Affiliate links on Android Authority may earn us a commission. Learn more.
Kotlin tutorial for Android for beginners: Build a simple quiz
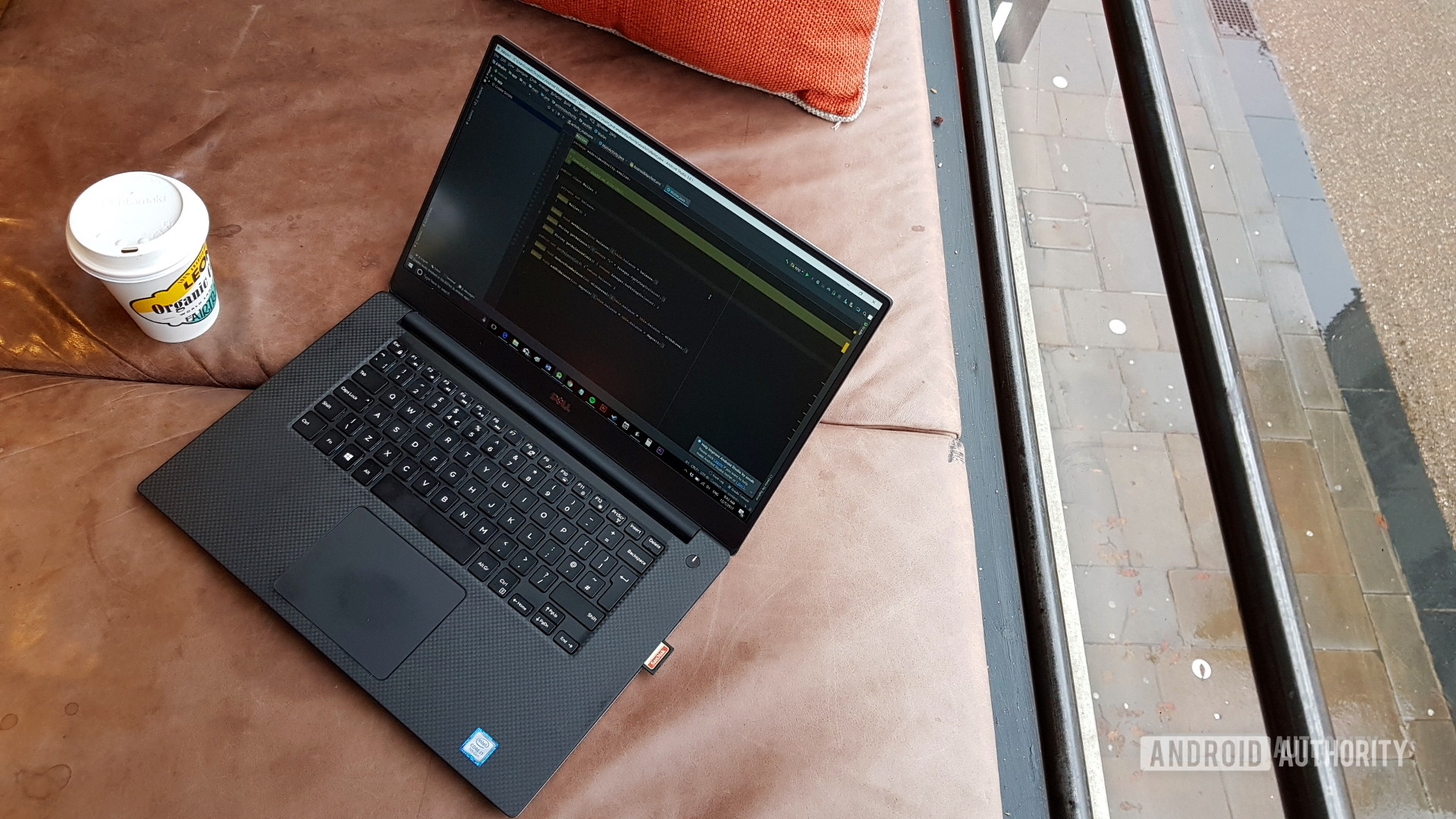
Kotlin is one of two official languages used in Android development and is Google’s preferred choice when it comes to the platform. This is good news for wannabe developers, owing to its significantly shallower learning curve and beginner-friendly nature. Finding your way through a Kotlin tutorial is easier than you may think, meaning anyone can now start building apps for the Android platform!
