Affiliate links on Android Authority may earn us a commission. Learn more.
Anatomy of an app: An introduction to activity lifecycles
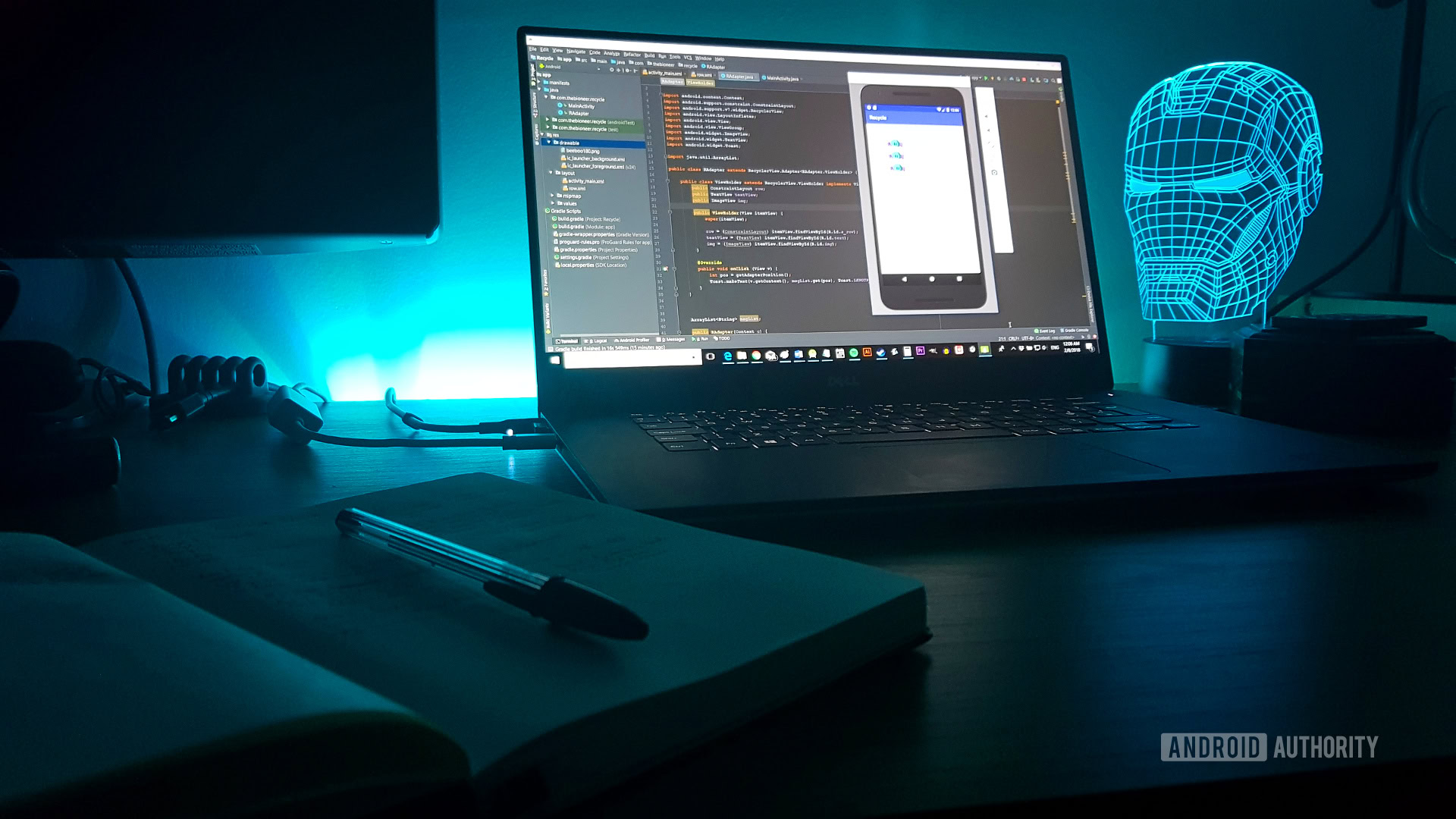
When you first boot up a new Android Studio project, you’re greeted not with a blank page, but a whole bunch of what is known as “boilerplate” code. This is code needed for the vast majority of apps and to get that “Hello World” example up and running.
But what does it all mean? What is an onCreate() anyway?
