Affiliate links on Android Authority may earn us a commission. Learn more.
Try catch Java: Exception handling explained
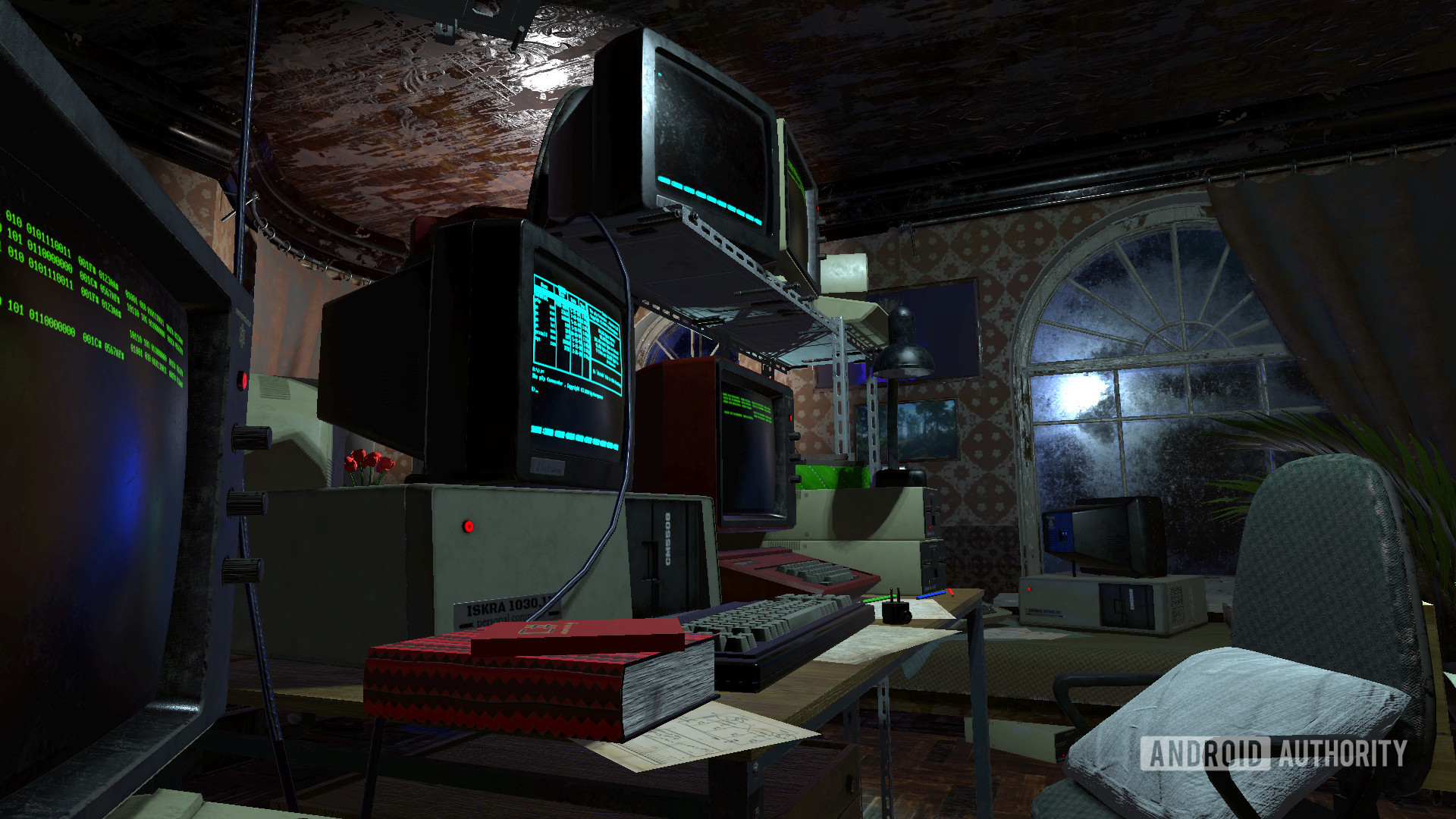
Error handling, also called exception handling, is a big part of Java, but it’s also one of the more divisive elements. Exception handling allows a developer to anticipate problems that may arise in their code to prevent them from causing issues for users down the line. The reason this can become a nuisance is that some methods in Java will actually force the user to handle exceptions. This is where “try catch” in Java comes into play.
What is “try catch” Java?
For someone new to programming, it can be hard to understand why you might write code that makes it possible for an error to occur.
See also: NullPointerException in Java – Explaining the Billion Dollar Mistake
A good example would be the FileNotFoundException. This does exactly what it says on the tin: this exception is “thrown” when Java looks for a particular file and can’t find it.
So, what happens if someone is using your app, switches to their file browser, then deletes a save-file that the app was using? In that scenario, your application might understandably throw an exception. We say that this is an exception rather than an error because it’s a problem that we might reasonably anticipate and handle.
So you use a “try catch” block.
Try essentially asks Java to try and do something. If the operation is successful, then the program will continue running as normal. If it is unsuccessful, then you will have the option to reroute your code while also making a note of the exception. This happens in the “catch” block.
Try catch Java example
Here’s an example of using try catch in Java:
try {
int[] list = {1, 2, 3, 4, 5, 6};
System.out.println(list[10]);
} catch (Exception e) {
System.out.println("Oops!");
}
Here, we create a list with 6 entries. The highest index is therefore 5 (seeing as “1” is at index 0). We then try to get the value from index 10.
Try running this and you will see the message “Oops!”.
Notice that we passed “Exception e” as an argument. That means we can also say:
System.out.println(e);
We will get the message: “java.lang.ArrayIndexOutOfBoundsException: 10”
See also: Java beginner course – A free and comprehensive guide to the basics of Java
Now that we have “handled” our exception, we can refer to it as a “checked exception.”
Forced exception handling
Notice that we could have written this code without handling the exception. This would cause the program to crash, but that’s our prerogative!
In other cases, a method will force the user to handle an exception.
So, let’s say that we create a little method that will check the tenth position of any list we pass in as an argument:
public class MyClass {
public static void main(String[ ] args) {
int[] list = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11};
System.out.println(checkTen(list));
}
public static int checkTen (int[] listToCheck) {
int outPut = listToCheck[10];
return outPut;
}
}
This works just fine and will print “11” to the screen. But if we add the “throws” keyword to our method signature, we can force the user to deal with it.
public static int checkTen (int[] listToCheck) throws ArrayIndexOutOfBoundsException {
Now we can write our code like so:
public class MyClass {
public static void main(String[ ] args) {
int[] list = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
try {
System.out.println(checkTen(list));
} catch (ArrayIndexOutOfBoundsException e) {
//System.out.println(e);
System.out.println("Oops!");
}
}
public static int checkTen (int[] listToCheck) throws ArrayIndexOutOfBoundsException {
int output = listToCheck[10];
return output;
}
}
This will then force the user to deal with the exception. In fact, many Java editors will automatically populate the code with the necessary block. Note that we need to use the right type of exception!
So, should you force other devs to handle exceptions when writing your own classes? That’s really up to you. Keep in mind that some scenarios really should cause a program to terminate, and forcing a developer to deal with such instances will only create more boilerplate code. In other cases, this can be a useful way to communicate potential issues to other devs and promote more efficient code.
Of course, in the example given here, there are a number of other possibilities for exceptions. What happens if someone passes a list of strings into your method, for example? All I can say to that is, welcome to the wonderful world of Java!
This is your chance to decide what type of developer you want to be! Once you’re ready to find out, check out our guide to the best resources to learn Java!