However, Kotlin was also slightly later to the party than its brother Java – only being an official language since 2017. It’s also less commonly used outside of Android, so many established developers still aren’t familiar with it.
In this Kotlin tutorial, we’re going to walk through the process of building a simple Kotlin app – a quiz – that will serve as a jumping-off point to help you learn Kotlin at large. Let’s go!
Also read: A guide to Android app development for complete beginners in 5 easy steps
Starting your first Kotlin Android project
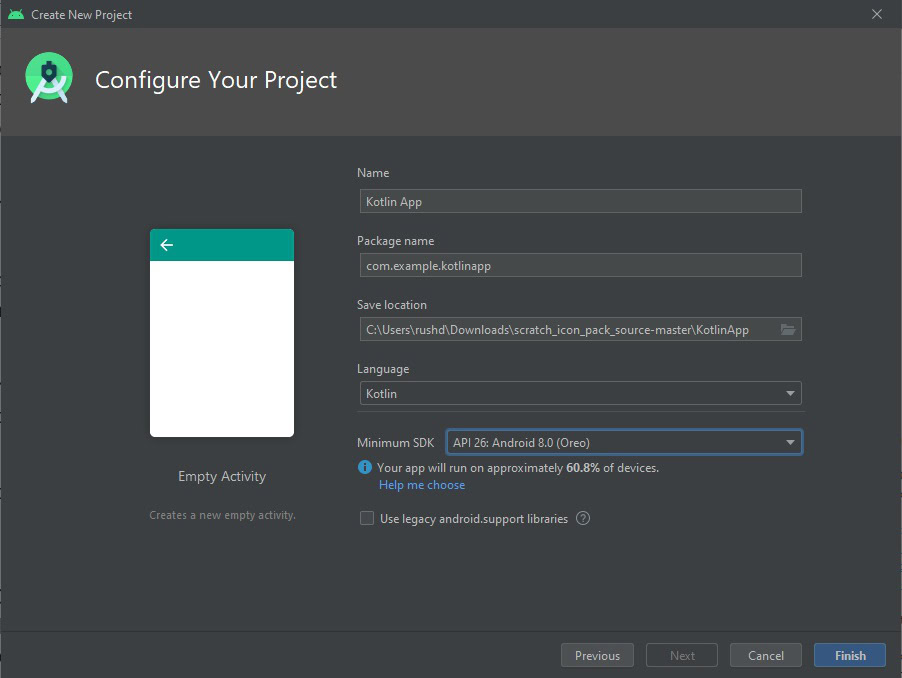
To learn Kotlin for Android development, you’ll first need to download Android Studio and set everything up.
See also: How to install Android Studio and start your very first project
Once that’s done, start a new project and make sure you’ve selected Kotlin as the language from the dropdown menu. Now pick an “Empty Activity” as your starting template.
You’ll be greeted with some code that looks like this:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
This boilerplate code is found in your MainActivity.kt file, and is the first thing that will run when you launch the app. All this code does, is to tell Android to display the layout file: activity_main.xml. Android Studio has handily opened that for us too, in another tab along the top of the main window. You can hit this tab at any time to see a visual designer where you can drag and drop elements like buttons and text views. If all you see is more code when you open this file, you’ll need to switch to the “Design” view by hitting the button in the top right.
In Android development, you will need to work with not only Kotlin/Java, but also XML. XML stands for “Extensible Markup Language” and is a “markup language.” This means that it doesn’t dictate logic or dynamic actions, but rather simply defines where things go on a page.
