:keyLabel="0"/>
<Key android:codes="-5" android:keyLabel="DEL" android:keyWidth="20%p" android:isRepeatable="true" android:keyEdgeFlags="right"/>
</Row>
<Row>
<Key android:codes="113" android:keyLabel="q" android:keyEdgeFlags="left"/>
<Key android:codes="119" android:keyLabel="w"/>
<Key android:codes="101" android:keyLabel="e"/>
<Key android:codes="114" android:keyLabel="r"/>
<Key android:codes="116" android:keyLabel="t"/>
<Key android:codes="121" android:keyLabel="y"/>
<Key android:codes="117" android:keyLabel="u"/>
<Key android:codes="105" android:keyLabel="i"/>
<Key android:codes="111" android:keyLabel="o"/>
<Key android:codes="112" android:keyLabel="p" android:keyEdgeFlags="right"/>
</Row>
<Row>
<Key android:codes="97" android:keyLabel="a" android:keyEdgeFlags="left"/>
<Key android:codes="115" android:keyLabel="s"/>
<Key android:codes="100" android:keyLabel="d"/>
<Key android:codes="102" android:keyLabel="f"/>
<Key android:codes="103" android:keyLabel="g"/>
<Key android:codes="104" android:keyLabel="h"/>
<Key android:codes="106" android:keyLabel="j"/>
<Key android:codes="107" android:keyLabel="k"/>
<Key android:codes="108" android:keyLabel="l"/>
<Key android:codes="35,64" android:keyLabel="\# \@" android:keyEdgeFlags="right"/>
</Row>
<Row>
<Key android:codes="-1" android:keyLabel="CAPS" android:keyEdgeFlags="left"/>
<Key android:codes="122" android:keyLabel="z"/>
<Key android:codes="120" android:keyLabel="x"/>
<Key android:codes="99" android:keyLabel="c"/>
<Key android:codes="118" android:keyLabel="v"/>
<Key android:codes="98" android:keyLabel="b"/>
<Key android:codes="110" android:keyLabel="n"/>
<Key android:codes="109" android:keyLabel="m"/>
<Key android:codes="46" android:keyLabel="."/>
<Key android:codes="63,33,58" android:keyLabel="\? ! :" android:keyEdgeFlags="right"/>
</Row>
<Row android:rowEdgeFlags="bottom">
<Key android:codes="44" android:keyLabel="," android:keyWidth="10%p" android:keyEdgeFlags="left"/>
<Key android:codes="47" android:keyLabel="/" android:keyWidth="10%p" />
<Key android:codes="32" android:keyLabel="SPACE" android:keyWidth="60%p" android:isRepeatable="true"/>
<Key android:codes="-4" android:keyBackground="@color/colorPrimary" android:keyLabel="DONE" android:keyWidth="20%p" android:keyEdgeFlags="right"/>
</Row>
</Keyboard>
You’ll notice a few interesting things here. The android:codes tell us what each key needs to do. This is what we’ll be receiving through our service shortly and you need to make sure the keyLabel (the text on the keys) lines up with what it actually does. Well, unless your objective is to create a “troll keyboard.”
If you place more than one code separated by commas, your keys will scroll through those options if the user double or triple taps. That way we can make a keyboard that works like the old T9 numpad keyboards on Nokia phones, for instance.
Negative codes represent the constants in the keyboard class. -5 is the equivalent of KEYCODE_DELETE. Play around, use your imagination, and see if you can come up with a “better keyboard.”
