Affiliate links on Android Authority may earn us a commission. Learn more.
Understanding variables in Java
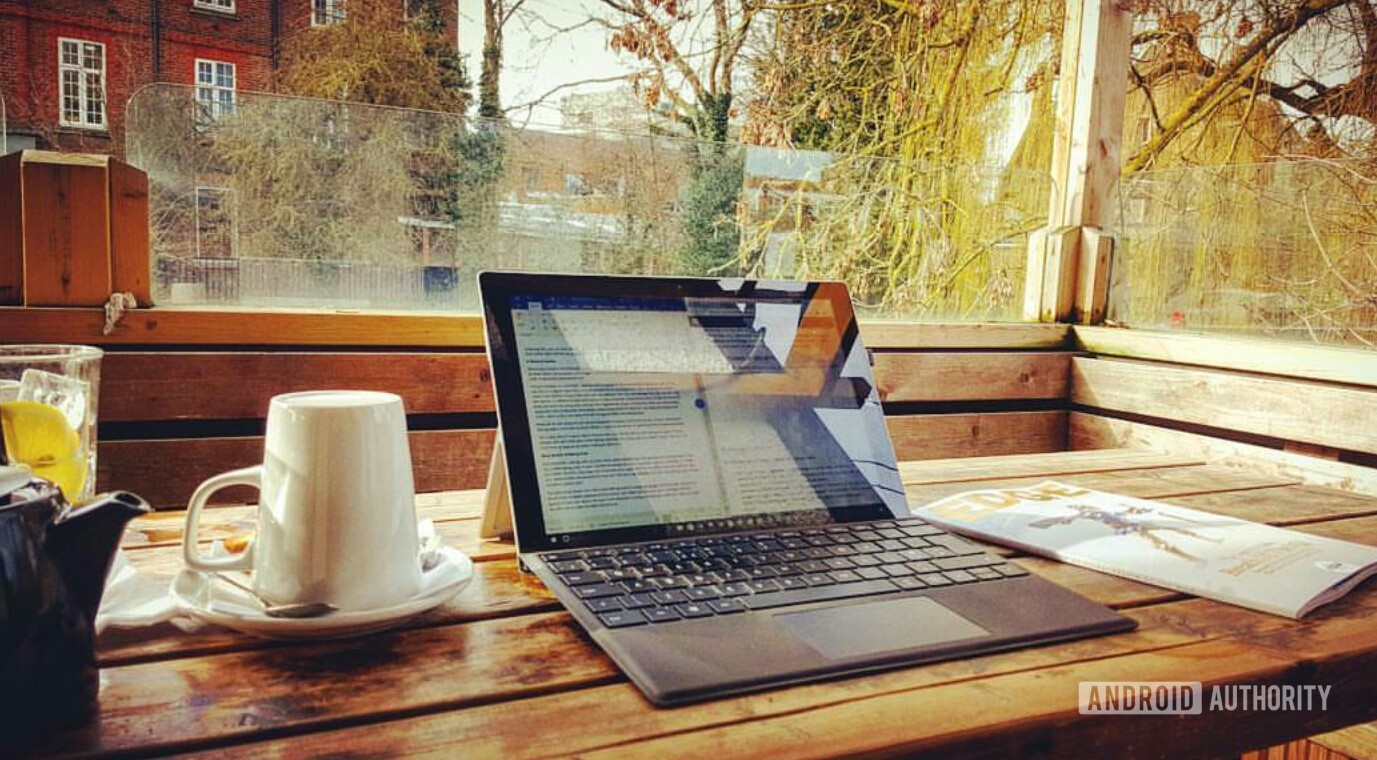
Variables are the bread and butter of coding. Without variables, apps would have no interactivity and no way of manipulating information. Therefore, learning about variables in Java should be among the very first things you do when picking up the language. In this post, you’ll find everything you need to know.
What are variables in Java?
The best way to understand variables is to think back to math lessons. You may recall solving algebra “problems” that looked something like this:
If 3 + n = 5, then what is n?
Of course, the answer is n = 2.
This is how variables work in programming. A variable is a label (usually a word) that can be substituted for a piece of data. This then allows us to ferry information around our app by getting values from other sources (like the web, or input from users) or to perform different functions depending on what value that variable contains.
For example, we might create a variable for a computer game called “health.” This would represent a number, which in turn would describe how much health a player had left. If the player gets shot, the health goes down (health = health – 1). If the player has no health, then the game ends.
Types of variable in Java
A variable that contains a whole number, as in the previous examples, is called an “integer” or “int” for short. However, this is just one type of variable in Java.
Understanding this is important, as we need to choose (declare) the type of variable when we first create it. This is because Java is “statically-typed” as opposed to a language like Python that is “dynamically typed.” There are pros and cons to each approach.
See also: Python vs Java: Which language should you learn and what are the differences?
When you declare your variable, you first write the type of variable you want, then the name you are going to give it, and then the value you are going to assign to it at its inception:
int health=10;
The other types of variables in Java are:
- byte – stores whole numbers from -128 to 127
- short – stores numbers from -32,768 to 32,767
- int – stores whole numbers from -2,147,483,648 to 2,147,483,647]
- long – stores an even wider range of whole numbers
- float – stores fractional numbers of around up to 6-7 decimal digits
- double – stores fractional numbers up to around 15 decimal places
- boolean – stores a binary true or false value
- char – stores a single alphanumeric character/ASCII value
These are referred to as “primitive data types” as they are built right into the functioning of Java and can’t be broken down any further.
The right variable for the job
Why are there so many different options for storing numbers? That’s because good programming should be efficient with memory. Bytes are allocated less memory than integers, so if you are absolutely sure that the value will never be higher than 127 or lower than -128, then you can safely choose to use them. However, because of Java’s strong typing, you need to know this for sure right from the start and declare the variable correctly. Using a Boolean is the most efficient of all, as it only takes up a single bit of information! You can use Booleans like “on/off” switches.
Good programming should be efficient with memory.
With that said, most casual programming will not need to be so efficient as to choose bytes over integers. It is often safe to use int for the majority of your whole numbers.
Strings and lists
If you have some familiarity with variables in Java, you might wonder why I left strings off of the list. A string is a series of alphanumeric characters and symbols that can be used to store names, phone numbers, or entire passages of text.
However, “string” is not a keyword in java but is actually a class. You don’t really need to know what this means, though our Java beginner course will teach you the basics.
For the most part, you are safe to use String just the same as any other variable. The main difference is that you will need to capitalize the word “String.” As a class, String also has methods, meaning that it can provide useful data about itself such as its length.
The same is true for other types, such as Arrays. Arrays in Java are variables that contain multiple values. These let you store things like lists of high scores or phone numbers and can also be organized, counted, and manipulated in other ways.
Aslo read: How to print an array in Java
Other types of variables in Java
There are other ways you can categorize variables in Java, and other ways you can manipulate data. For instance, a constant is a variable whose value never changes. This is primarily useful for writing more readable code.
Variables also act differently depending on how they interact with their class (instance variables vs static variables). You won’t need to understand these differences for a while, but stay tuned for more tutorials looking at these nuances.
Want to continue your education into variables in Java right away? Then, alternatively, see our guide to the best free and paid resources for learning Java.