When you build Android apps, you’ll need to create layouts using XML and the visual designer, and then define how those elements work in the corresponding Kotlin or Java code.
See also: An introduction to XML for new Android developers – the powerful markup language
For now, just follow along with the instructions and hopefully it will all become clear!
Kotlin: getting started and creating the layout
Before we start to learn Kotlin programming, we’re first going to make some changes to the XML file so that we can create the design we want.
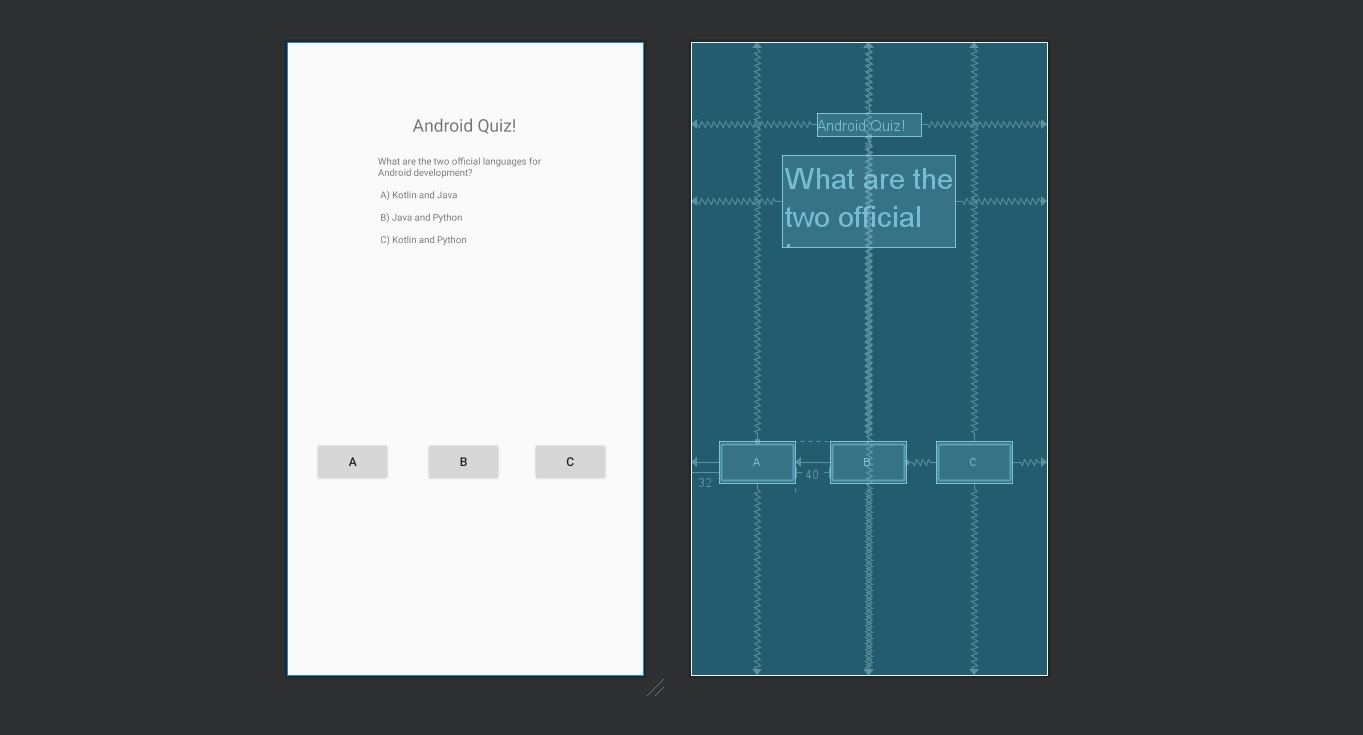
To make life easier, you can simply click on the “Code” view and then paste the following to overwrite what’s there already:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Android Quiz!"