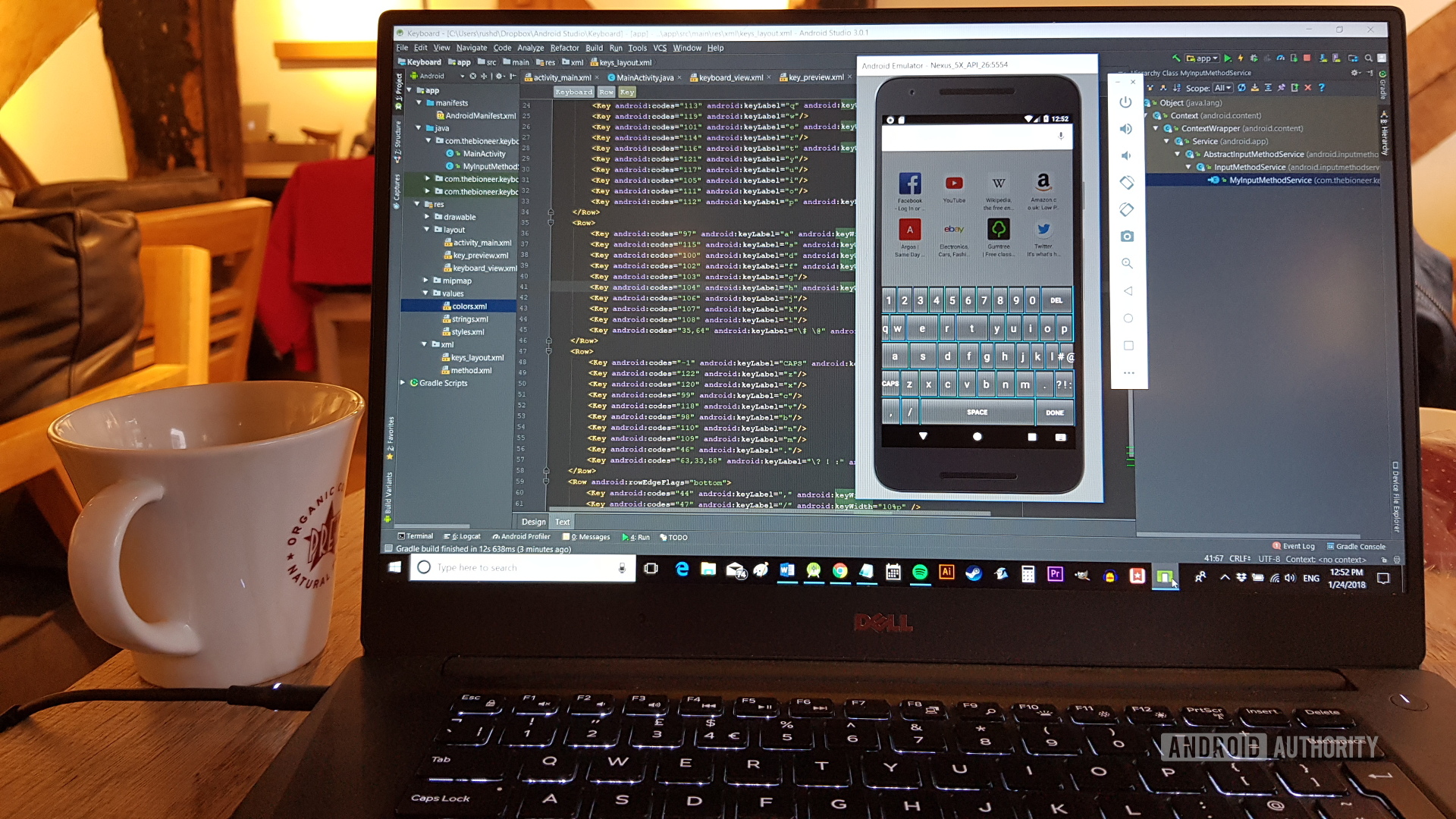
An obvious choice is to make the more popular keys a little larger. That’s what I’ve started doing.
At your service
Now it’s time to create a java class. This is going to be called MyInputMethodService and, as the name suggests, it’s going to be a service. The superclass will be android.inputmethodservice, meaning it will inherit properties from that kind of class and behave like an input method service should (politely).
Under Interface(s), we’ll be implementing OnKeyboardActionListener. Start typing and then select the suggestion that springs up.