If you have ever tried reverse-engineering a project or following instructions, you’ll likely have spotted that a lot of the same lines come up time and again: things like onPause(). What does all that mean?
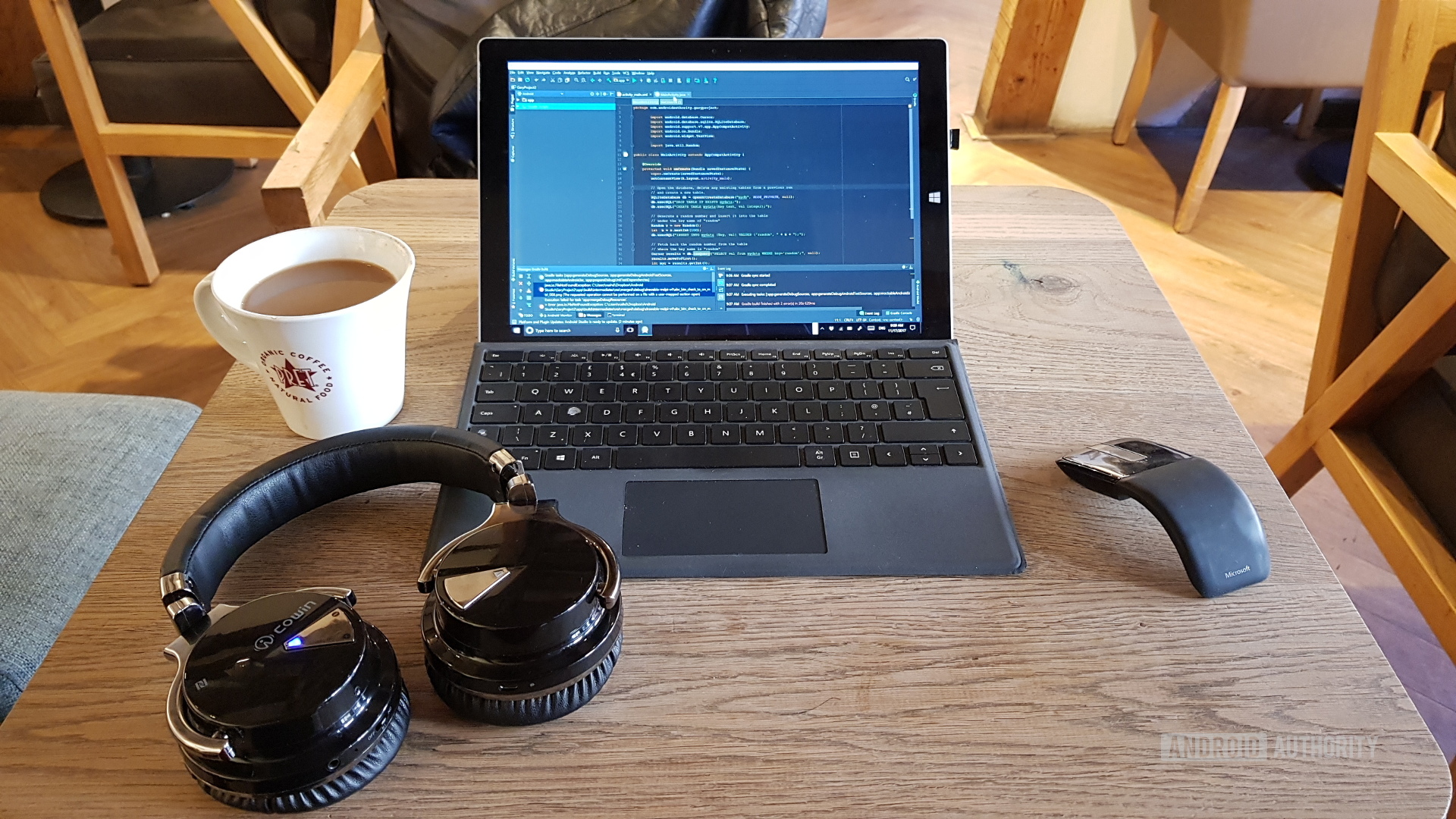
Let’s look at the Android activity lifecycle — how it handles things like screen rotations, or being sent to the background while the user does something else. As we explore this concept, you’ll discover what a lot of these common lines of code mean and why they need to be there.