android:textSize="20sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.117" />
<TextView
android:id="@+id/textView"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="What are the two official languages for Android development? \n\n A) Kotlin and Java \n\n B) Java and Python \n\n C) Kotlin and Python"
android:textSize="11sp"
app:layout_constraintBottom_toTopOf="@+id/button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2"
app:layout_constraintVertical_bias="0.083" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:text="A"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.674" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="40dp"
android:text="B"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.674" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="C"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.472"
app:layout_constraintStart_toEndOf="@+id/button2"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.674" />
</androidx.constraintlayout.widget.ConstraintLayout>
This, in turn, means we can focus on the Kotlin programming, and not have to worry about what our app looks like. Much.
Making your buttons do things!
Now we have our layout, the good news is that extremely easy to refer to those elements and change them within the Kotlin programming.
To do this, we need to refer to the “IDs” that we gave our views. A “view” is the technical name for the widgets that make up our layout, such as buttons and text labels. If you want to learn more about the Android
You’ll see we already did this in the XML:
android:id="@+id/button"
In this case, the button’s “ID” is “button.” Note that this is case-sensitive. The word “Button” with a capital “B” actually refers to the broader concept of all buttons in Kotlin.
We can, therefore, refer to the button in our code. Were we to write button.setText(“Right Answer!”) then it would change the text on the first button to say “Right Answer!”
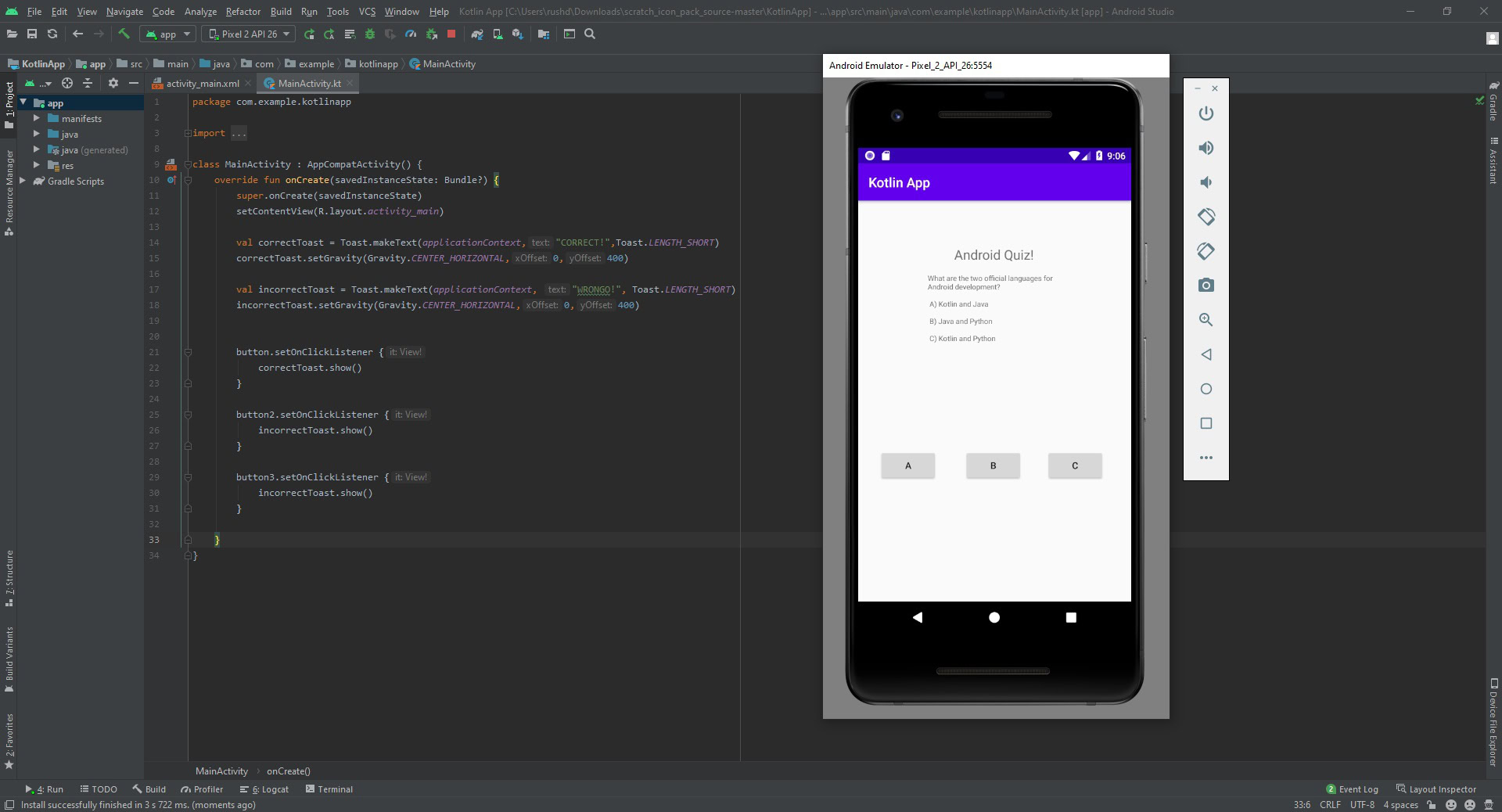
But we don’t want to do that. Instead, we’re going to make it so that clicking the first button says “CORRECT!” and lets our player know that they clicked the right solution.
To do this, we’re going to show a message on the screen that is known as a “toast message.” This is a little floating text bubble that fades away after a couple of seconds.
Don’t worry about the line of text that builds and displays the toast message, this is something you will rarely need and that is part of Android rather than Kotlin.
