Affiliate links on Android Authority may earn us a commission. Learn more.
How to use loops in Java
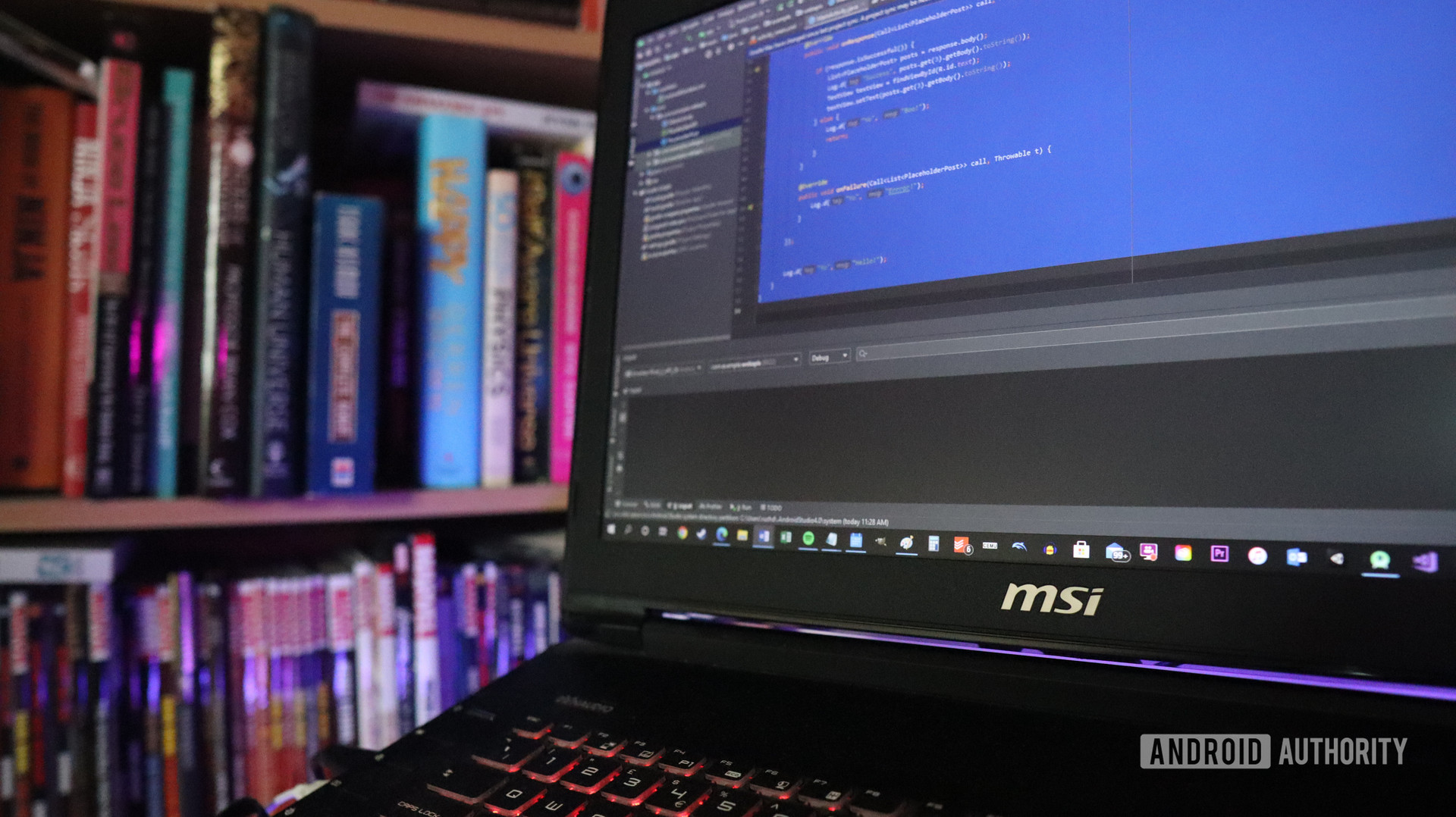
A loop is a structure in programming that allows you to run the same section of code over and over. This can be used when you want to perform an iterative task (like counting, or sorting through a list) or to create an ongoing, cyclical experience for the user (as in a game loop). In this post, we will examine how to use loops in Java.
Also read: How to use loops in Python
For loops in Java
There are three types of loops in Java:
- For loops
- While loops
- Do-while loops
Starting with for loops, this is the best option for iterative tasks. The reason is that for loops have a fixed number of iterations. We write this out by first initializing a variable, then setting the condition, and finally defining the increments.
For example:
for (int n=1;n<=10;n++) {
System.out.println(n);
}
Here, we are using the integer “n”. The loop will run as long as n is smaller than or equal to 1. The value of n will increase by one on each iteration.
Run this code and it will simply count from 1-10 on the screen.
If we said:
for (int n=1;n<=20;n+=2) {
System.out.println(n);
}
We would see the numbers 1-19, counting in twos! And so on.
And we don’t necessarily need to use integers:
for (String hi = "hi";hi.length()<=10;hi = hi + "h") {
System.out.println(hi);
}
While loops in Java
While loops work just like for loops, except you can determine any condition using values you may have defined elsewhere in your code. To use while loops in Java, you simply need to add the condition in brackets.
int health=10;
while(health > 0) {
System.out.println(health);
health--;
}
}
Do while loops
A do while loop is very similar to a while loop, except that the structure is turned upside down so that the code block always runs at least once.
do{
System.out.println(health);
} while(health > 0);
Here, you’ll see “health = 0” if the health is lower than one.
Which loop to use when?
If all these different loops are driving you loopy, it may be helpful to understand why we have three approaches to do essentially the same thing.
- For loops are useful when you have a fixed number of iterations. For example, this is a good way to run through a text document line-by-line. (First you would need to get the number of lines.)
- While loops are useful if you need something to run indefinitely until a certain condition is met. A great example might be your game loop, which could run until the player hits “exit” or runs out of health. Check out our post on how to write your first Android game in Java for a demonstration of how that might work.
- Finally, do while loops can be used when you want to run a code block at least once. For example, in many user interface scenarios, it can be useful to tell the user when there is nothing to perform.
Of course, there are workaround ways to do any of these things with any of the different loops in Java! But choosing the right code for the job is the hallmark of efficient programming.
Java break and continue statements
Before you can claim to have mastered loops in Java, you need to get to grips with the “break” statement. A break statement is your “get out clause” that effectively allows you to cancel the loop at any time.
For example, you might want to run your game loop until the user dies, but likewise, allow them to exit at any point. For this, you could use the break statement:
int specialCondition = 3;
int health=10;
while(health > 0) {
System.out.println(health);
health--;
if (specialCondition == 3) {
System.out.println("Special condition met!");
break;
}
}
The “continue” statement works in a similar manner but allows you to return to the start of the loop, cutting off anything below. This can be useful for avoiding complex, nested if statements within your loop.
There are other fancy tricks we can pull off, such as nesting loops and using labels. These are topics we may return to in future. For now, you know all the essential skills you need to start looping!
Also read: How to print an array in Java
Want more? Then check out our free, comprehensive Java beginner course to gain a big-picture understanding of the language. Alternatively, you can find plenty of full courses by checking out our list of the best resources to learn Java.