Affiliate links on Android Authority may earn us a commission. Learn more.
Java tutorial for beginners part 3 - Importing classes, try blocks and more!
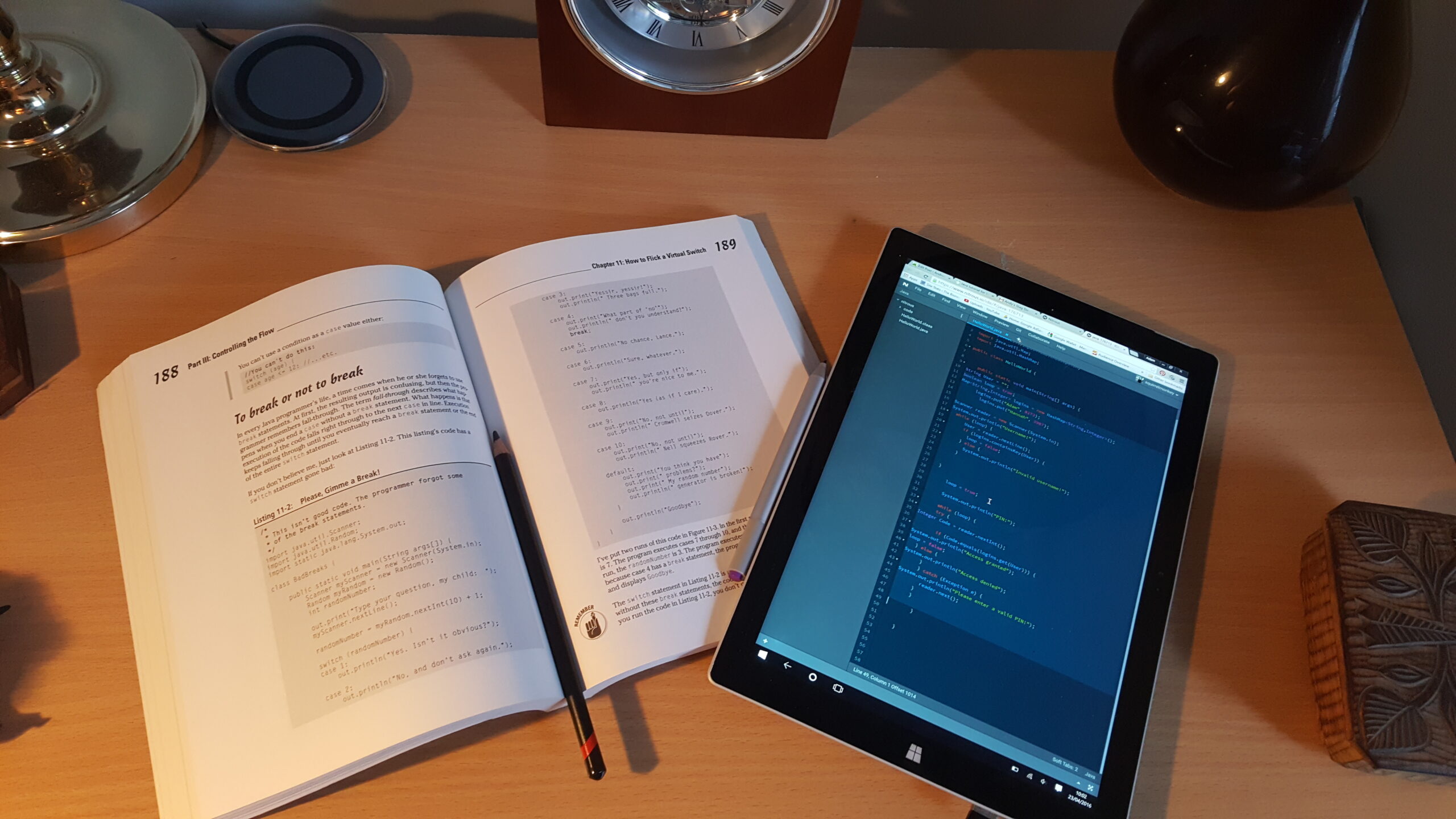
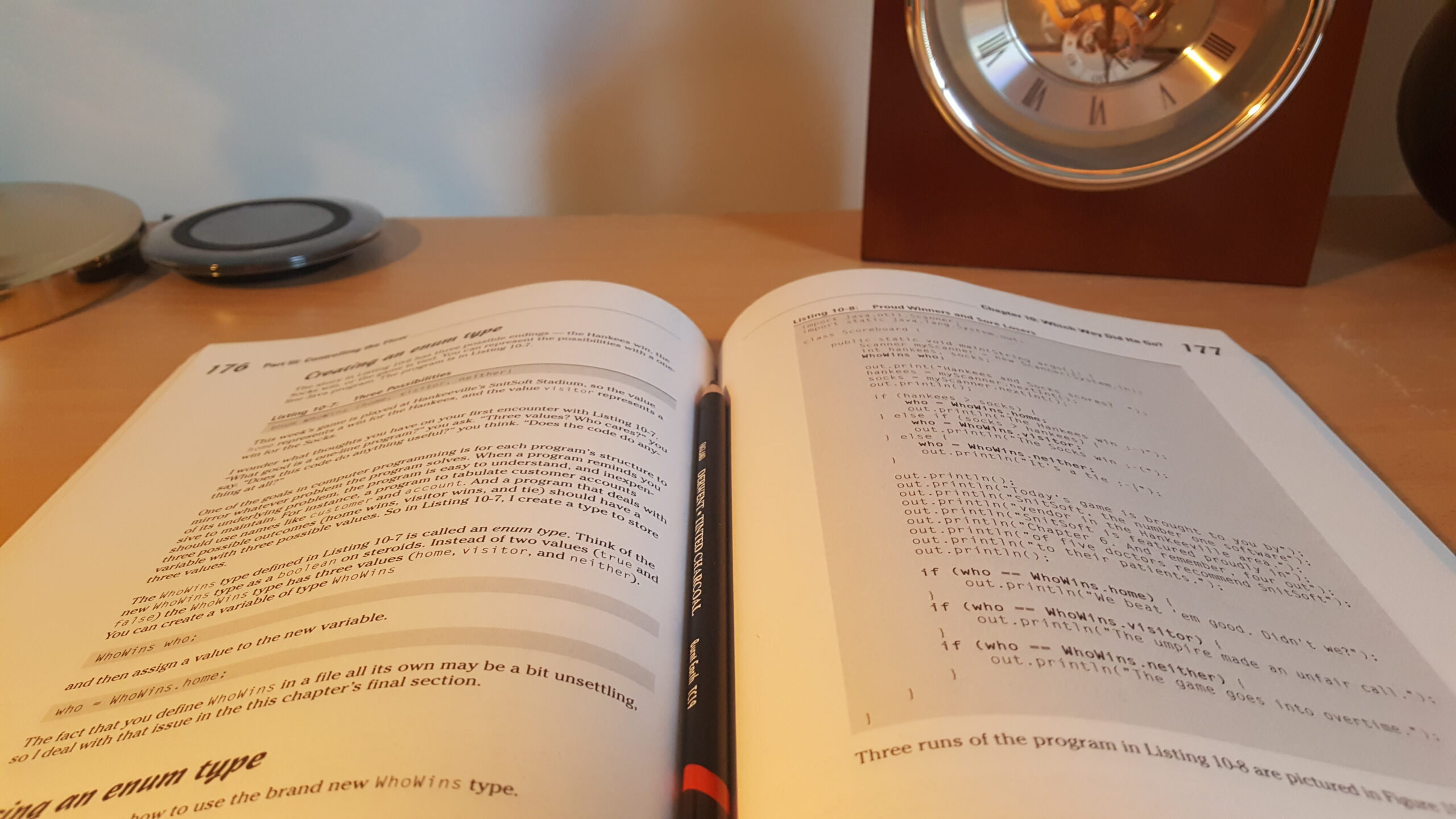
In part 1 of this series, Gary went over the basics of Java and introduced us to variables, objects and loops. In part 2 we took a look at using methods, arrays and conditional statements. And with that basic foundation you should now be able to create some fairly useful programs and hopefully start building the confidence you need to jump into Android development.