We’re going to make it so that these messages show when we click on one of these buttons:
button.setOnClickListener {
Toast.makeText(applicationContext,"CORRECT!",Toast.LENGTH_SHORT).show()
}
button2.setOnClickListener {
Toast.makeText(applicationContext, "WRONGO!", Toast.LENGTH_SHORT).show()
}
button3.setOnClickListener {
Toast.makeText(applicationContext, "WRONGO!", Toast.LENGTH_SHORT).show()
}
}
Place this code inside the onCreate function (that’s all the code in the curly brackets following the word onCreate). In Kotlin, like Java, code can be “blocked” together by placing it inside curly brackets. This grouping is useful if we want a specific set of code to run outside of a linear path for example. We’ll learn what a “function” is in a moment.
Now click the green play button in the Android Studio IDE, making sure you have a virtual device set-up or an Android device plugged in. You’ll now see your game appear on the screen, and you’ll be able to select an answer. Lo and behold, clicking on “A” should display the correct message and the other two should display the incorrect message.
Kotlin tutorial – Using functions in Kotlin
You might think you’ve now made your first Kotlin android app, but in truth there’s actually very little Kotlin programming here. Rather, we are relying mostly on the XML for the appearance, and the Android SDK for the performance. “setText” for example is not an example of Kotlin, but rather it is part of an Android class (TextView). That is to say that Kotlin itself doesn’t support these types of buttons and text labels but is simply being used for the structure in this case.
And so far, this isn’t much of a program. There’s only one question, and the answer is mapped to a single button. If we want to make this into a dynamic program that can show multiple questions and answers, then we need to add some logic. This is where the Kotlin code comes in.
First, let’s structure this a bit better. In programming, we never want to type something out more times than necessary, so we’re going to put our toast messages into a function. A function is a chunk of code that can be referenced at any point throughout the rest of the program.
Also read: Kotlin vs Java for Android: key differences
To define a function, we need to give it a name that will describe what it does. We then prefix this with the word “fun” and follow it with curly brackets. Make sure you do this outside of the onCreate function but inside the MainActivity class (while it’s a massive oversimplification, this is effectively the file name).