The Android activity lifecycle
Android apps — more specifically Android activities — go through a number of stages from when they’re first loaded to when they get closed down.
These are handled as “events” inside your code when the user changes your app’s state in some way: by launching it, pausing it, closing it, and so on. Events run in methods (sequences of instructions inside curly brackets) and this means that we can then hijack those methods and decide what we want to happen at each stage. This is where the line @Override comes from: it means we’re using a method that isn’t brand new, but rather one that would always have executed (usually inherited from the superclass, which will be explained below) and we’re just hopping on and adding some tweaks.
For a typical activity, the key events include:
- onCreate
- onStart
- onResume
- onPause
- onStop
- onDestroy
onCreate() is called when the activity is first brought to life. Apps use onResume() when returned to focus after another event. Apps only pause (via onPause()) in a few specific scenarios, like during multiscreen operations or while using transparent apps on top. An app calls onStop() if it’s sent to the background while another app is opened. In here, we might stop any memory hungry processes that aren’t necessary while the app is not visible. When a user completely shuts an app down, or when the system shuts it down to conserve memory, it calls onDestroy(). Changing an app’s configuration — like rotating it — also calls onDestroy(), followed immediately by calling onCreate() again to build a new Activity. You can differentiate between the two functions with isFinishing.
The line super.onSaveInstanceState(); tells Android to save the “state” of all of our views so all the text fields will still contain the same text and any elements that have moved around the page will still be where our user last left them if an app is destroyed via rotation. If you don’t include that line, then some strange things can happen. Here, the word super tells us we are calling a method from the superclass — in this case AppCompatActivity.
This graphic from Google showing the Android activity lifecycle can help:
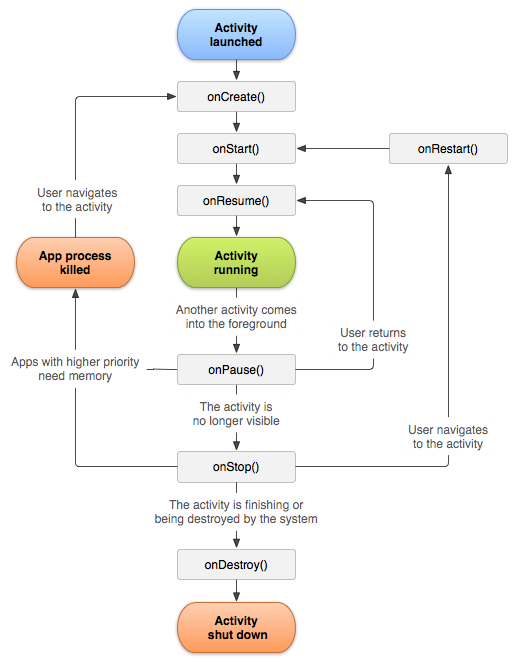
Notice how we also override the onClick method belonging to the Button. The button is handled by a separate class (hence our need to import it) and we’re once again hijacking the code to define what happens when the button gets clicked ourselves!
Inheritance and AppCompatActivity
This is the next line we’ll put under the microscope:
public class MainActivity extends AppCompatActivity {
This line essentially defines our code as a class. It’s what implements the Android activity lifecycle. A class is a module of code that works elsewhere and has specific properties we’ll discuss in a future post (technically it describes an object). Because the class is described as public, we could theoretically access its methods from other classes too.
