Affiliate links on Android Authority may earn us a commission. Learn more.
How to use if statements in Java - The key to understanding how to code
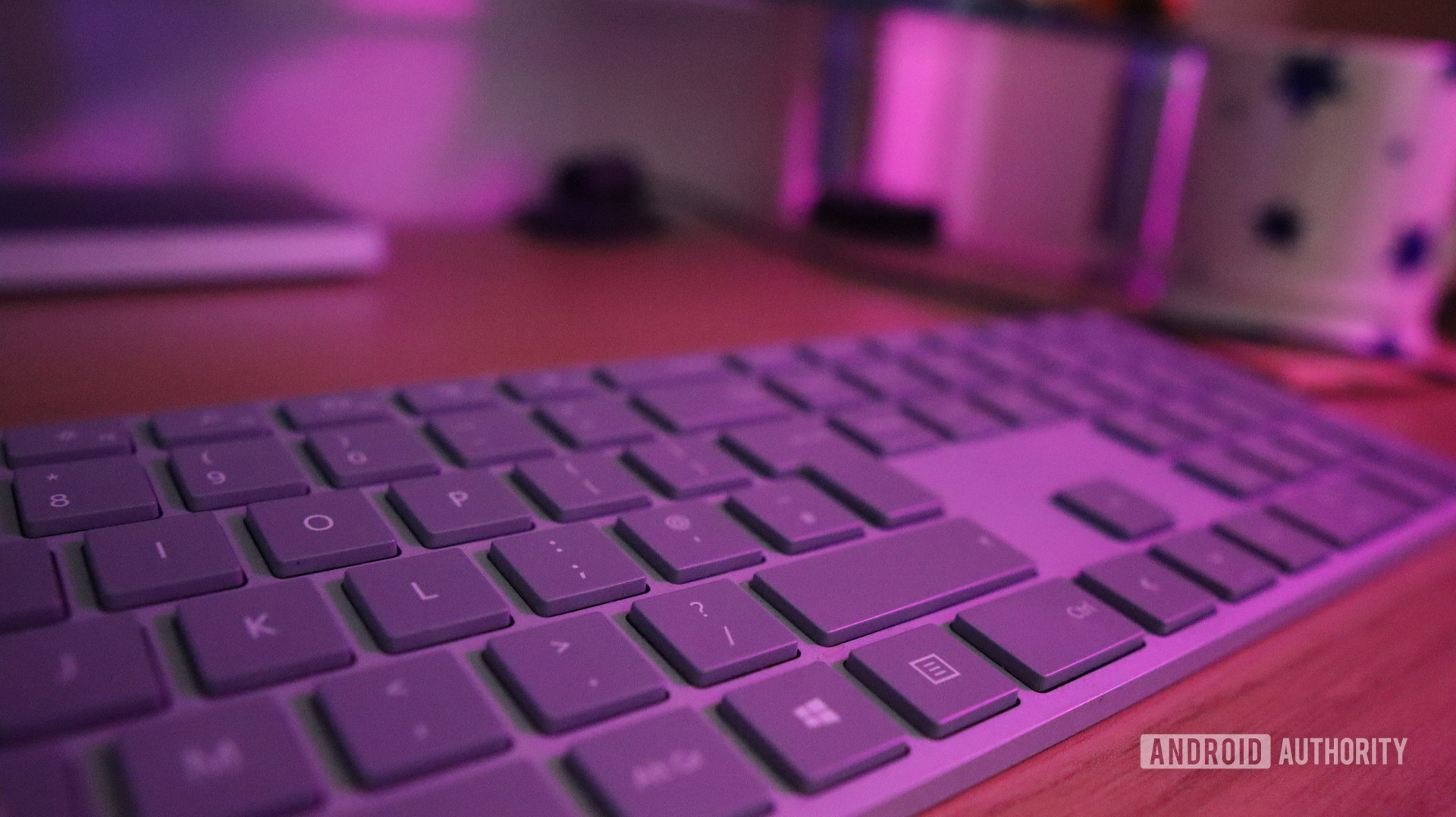
When I first learned to program, it was grasping if statements that helped it all click into place for me. Once you can use if statements, you can build all kinds of useful and interesting tools. Moreover, this is a basic programming structure that you’ll use in nearly every language.
In this post, we’ll explore how to use if statements in Java.
See also: How to use if statements in Python
Understanding if statements in Java
So, just what is an if statement in Java?
Essentially, an “if statement” is what you use for “flow control.” If statements allow for branching code that lets programs react dynamically to inputs.
The great thing about if statements, is that they do exactly what they say on the tin and work as you would expect. In “pseudo code,” you are essentially saying:
“IF (something happens) THEN (do something else)”
“IF (something happens) THEN (don’t do anything)”
Or:
“IF (something happens) AND (something else happens) THEN (do something)”
This might let you control a player’s health in a computer game (IF (enemy bullet hits player) THEN (reduce health by one point)). It’s also how you would end the game once the player runs out of health (IF (health is zero) THEN (show game over screen)).
You might even use if statements to control the character: IF (player presses the right arrow) THEN (move player right).
How to write if statements in Java
So, how does this look in actual Java code?
In Java, we don’t need to write “THEN.” Instead, we use an open curly bracket to say that everything that follows is part of that “code block” and should run under the conditions we specify. We then close the code block with the opposite curly bracket when we want to return to the main flow of the code.
Just like the pseudocode example I showed earlier, we’re still placing the test statement inside brackets. However, this will be written using operations to compare values.
For example:
int health = 2;
if (health == 0) {
System.out.println("Game Over!");
}
Here, as long as health is equal to zero, we will see the words “Game Over!” on the screen, otherwise we will not. “health” is a variable, which means it can store a value. Check out our recent post to learn more about variables in Java.
Nested if statements in Java
There are many other tricks you can perform once you understand how to use if statements in Java.
For example, you might also want to use “nested if statements.” This means you have an “if within an if” and can thereby create a vast range of conditions that are required for a particular action to take place.
You do this as simply as you might hope; by placing an if statement within the code block of another if statement. You can nest as many if statements as you like, just try not to lose track and remember to close the right number of brackets!
If you find yourself with an unwieldy number of these statements, you might wish to use another method, such as Switch.
And + or
Alternatively, you can set multiple conditions in the original statement using “AND” and “OR.” Again, these work as you expect. When you use and, you can use two separate conditions that must be required for the code to run. When you use or, your code block will run if either condition is met.
In Java, and is represented by two ampersands (&&) and or is represented by two vertical lines (||). These go inside the brackets to separate your test statements, and you can use as many as you like.
For example:
int health = 2;
int time = 0;
if (health == 0 || time == 0) {
System.out.println("Game Over!");
}
Else statements in Java
The last thing we’re going to look at today is how to use “else” statements. Else works in conjunction with if statements in Java by defining what will happen if the condition is not met.
We use the else keyword immediately after the closing bracket and then open up another
For example:
if (health == 0 || time == 0)
{
System.out.println("Game Over!");
} else {
System.out.println("Game On!"");
}
We can also use an “else if” which tests a new condition but only if the previous condition is found to be untrue.
For example:
if (health == 0 || time == 0)
{
System.out.println("Game Over!");
} else if (health == 1) {
System.out.println("Low health!"");
}
Again, we can use as many of these as we like!
Closing comments
So, there you have it: that’s how to use if statements in Java!
With this understanding, you can now create dynamic, changing, and interactive apps! To see how this fits into the “big picture” be sure to check out our free and comprehensive Java beginners’ guide. Or why not master the language in its entirety by checking out some of the best free and paid resources to learn Java?