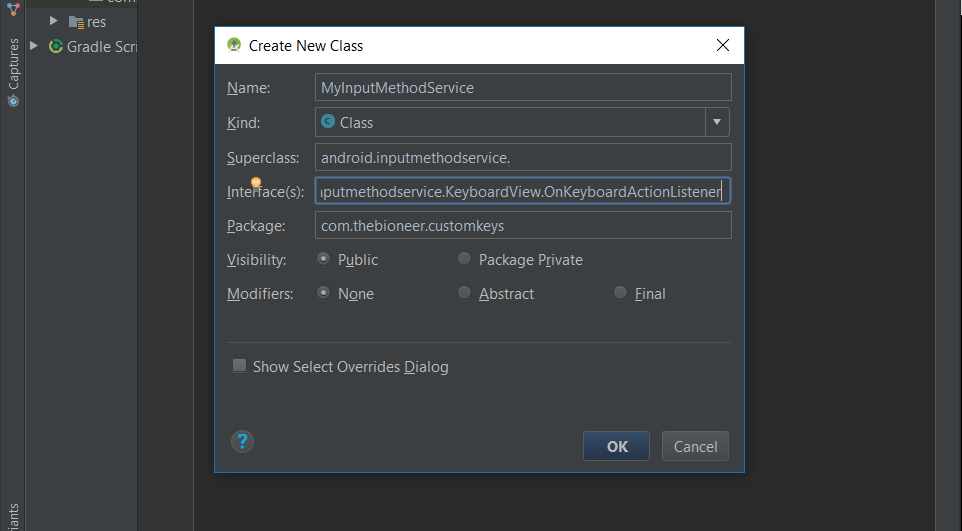
Being a service, this means your app can run in the background and then listen out for the moment when it is needed – when the user selects an edit text in another application for example.
Your class will be underlined red when this is generated, which is because it needs to implement the methods from InputMethodService. You can generate this automatically by right clicking on your class and choosing generate — implement methods.
Here’s what it should look like:
public class MyInputMethodService extends InputMethodService implements KeyboardView.OnKeyboardActionListener {
public MyInputMethodService() {
super();
}
@Override
public void onPress(int i) {
}
@Override
public void onRelease(int i) {
}
@Override
public void onKey(int i, int[] ints) {
}
@Override
public void onText(CharSequence charSequence) {
}
@Override
public void swipeLeft() {
}
@Override
public void swipeRight() {
}
@Override
public void swipeDown() {
}
@Override
public void swipeUp() {
}
}
You also need to override the onCreateInputView() method, which is going to grab the keyboard view and add the layout onto it.
Now add the following code, remembering to import all classes as necessary.
private KeyboardView keyboardView;
private Keyboard keyboard;
private boolean caps = false;
@Override
public View onCreateInputView() {
keyboardView = (KeyboardView) getLayoutInflater().inflate(R.layout.keyboard_view, null);
keyboard = new Keyboard(this, R.xml.keys_layout);
keyboardView.setKeyboard(keyboard);
keyboardView.setOnKeyboardActionListener(this);
return keyboardView;
}
When the input view is created, it takes the layout file keyboard_view and uses it to define how it looks. It also adds the keys_layout file we created and returns the view for the system to use.
I’ve also added a Boolean (true or false variable) called caps so we can keep track of caps-lock.
The other important method here, is the one handling key presses. Try this:
@Override
public void onKey(int primaryCode, int[] keyCodes) {
InputConnection inputConnection = getCurrentInputConnection();
if (inputConnection != null) {
switch(primaryCode) {
case Keyboard.KEYCODE_DELETE :
CharSequence selectedText = inputConnection.getSelectedText(0);
if (TextUtils.isEmpty(selectedText)) {
inputConnection.deleteSurroundingText(1, 0);
} else {
inputConnection.commitText("", 1);
}
case Keyboard.KEYCODE_SHIFT:
caps = !caps;
keyboard.setShifted(caps);
keyboardView.invalidateAllKeys();
break;
case Keyboard.KEYCODE_DONE:
inputConnection.sendKeyEvent(new KeyEvent(KeyEvent.ACTION_DOWN, KeyEvent.KEYCODE_ENTER));
break;
default :
char code = (char) primaryCode;
if(Character.isLetter(code) && caps){
code = Character.toUpperCase(code);
}
inputConnection.commitText(String.valueOf(code), 1);
}
}
}
This is a switch statement which looks for the key code and acts accordingly. When the user clicks specific keys, the code will change course. KEYCODE_SHIFT changes our caps Boolean, sets the keyboard to “Shifted,” and then invalidates the keys (to redraw them).