In this 3rd installment of the series, I’ll be showing you how to import classes in order to further extend the capabilities of Java. I’ll also be looking a little more at what object oriented programming means and addressing some more advanced tricks such as using maps and try blocks. By the end we’ll build a simple ‘user login’ screen and hopefully you’ll have a good grasp of how to take the next step and start building apps using the Android SDK. You’re nearly there!
Modular programming
Part one showed us that a class ‘defines an object’ and that an object is ‘a self-contained item that interacts with other objects’. An object can be a piece of data or it could be a UI element such as a button. A method meanwhile is a small, separate piece of code that performs a specific function and often gives us some kind of output, usually from a class.
Java is an ‘object oriented’ programming language. That means that it’s built from many objects that interact with each other, rather than being a long list of instructions. It also means that Java can be ‘modular’, which is one of the big advantages of this approach to programming. It can be quite tricky to get your head around but once you do, this type of programming is very logical and very satisfying!
It can be quite tricky to get your head around but once you do, this type of programming is very logical and very satisfying!
Essentially, modular programming allows you to lift whole pieces of code (methods and objects) directly from previous projects and paste them into your new program; thereby gaining access to that functionality. This is ideal for large teams working on big projects as it allows them all to work on separate aspects of a program and then assemble them together into one large piece of software. Using private members (which you should remember from part one) also helps with this, as it means your methods won’t be dependent on outside code and will be self-contained. It also helps us to more easily track down bugs as we know precisely where in the code each variable is being edited. If something goes wrong, we know where to find the issue!
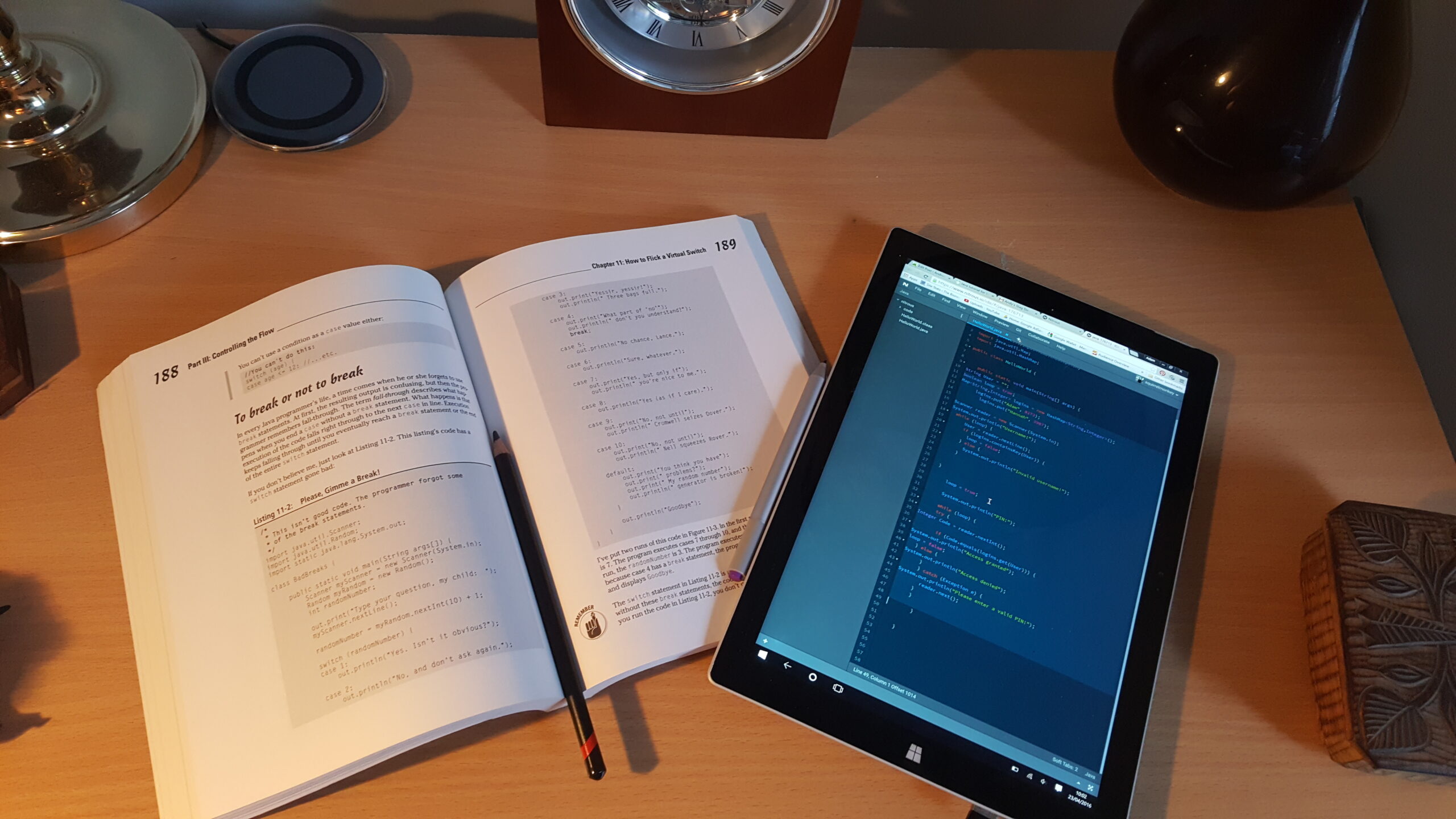
