Affiliate links on Android Authority may earn us a commission. Learn more.
How to reverse a string in Python
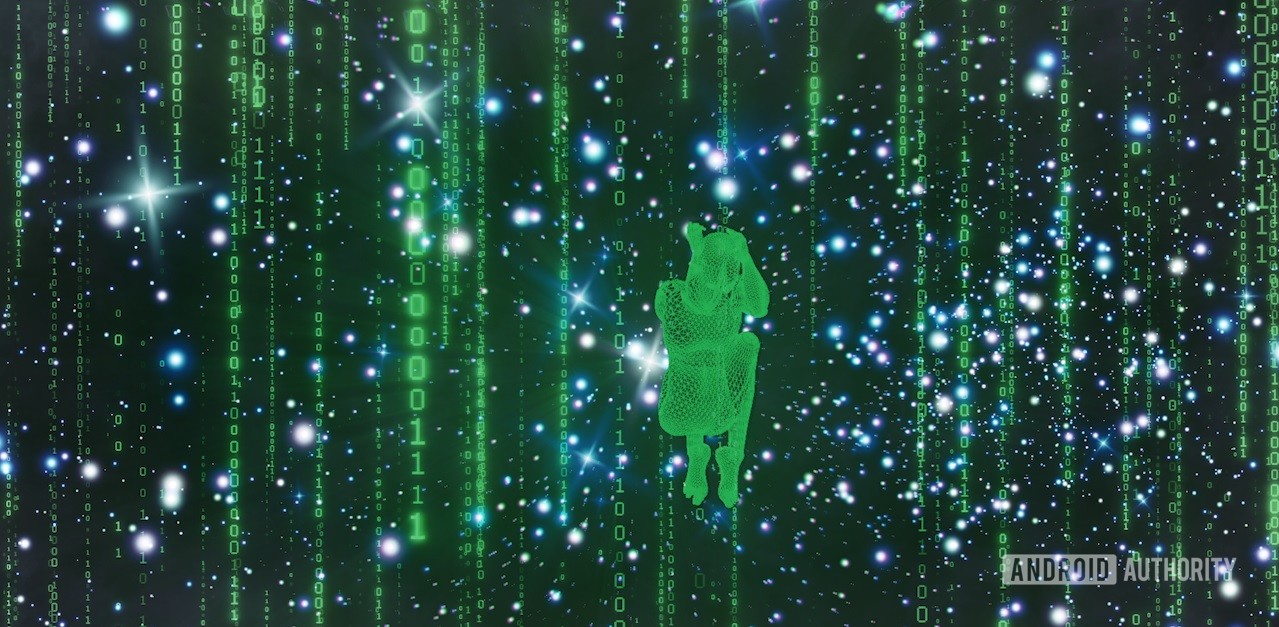
As there is no in-built function, if you want to know how to reverse a string in Python, you will need to use one of two McGyver techniques. Fortunately, these are still relatively straightforward and don’t take long at all to learn. Here’s what you need to know.
How to reverse a string in Python using slicing
The first way to reverse a string, is to use a slice that steps backward. Slices in Python let you return chunks of data from strings, tuples, and lists. This is useful if you ever want to get a few items from a list, or if you want to grab a chunk of a string.
Usually, a slice is used in order to provide a range such as “4-7”:
stringy = "Hello world"
print(stringy[4:10])
This will return “o worl”. Remember that the first value in a string or list has the index “0”. This is true for most programming languages. If we leave a number blank and just add a colon, then Python will refer to the first or last value respectively.
Also read: When to use lists rather than dictionaries in Python
We can also add a third number if we want to define the “step.” For instance:
print(string[4:10:2])
This will now show us the values 4-10, but will skip every other entry!
Which, of course, brings us the reverse step:
stringy = "Hello world"
print(stringy[10:4:-1])
Combine these two techniques and you get a slice that moves backward through the whole string:
print(stringy[::-1])
This starts and ends at the start and end of the string respectively and moves backwards. There you have it: that is how to reverse a string in Python!
How to reverse a string in Python manually
You could, alternatively, reverse your string manually. This might be useful if you ever need to check the values as you run through the string:
This method runs through the string in reverse, starting at the last position in the string. It then moves backward through each value, adding that value to a new string. The result is a new, reversed string.
stringy = "Hello World"
reversed_stringy = ""
index = len(stringy)
while index > 0:
reversed_string = reversed_stringy + stringy[index - 1]
index = index - 1
print(reversed_stringy)
This method is a little slower and more cumbersome, but it’s also more flexible to suit your needs.
Now you know not one but two methods for how to reverse a string in Python! Let us know which other methods you use in the comments down below. And don’t forget to check out our guide to the best online Python courses. There you can find some amazing deals on courses that will provide you with a full Python education; taking you from beginner to pro for a fraction of the usual cost!