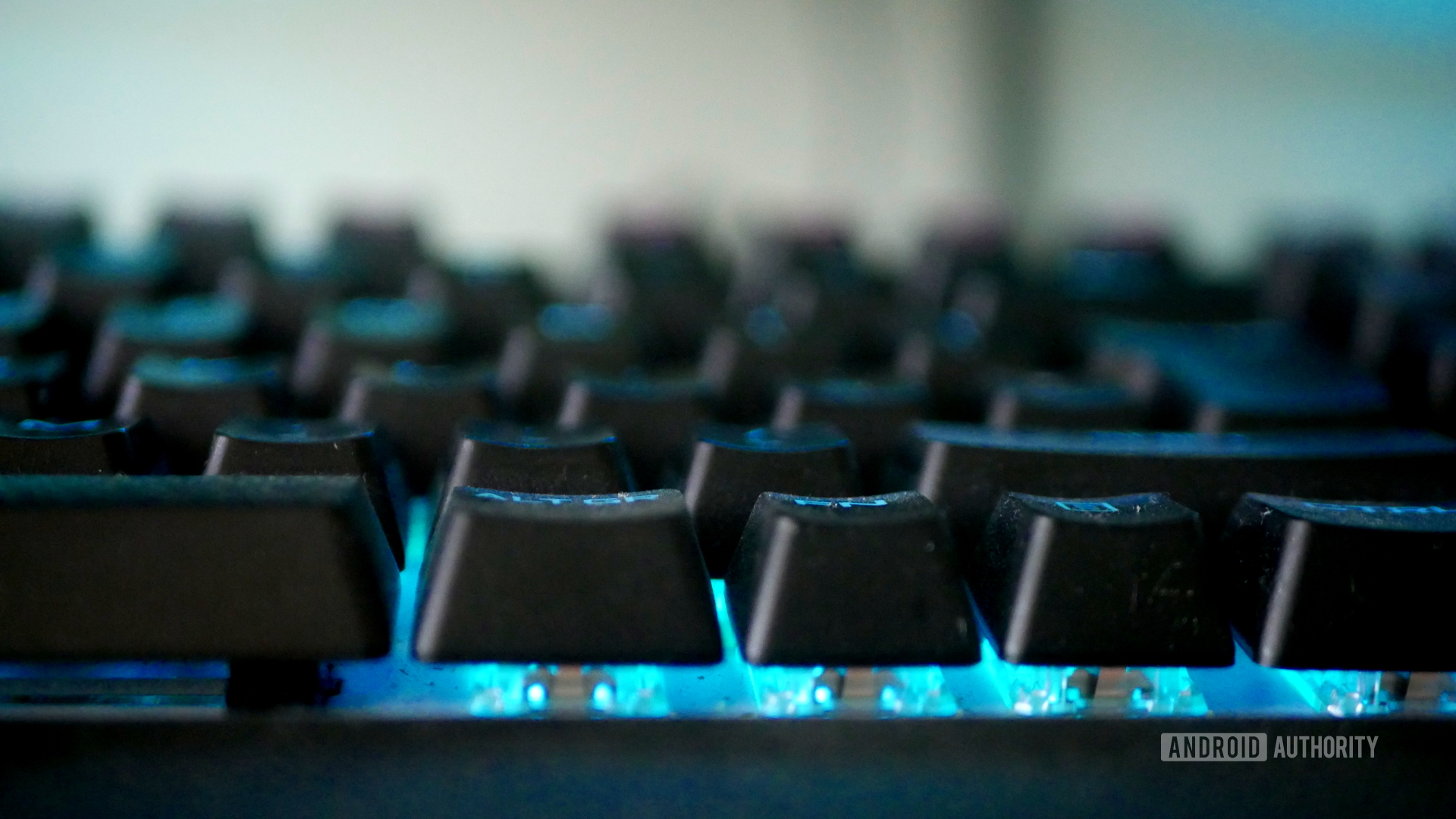
commitText simply sends text (which can include multiple characters) to the input field. sendKeyEvent will send events like “return” to the app.