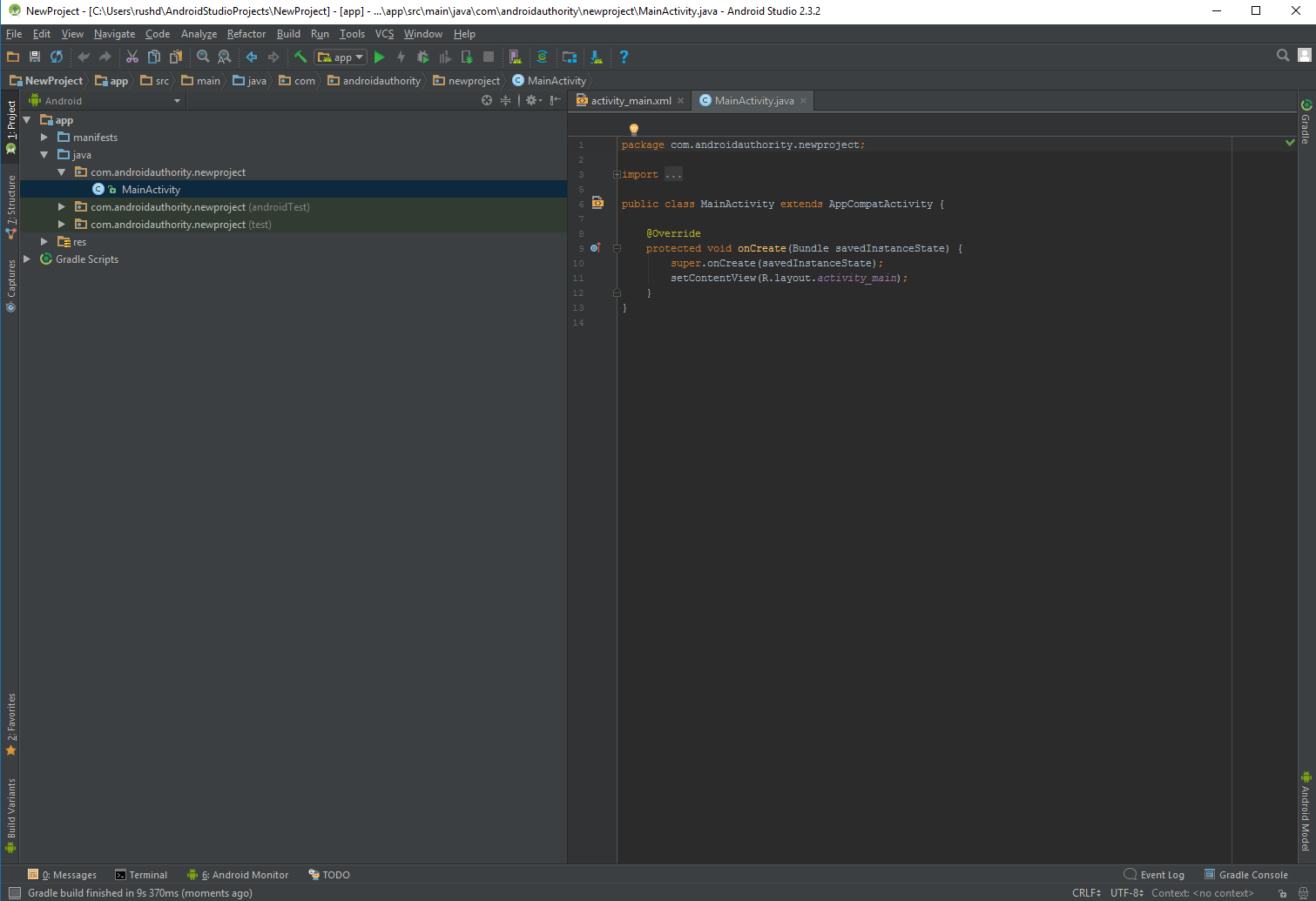
The next part of the line, extends AppCompatActivity, means that we’re inheriting properties from another class. This gives us access to additional methods that define the behavior of our activity. Methods such as onCreate and findViewByID. This line is what takes our Java code and turns it into an Android activity! We “inherit” the methods making the code behave as such, which lets us access them in our code using @Override as you saw earlier. It’s all starting to make sense! Maybe. Probably not.
Technically, our activity class is really a “subclass” of AppCompatActivity. This is the way we actually want to handle most of the classes we intend to use as activities, as it will allow them to behave the way activities are supposed to behave with the functionality expected of an app. There are exceptions however, like when you might want to extend Activity instead of AppCompatActivity. This removes features like the action bar, which can be helpful in game development. It still builds an activity, just a slightly different kind. It’s only when you start creating activities that purely handle data, or execute tasks in the background, that you will start using other types.
Most activities you create are going to feature these statements and will override the same methods. Each new screen you create is automatically populated with this code and which is what all that boilerplate code is telling us.
Summary
This explains how your activities will run and why certain elements in your code need to be there. Hopefully, you now have a bit more understanding of how things are operating behind the scenes and what is meant by the term Android activity lifecycle.
