Affiliate links on Android Authority may earn us a commission. Learn more.
How to use Python modules
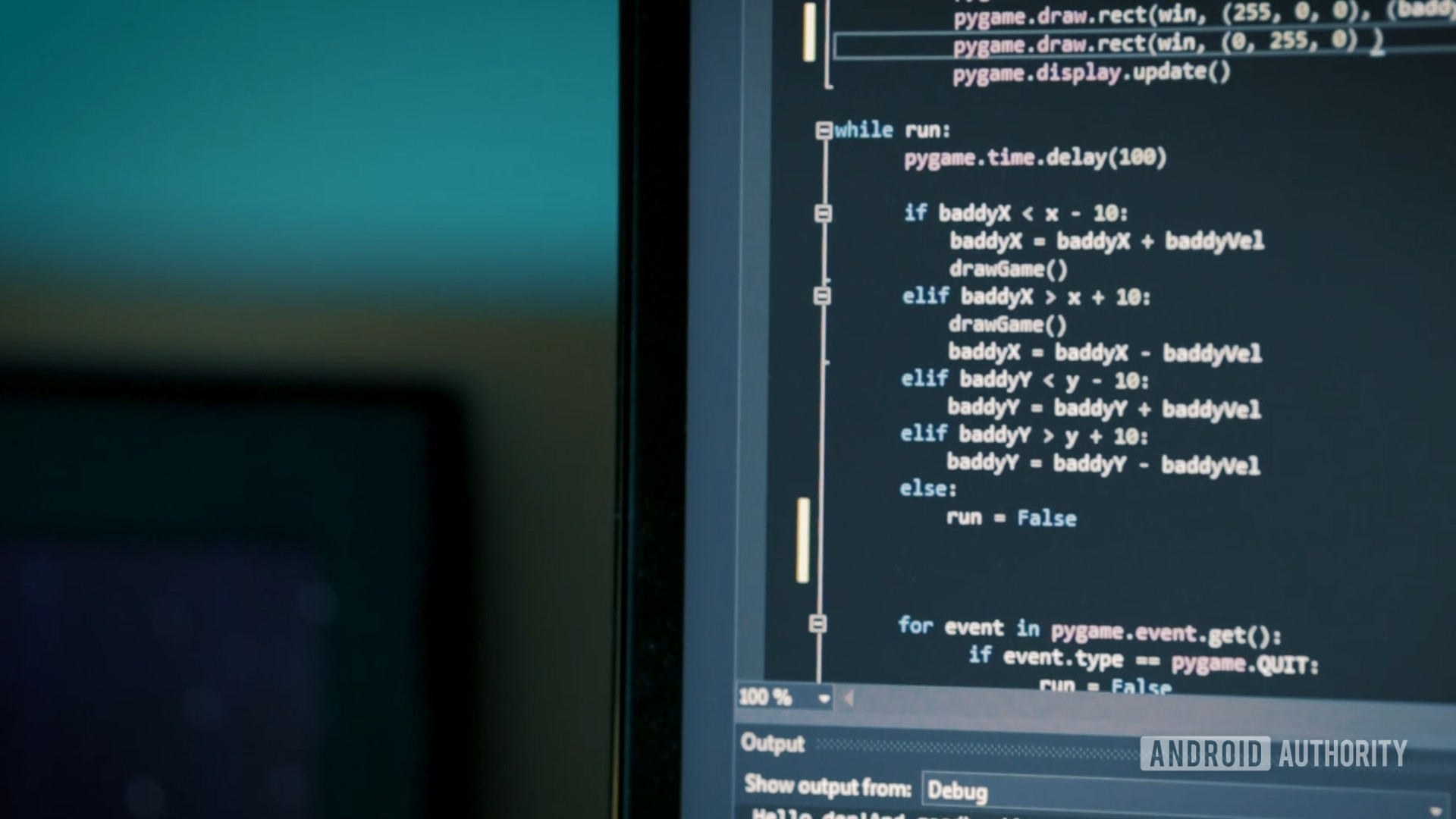
Once you know how to add and use a Python module, you will greatly extend the capabilities of the language.
A Python module is an external class or set of functions that exist outside the main file of your program. This can be something that you built yourself, or it can be provided by the community. Either way, the modular nature of Python means these elements can be used to seamlessly extend the capabilities of an application, or to share utilities you’ve built across multiple projects.
In this post, we’ll explore how to add and use a Python module.
How to use built-in modules
Your basic Python installation comes with a host of Python modules that are ready to use. These provide basic functionality that a large proportion of programmers will rely on.
Also read: How to install Python and start coding on Windows, Mac, or Linux
For example, if you want to generate a “pseudo random number” (“pseudo” because there is no such thing as a random number in programming), then you will need to lean on a module called “random.” This is ready and available, so all you need to do is to add the following line to your code:
import random
From here, you will be able to access functions that belong to that module. For example:
import random
n = random.randint(1, 5)
return n
This gives us a number between one and five!
How to get new modules
One of the keys to effective programming is learning not to “reinvent the wheel.” That is to say, that if you need to perform a particular job in your code, there’s a good chance that someone has done precisely that before. You could waste time figuring out how to do it yourself, or you could just use the code that someone else provided for free! Guess which is a smarter use of your time?
The great news is that you don’t need to copy and paste code you found on a forum into your project! Instead, you can just find the Python module that does what you need it to, then grab that to use in your code.
First: search for the thing you want to do. For example, if we want to open Word documents in Python, we could just Google:
“How to open Word documents.”
What we’ll quickly find, is that there is a module available for that, called docx.
To get that Python module, we will use a tool called “pip.” We covered how to use this in our comprehensive beginners’ guide to Python. To access pip open up the terminal or load a command prompt in the directory where Python is installed (unless you added Python to path, in which case that latter point doesn’t matter!).
Now type:
“python –m pip install docx”
Now add this to your code:
import docx
You’ll now be able to access all the functions from this module: such as opening and writing doc files.
Alternatively, if you are using PyCharm, you can simply type that line, and the click on the underlined text and select “install package.”
What’s the difference between a Python module, class, or package?
You might be wondering what a Python “package” is, and how it relates to the Python module. Essentially, a package is a group of modules with an __init__.py fie that ties them all together.
A class is a piece of code that describes a “data object.” An object could be a bad guy in a computer game, or it could be an entry in a contact management database. A single class can be used to create endless “instances” of the object.
Also read: How to use classes in Python
Many classes are kept in separate files, meaning they work just like a Python module. However, not all classes are modules, and likewise, classes can be included in-line in the main flow of your code.
How to make your own Python module
Want to know how to make and use a Python module? It’s extremely simple!
Simply create a new .py file in the same folder as your main Python code. Then “import” that file as you now know how to do, then access the functions from within that module.
So, if we make a new .py file called “useful tools,” this might look like so:
def say_hi():
print("Hello World!")
From any other Python file, we can then use:
import useful_tools
useful_tools.say_hi()
And that is how you create and use a Python module!
With this useful skill under your belt, you might now be ready to take on more Python challenges. We’ve compiled a list of the best online Python courses to take you to that next level. Check that out here.
For more developer news, features, and tutorials from Android Authority, don’t miss signing up for the monthly newsletter below!