The class in its entirety should look like this:
public class MyInputMethodService extends InputMethodService implements KeyboardView.OnKeyboardActionListener {
private KeyboardView keyboardView;
private Keyboard keyboard;
private boolean caps = false;
@Override
public View onCreateInputView() {
keyboardView = (KeyboardView) getLayoutInflater().inflate(R.layout.keyboard_view, null);
keyboard = new Keyboard(this, R.xml.keys_layout);
keyboardView.setKeyboard(keyboard);
keyboardView.setOnKeyboardActionListener(this);
return keyboardView;
}
@Override
public void onPress(int i) {
}
@Override
public void onRelease(int i) {
}
@Override
public void onKey(int primaryCode, int[] keyCodes) {
InputConnection inputConnection = getCurrentInputConnection();
if (inputConnection != null) {
switch(primaryCode) {
case Keyboard.KEYCODE_DELETE :
CharSequence selectedText = inputConnection.getSelectedText(0);
if (TextUtils.isEmpty(selectedText)) {
inputConnection.deleteSurroundingText(1, 0);
} else {
inputConnection.commitText("", 1);
}
case Keyboard.KEYCODE_SHIFT:
caps = !caps;
keyboard.setShifted(caps);
keyboardView.invalidateAllKeys();
break;
case Keyboard.KEYCODE_DONE:
inputConnection.sendKeyEvent(new KeyEvent(KeyEvent.ACTION_DOWN, KeyEvent.KEYCODE_ENTER));
break;
default :
char code = (char) primaryCode;
if(Character.isLetter(code) && caps){
code = Character.toUpperCase(code);
}
inputConnection.commitText(String.valueOf(code), 1);
}
}
}
@Override
public void onText(CharSequence charSequence) {