Inside here, we’re going to show our toasts. To see how this works, let’s place just one toast message inside our function and then call it from button one:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
button.setOnClickListener {
showToast()
}
}
fun showToast() {
Toast.makeText(applicationContext,"CORRECT!",Toast.LENGTH_SHORT).show()
}
}
Run this and the code will behave precisely as it did before. The only difference is that the code is better organized.
See how we only now have to write the function name with two brackets in order to execute our line of code at any point? If showToast() did something more complex, this could save us countless hours; especially if we ever needed to change the way the toast message displayed!
What we really want to do though, is to change the answer that shows depending on the button that gets clicked. We could do this with a second function, but better yet would be to place both messages inside a single function.
To this end, we’re going to use two new concepts from Kotlin programming.
Kotlin arguments, variables, and conditional statements
Now you know how to use functions to group sections of code in Android, the next part of this Kotlin tutorial is where the real magic is going to happen. Now you’re going to learn three invaluable skills for coding in Kotlin and any other language!
First, a variable is a “container” for data. To put this another way, a variable lets you use a word to represent another word, a number, or even a list of items. You will likely remember variables from algebra lessons:
a + 2 = 3, find a!
Here, a is obviously used to represent “1.” We use variables similarly in programming so that we can change the way our code behaves depending on user interactions or other inputs.
What we can also do with variables though, is to pass them between functions. When we do this, we call it an “argument.” This essentially lets us pass an input into our function, to change the output.
Also read: The best Android developer tools for getting started
To define the arguments that a function will accept, we simply have to place them inside the curly brackets.
We’re using a type of variable called an integer, which is referred to as “Int” in Kotlin. Integers, you may also remember from math, are whole numbers with no decimal places.
We, therefore, need to update our function to look like so:
fun showToast(answer: Int) { }
Here, the name of the function is “answer” and it is of the type integer. Now, when we subsequently call our function, we need to add a whole number inside the curly brackets:
showToast(1)
You can pass as many arguments into a function as you like, so long as you define them each and separate them with commas.
In this case, we are going to assign each button to a number. A = 1, B = 2, and C = 3. Now the showToast function knows which button the user clicked!
button.setOnClickListener {
showToast(1)
}
button2.setOnClickListener {
showToast(2)
}
button3.setOnClickListener {
showToast(3)
}
Now we just need to change the toast message that shows depending on which answer we read!
To do this, we use something called a “conditional statement.” That means that a line of code is only going to play out under certain conditions. In this case, we will only show the correct message if the user clicked the right answer. We achieve this with the following code:
if (answer==1) {
Toast.makeText(applicationContext, "CORRECT!", Toast.LENGTH_SHORT).show()
}
This is an “if” statement, and it will only show the code inside the curly brackets if the statement in the regular brackets is true. In this case, if the variable “answer” holds the value “1,” then we can run the code!
What do we do if the answer is 2 or 3? Well, we could always use two more conditional statements! But a quicker solution would be to use the “else” statement. This does exactly what you would expect it to do when it follows on from an “if” statement:
fun showToast(answer: Int) {
if (answer==1) {
Toast.makeText(applicationContext, "CORRECT!", Toast.LENGTH_SHORT).show()
} else {
Toast.makeText(applicationContext, "WRONGO!", Toast.LENGTH_SHORT).show()
}
}
Making the game fun
If you didn’t follow all of that the first time, I recommend re-reading through it a few times. This is the most important part of the Kotlin tutorial, and with just those few skills, you’ll be able to handle an awful lot of code!
Next, we’re going to flex our new coding chops to make this into an actual game.
First, we’re going to create three more variables. These will be global variables created outside of any functions and therefore accessible to all functions.
Note that in Kotlin, you don’t need to assign a variable to a type. In languages like Java, you need to state right away whether your variable is an integer (whole number), string (word), number with a decimal (float), etc. In Kotlin, we can just write “var” and let Kotlin figure it out!