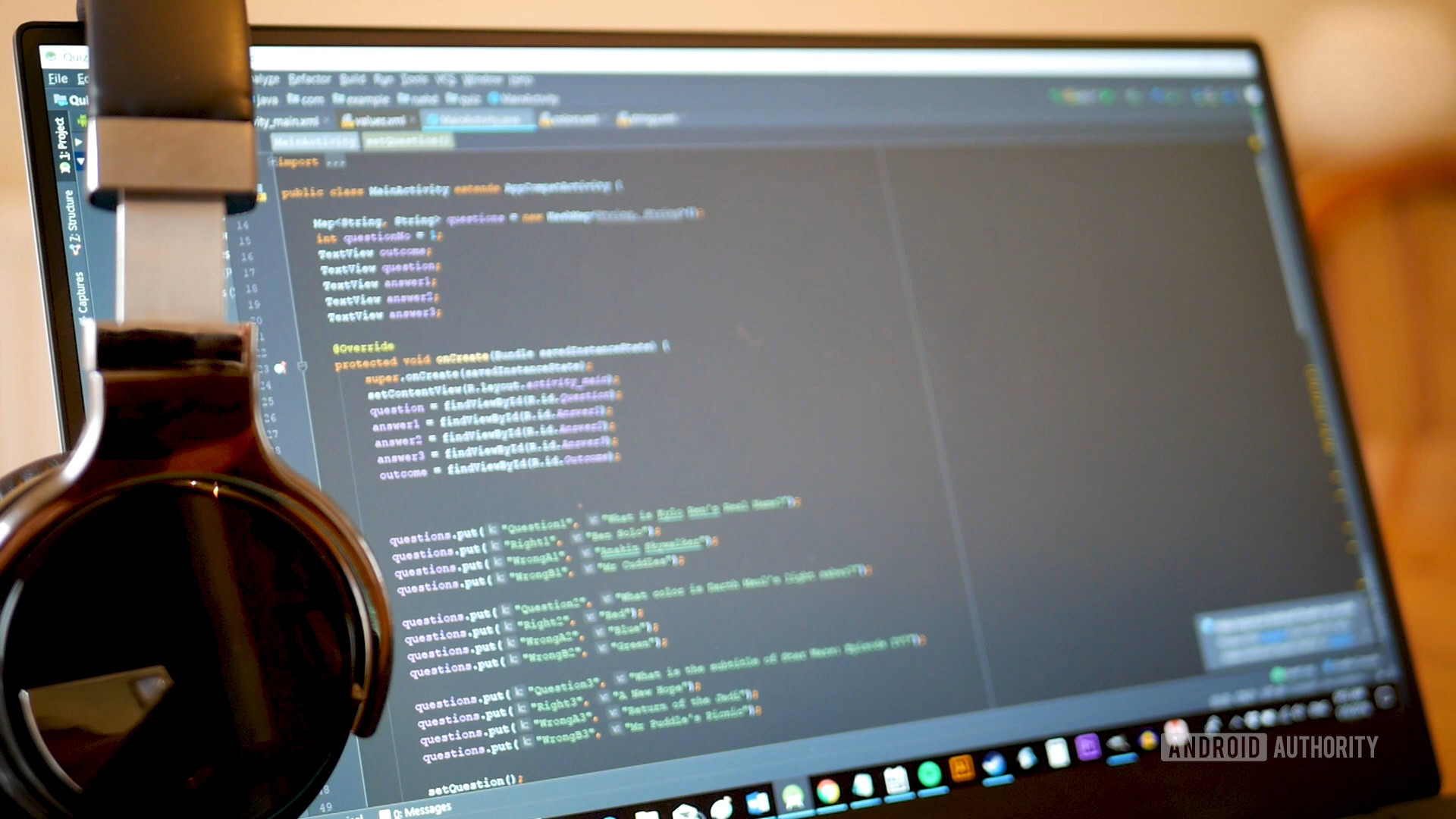
To get a fuller grasp of this, stay tuned for an upcoming post where I’ll be looking at launching new activities from within your app. There, we’ll see how to set up a new activity with inheritance and demonstrate how an app would handle multiple Android activity lifestyles.
Related
- I want to develop Android Apps — What languages should I learn?
- Best Android developer tools
- Cross-platform mobile development — challenges, options, and why you should consider it
- Android Go: Getting your app ready for Google’s new, slimline OS
- The best free and paid Android app development courses
- A very simple overview of Android App development for beginners
- Android SDK tutorial for beginners