}
@Override
public void swipeLeft() {
}
@Override
public void swipeRight() {
}
@Override
public void swipeDown() {
}
@Override
public void swipeUp() {
}
}
Testing it out and customization
In order to test your new keyboard, you’ll need to add it via your device’s settings. To do this, go to Language & Input — Virtual Keyboard — Manage Keyboards and turn on the keyboard you created. Select “OK” a few times to dismiss the notifications.
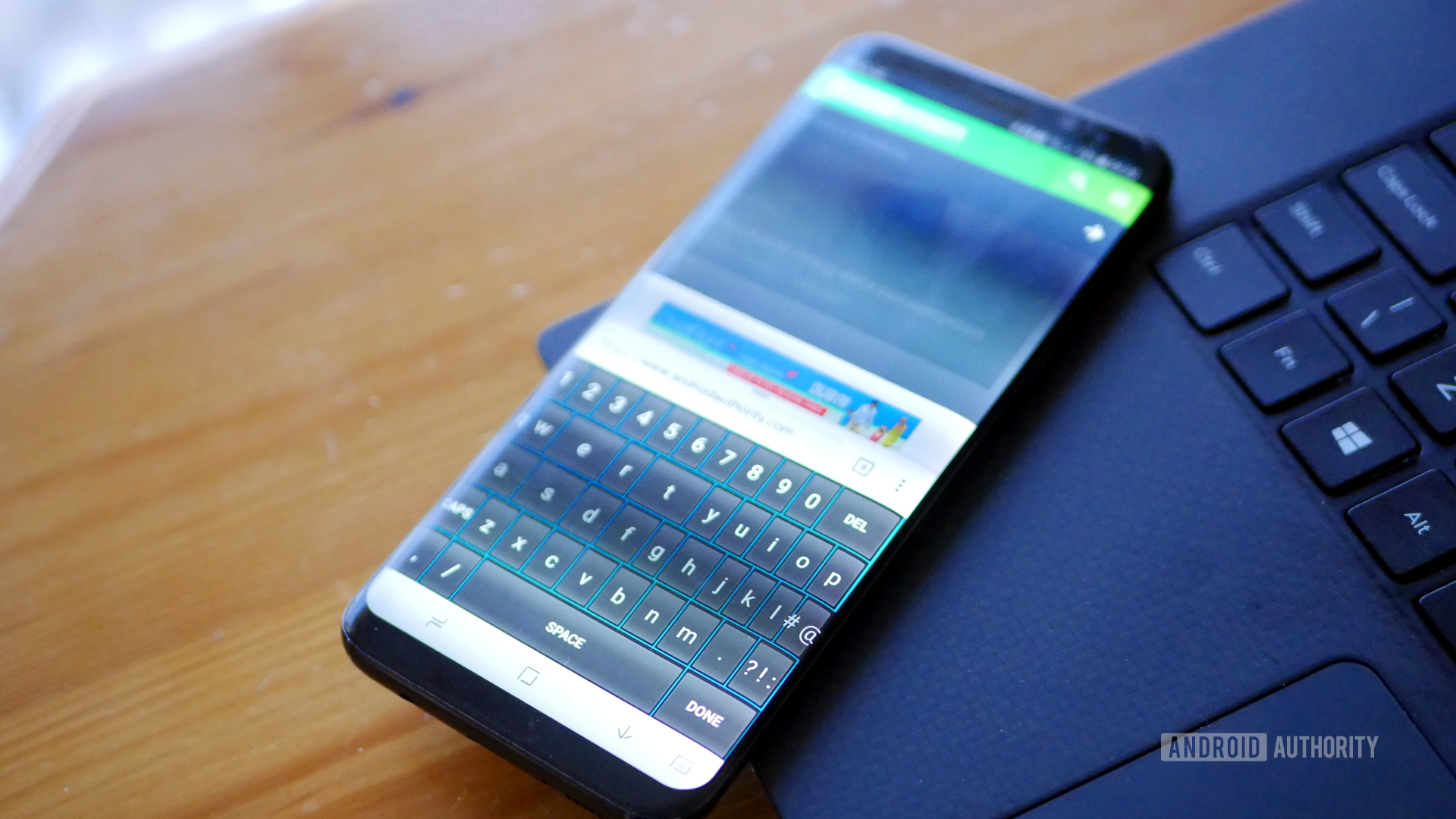
Now open up any app with a text input and bring up your keyboard. You’ll notice a little keyboard icon in the bottom right. Select that and then pick your app from the list. If all has gone to plan, your keyboard should now spring to life!
Play around with different key sizes, customization and features to create the perfect typing experience.
This is a little confusing for new users, so if you plan on selling this app, it might be a good idea to add some text to the MainActivity.Java file, explaining how to select the keyboard. You could also use this in order to add some customization or settings for the users to tweak.
You could add plenty of customization options. How about letting the user change the height and size of their keyboard? You could let them change the colors, use different icons for the keys (android:keyicon), or change the images entirely (android:keybackground=@drawable/). For more advanced options — like changing the color of each individual key — you’ll need to use Java, not XML.