Before the onCreate() function, add these three lines:
var questionNo = 0
var questions = listOf("What are the two official languages for Android development? \n\n A) Kotlin and Java \n\n B) Java and Python \n\n C) Kotlin and Python", "How do you define a function in Kotlin? \n\n A) void \n\n B) var \n\n C) function", "What is a variable used for? \n\n A) To contain data \n\n B) To insert a random value \n\n C) Don't know", "What does SDK stand for in Android SDK? \n\n A) Software Development Kit \n\n B) Software Development Kotlin \n\n C) Something Don't Know")
var rightAnswers = listOf(1, 2, 1, 1)
The first variable is another integer and will be used to keep track of the question number we’re on. The second is a little more complicated. This is a “list” meaning that it is a variable that can contain multiple values – in this case multiple strings. Each of our strings is separated by a comma and will be given an index to reference later (note that the first item on the list is given the index: 0). Note that the \n symbol is recognized by Kotlin (and most languages) as meaning “new line” and won’t actually show up in our output.
(This does look a little ugly, and if you were building an actual app, you might choose to store these values in a separate XML file instead.)
The final line creates another list, this time filled with integers. These are the correct answers for each of our questions!
Also read: How to make an app with no programming experience: what are your options?
Next, we’re creating a new function called updateQuestion. All we’re going to do in here is to change our question depending on the question number. We do this like so:
fun updateQuestion() {
questionNo = questionNo + 1
textView.setText(questions.get(questionNo))
}
Because we only call this function when someone gets an answer right, we can safely increase the value of our questionNo variable at the start of the function. Next, we are going to set the question that displays by updating the textView.
When we use “get” in this way with a list, we only need to insert the index in the brackets in order to retrieve the value that is placed there. In this way, we can grab the next question along by incrementally increasing that value.
Finally, we change our “right answer” condition to whatever the right entry in our list of right answers is. The resulting code should look like this:
class MainActivity : AppCompatActivity() {
var questionNo = 0
var questions = listOf("What are the two official languages for Android development? \n\n A) Kotlin and Java \n\n B) Java and Python \n\n C) Kotlin and Python", "How do you define a function in Kotlin? \n\n A) void \n\n B) var \n\n C) function", "What is a variable used for? \n\n A) To contain data \n\n B) To insert a random value \n\n C) Don't know", "What does SDK stand for in Android SDK? \n\n A) Software Development Kit \n\n B) Software Development Kotlin \n\n C) Something Don't Know")
var rightAnswers = listOf(1, 2, 1, 1)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
button.setOnClickListener {
showToast(1)
}
button2.setOnClickListener {
showToast(2)
}
button3.setOnClickListener {
showToast(3)
}
}
fun showToast(answer: Int) {
if (answer==rightAnswers.get(questionNo)) {
Toast.makeText(applicationContext, "CORRECT!", Toast.LENGTH_SHORT).show()
updateQuestion()
} else {
Toast.makeText(applicationContext, "WRONGO!", Toast.LENGTH_SHORT).show()
}
}
fun updateQuestion() {
questionNo = questionNo + 1
textView.setText(questions.get(questionNo))
}
}
Continuing the Kotlin tutorial
Run this code and you should now find that the question updates each time you get the answer right! This is a full, working game, and you could create many more projects like it with the skills you learned here.
