Affiliate links on Android Authority may earn us a commission. Learn more.
How to print in Python
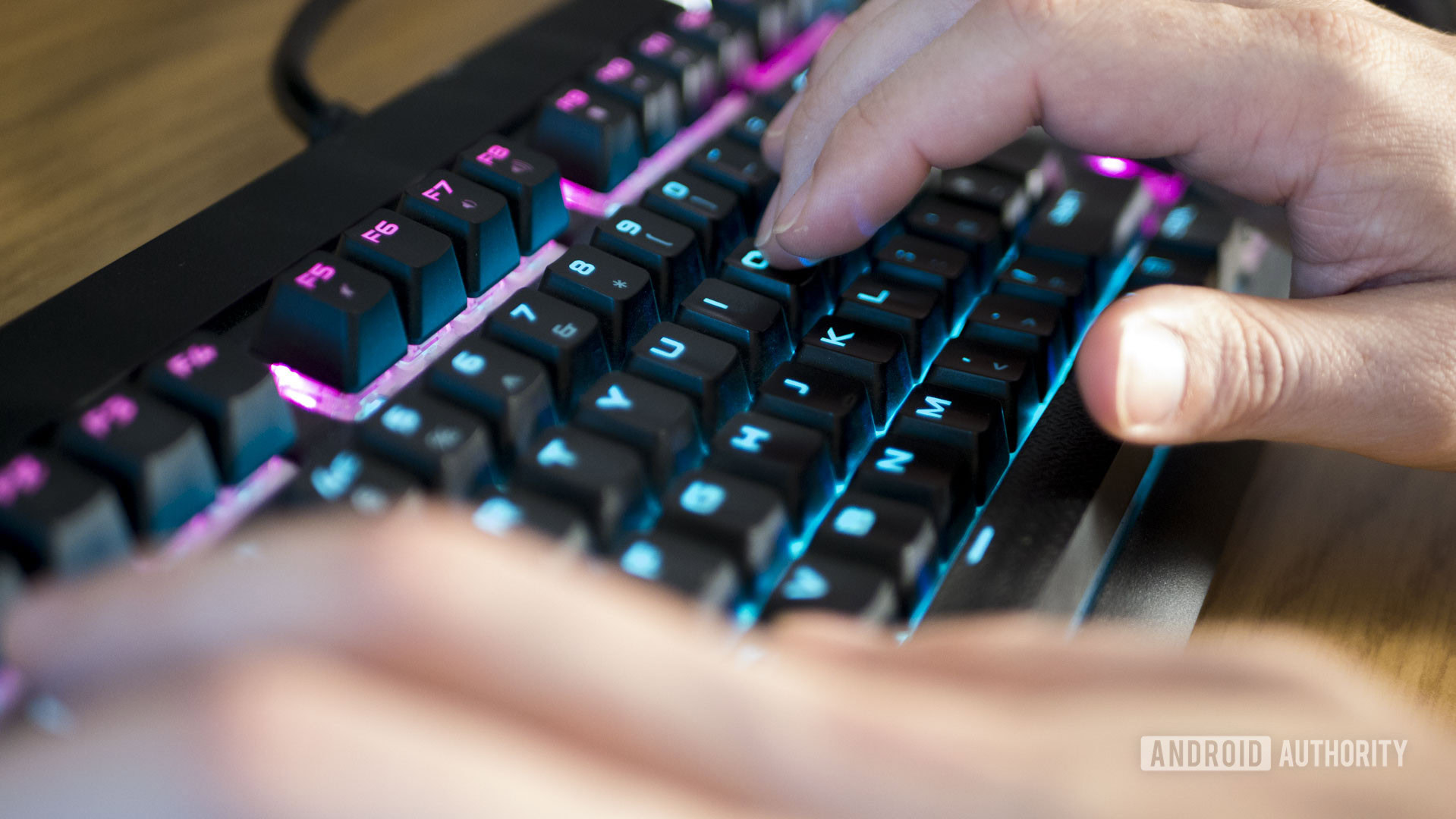
When it comes to learning to code in a new language, it makes sense to start at the beginning. Learning how to print in Python is most certainly the beginning.
The “print” command, as in BASIC, is what you will use in Python to display text on the screen. This is what we use to write “Hello World!” which is the first thing a programmer will traditionally do when working with a new language.
In this post, we will learn how to print in Python, and how to do a couple of (slightly) more advanced things, such as showing strings and other variables.
Also read: How to call a function in Python
How to print in Python 2 and Python 3
Printing in Python is extremely simple. All you need to do is to write:
print(“Your text here”)
However, this is very slightly complicated by the fact that there are two popular versions of Python. That example will work with Python 3 (the currently supported version), whereas you’ll need to drop the brackets if you’re using Python 2:
Print “Your text here”
Otherwise, that’s how to print in Python! Pretty simple, right?
Printing strings and other variables
When you place text inside quotation marks like that, you are creating a “string.” The word string in programming refers to any sequence of alphanumeric characters and symbols.
Strings can also be stored as variables. This means you will use a word in order to represent the string, and then refer to it later on in your code.
For example:
hello_world = “Hello world!”
print(hello_world)
This code stores the string “Hello world!” as helloWorld. We can then later print that text. To print a variable such as a string, we simply place it inside the brackets without the quotation marks.
Also read: How to install Python and start coding on Windows, Linux, or Mac
Why might you want to print a variable like this? Well, this becomes useful any time you think you might want the content you show to change during the course of your program. It’s also useful for getting information from elsewhere: such as when accepting inputs from the user.
name = input("Enter your name please!")
print("Hello " + name)
As you can imagine, this asks for the user’s name and then greets them personally. And this also shows you how to print in Python when combining a variable with some text. Simply close the quotation marks and then use the plus symbol. Notice that I inserted the space? Another way you can do this is to separate the elements you want to print using a comma. For example:
print ("Hello", name)
How to print in Python – A few more tricks
If you want to print in Python and you want to include a new line, a good way to do that is to use the character “\n” as part of your string. Another option is to follow your print statement with another blank print statement:
print("Hello")
print()
print(name)
Finally, note that you can use either double or single quotes. This will then allow you to include those characters within your string:
print('He's "really" good at it!')
And if you want to use both types of quotes, you could solve this by using triple quotation marks:
print("""I said "Hello" and I'm still waiting for them to get back to me""")
And that’s how to print in Python! Let us know if we missed anything, and happy printing!