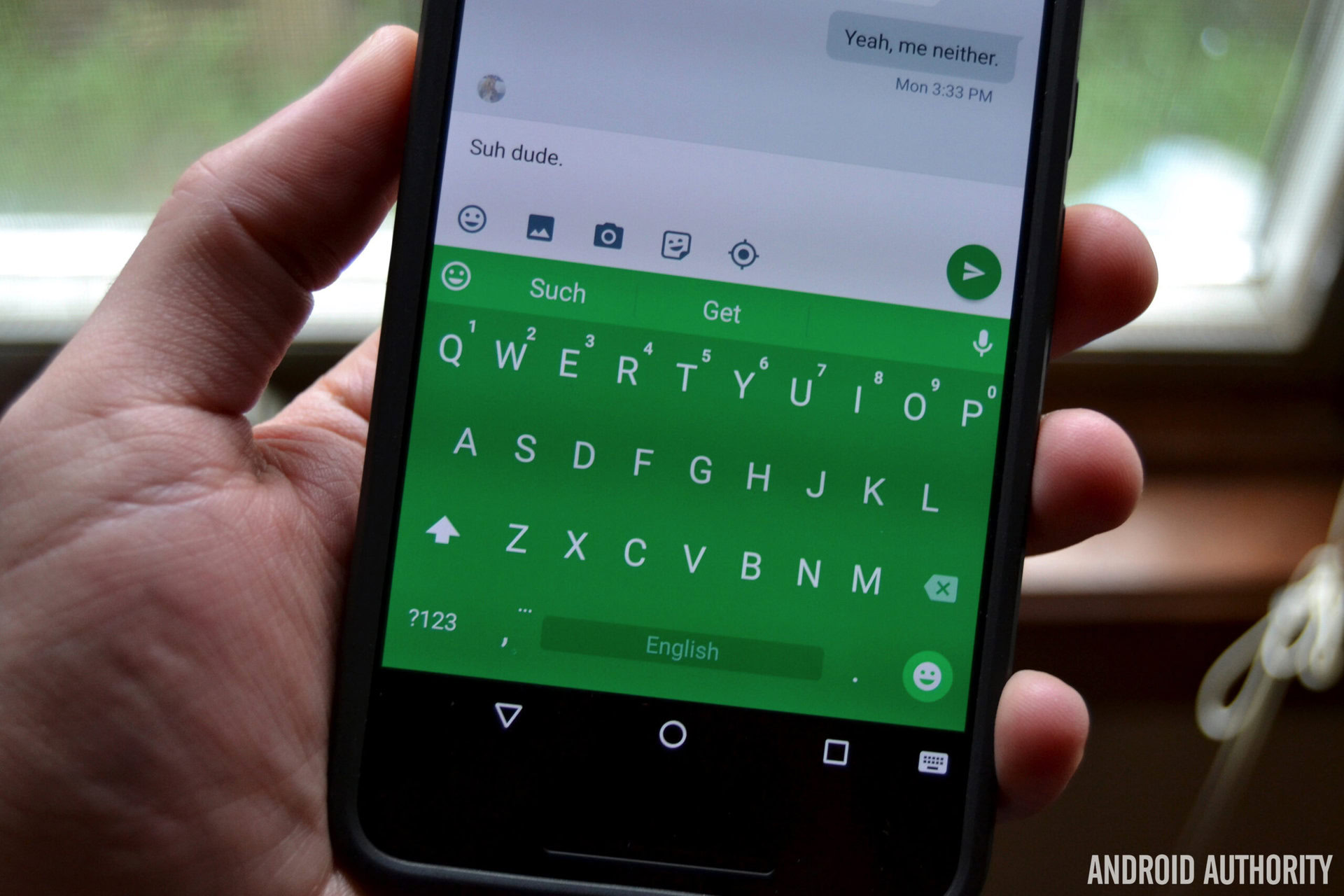
Another common feature of keyboards is to add sounds on each click. You can do this easily by adding a new method in your service and calling it onKey.
The nice thing is that Android actually provides some sounds for us ready to use, so we can do this very easily:
private void playSound(int keyCode){
v.vibrate(20);
am = (AudioManager)getSystemService(AUDIO_SERVICE);
switch(keyCode){
case 32:
am.playSoundEffect(AudioManager.FX_KEYPRESS_SPACEBAR);
break;
case Keyboard.KEYCODE_DONE:
case 10:
am.playSoundEffect(AudioManager.FX_KEYPRESS_RETURN);
break;
case Keyboard.KEYCODE_DELETE:
am.playSoundEffect(AudioManager.FX_KEYPRESS_DELETE);
break;
default: am.playSoundEffect(AudioManager.FX_KEYPRESS_STANDARD);
}
}
Now just use playSound() at the top of the onKey method and make sure to create a vibrator and audio manager (private AudioManager am; private Virbator v;). You could just as easily swap out the key sounds for your own in the assets folder, or change the duration and behavior of the virbration.