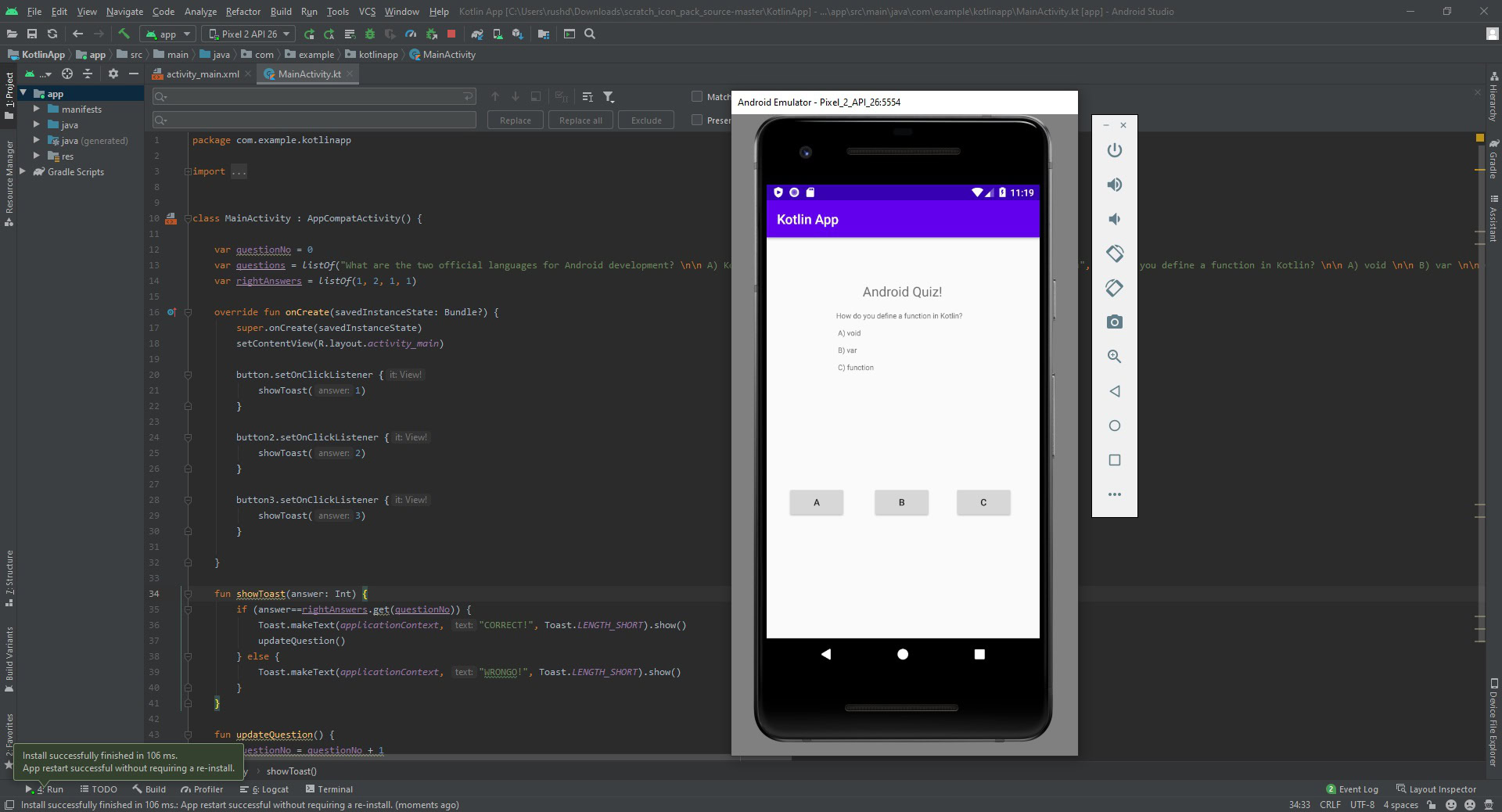
The only slight issue with all this is that right now, the game will crash out as soon as you run out of questions!
But rather than hand you the answer on a silver plate, I’m going to invite you to go off and find the answer yourself. That is how you will truly learn Kotlin, after all: by building your own projects and learning how to do each new thing as you need it.
Find another Kotlin tutorial, keep learning, and you’ll be making awesome Android apps in no time!
For more developer news, features, and tutorials from Android Authority, don’t miss signing up for the monthly newsletter below!