Affiliate links on Android Authority may earn us a commission. Learn more.
How to use for loops in Java
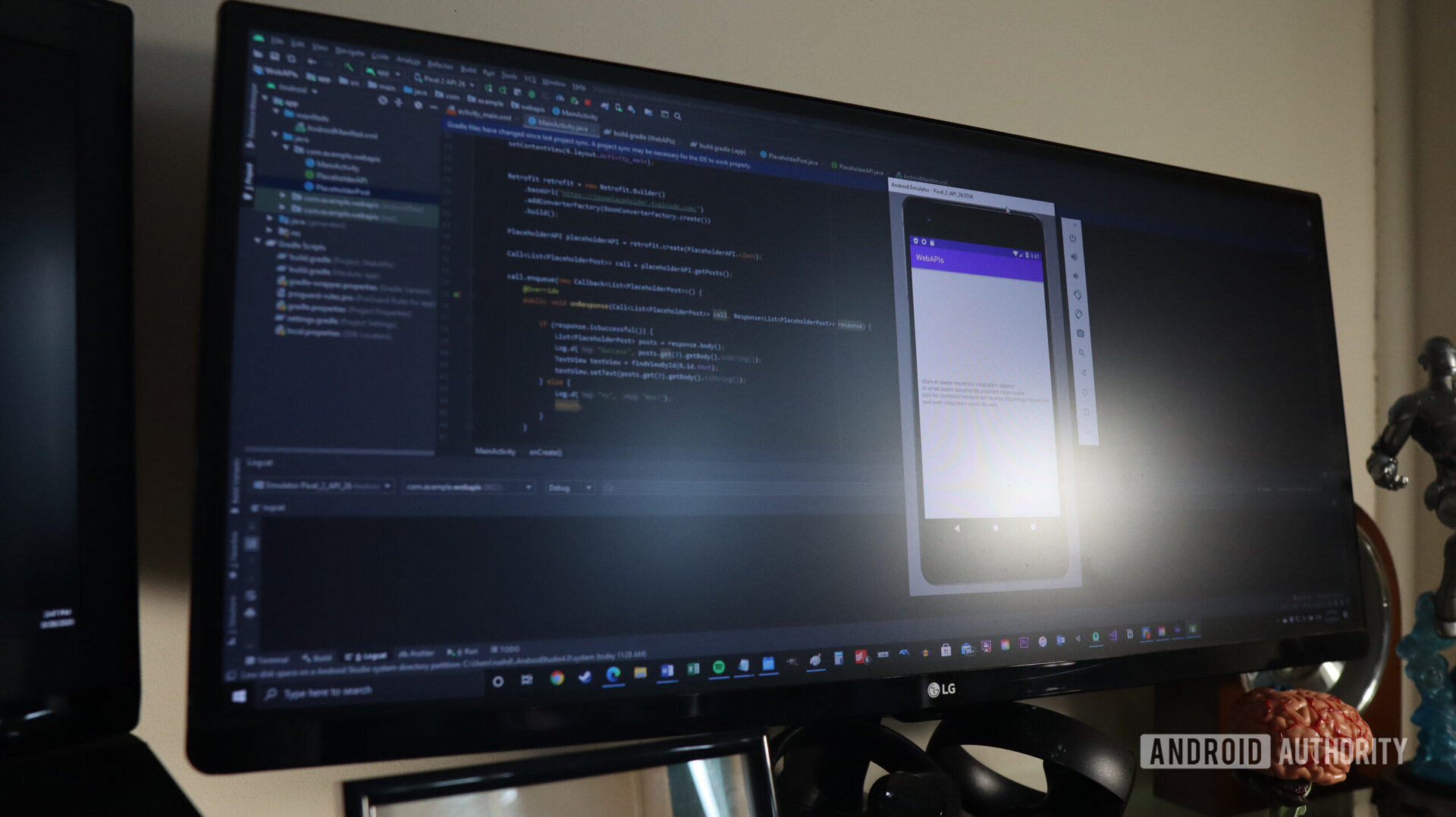
For loops in Java are just one type of loop that can be used to repeat a code block for iterative operations. If you need to open a text file for example, then you might use a loop to go through each line of the document and print it to the screen.
Likewise, games often use a “game loop” which loops around every time the frame is refreshed to check for inputs and update the positions of enemies, physics items, etc.
See also: How to use loops in Java
For loops in Java are extremely powerful and lend themselves to many different coding applications. In this post, we will explain how to use them, and look at more advanced concepts such as labelling.
How to use for loops in Java
What sets a for loop apart from other types of loop in Java, is that it has a fixed number of iterations. The syntax for a for loop in Java is:
for (declare variable; condition; increment) {
Everything inside the curly brackets (code block) will then be executed repeatedly until the condition is met. For example:
for (int n=1; n<=100; n++) {
System.out.println(n);
}
This will count to 100 and print the numbers to the screen.
Break and continue
Now you understand the basics of for loops in Java. However, there are a number of more advanced concepts that can help you to write smarter code and better loops.
For example: break and continue.
Break is a keyword that will end the loop at any point. This could be useful if you want to let the user hit “esc” to stop the game for instance.
for (int n=1; n<=100; n++) {
System.out.println(n);
if (n == 30) {
break;
}
}
Continue, meanwhile, will restart the loop at the beginning. This means you can choose to skip a portion of the code block for a particular iteration.
In a game scenario, this might be useful if the player presses “pause.”
Nested for loops
There is nothing to stop you from playing a for loop inside a for loop. This is referred to as a “nested loop” and you can repeat this process as many times as you deem necessary.
for (int n=1; n<=10; n+=10) {
System.out.println(n);
for (int i=1; i<=100; i++) {
System.out.println(i * n);
}
}
The following will count to 100 but show the number “1” twice.
The only problem when doing this, is that if you use break or continue at any point, you will break out of every level.
Labels are a useful tool that can be used with for loops in Java. Labels allow you to choose precisely which loop you want to break and where you wish to go in your code. You use them simply by choosing a name for your loop and then adding them just before your loop code using a colon.
public static void main(String []args){
outerloop:
for (int n=1; n<=10; n+=10) {
System.out.println(n);
innerloop:
for (int i=1; i<=100; i++) {
System.out.println(i * n);
if (i == 50) {
break innerloop;
}
}
}
}
Now you’re in the loop!
Now you should have a handle on how to use for loops in Java.
Don’t forget to check out our list of the best resources to learn Java if you want to really develop your coding skills. There, you’ll find courses like the Complete Java Bundle, which Android Authority readers can sign up to for just $39. That’s a huge 96% discount on the $