Closing comments
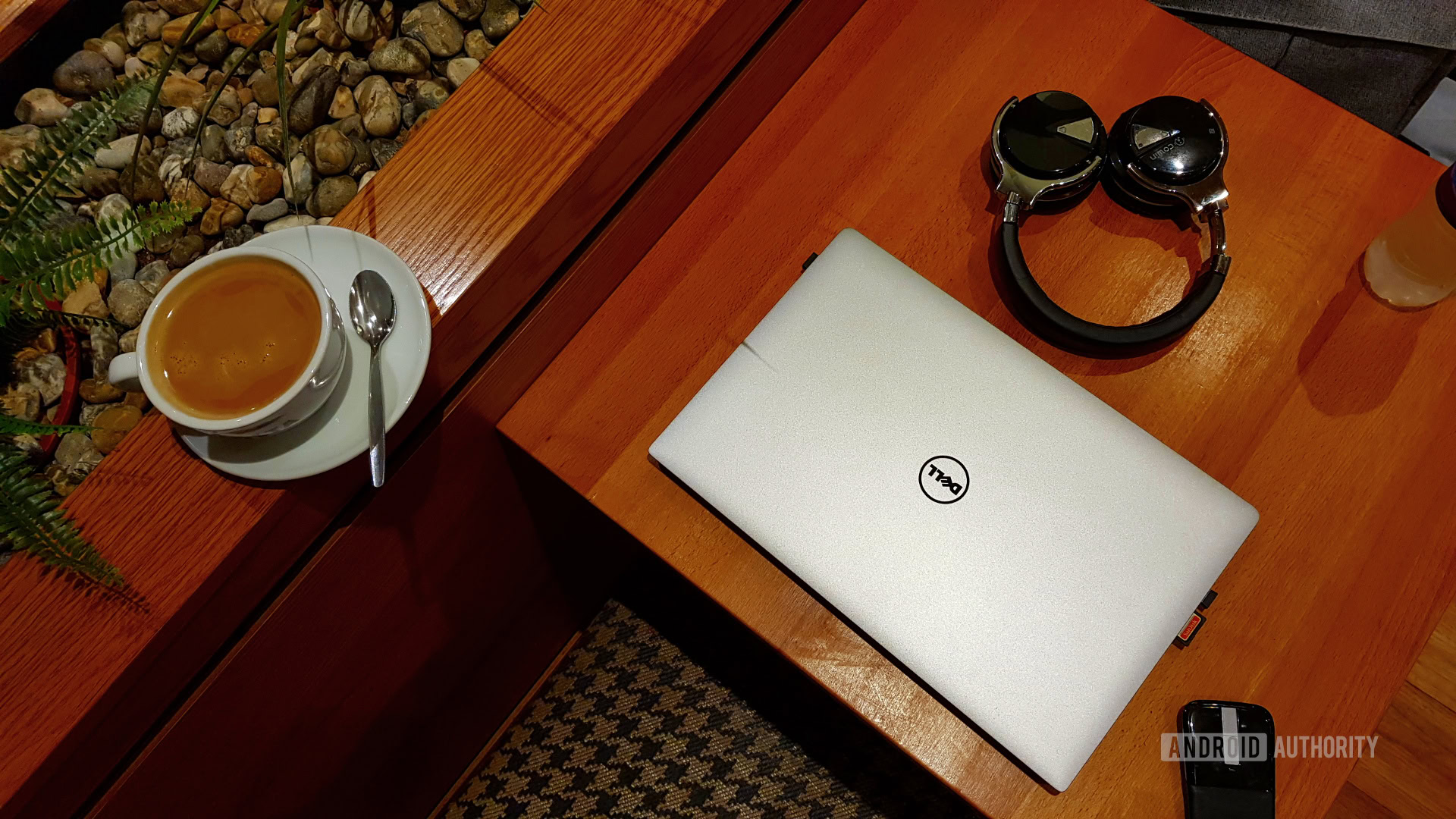
Now you have your very own custom keyboard! Another challenge ticked off of your Android development list. Play around with different key sizes, customization, and features to create the perfect typing experience.
Be sure to share your finished products in the comments down below! Happy text-inputting!