This also makes life easier for you in the future, as it means you won’t need to keep repeating yourself and writing the same routines over and over again – you can just paste methods and objects from your previous work.
Importing classes
Java itself also takes full advantage of this modular approach by allowing you to import additional classes and reference additional methods when you want extra functionality.
Let’s refer back to a piece of code we used earlier:
String User = "Adam";
if (User == "Adam" || User == "Hannah") {
System.out.println("Hello " + User);
}
Here, the code checks if the username is ‘Adam’ or ‘Hannah’ and then says hello if the user is authorized. To do this, we are actually referencing another method when we say System.out.println(). This is a ‘built-in’ method that is part of the compiler package. Although you can’t see it, there is a method performing this functionality behind the scenes. The methods you write yourself are called ‘user-defined’ methods.
But this code would make a lot more sense if the user was actually given the opportunity to enter their own name! So how do we go about doing this?
This is where it becomes helpful to start importing additional classes. Specifically, we want the Scanner class which will give us the ability to read input from the user. To do this, we just add this line at the top of the code:
import java.util.Scanner;
Now we can reference ‘Scanner’ later on, as though it were a class in our own program, like so:
Scanner reader = new Scanner(System.in);
System.out.println("Username: ");
String User = reader.nextLine();
System.out.println("Hello " + User);
if (User.equals("Adam") || User.equals("Hannah")) {
System.out.println("Access Granted");
}
Note that we’re now using ‘equals’ instead of ‘==’. That’s because ‘==’ actually checks the references to the object rather than the content itself. You don’t need to worry too much about that right now, just focus on how we imported an additional class and then used it in our code.
And guess where else you can get a ton of classes with useful functions? That’s right… from the Android SDK!
There are many more classes and packages (packages are essentially just collections of classes that are grouped together) and these allow you to do all sorts of things like use graphics or play sounds. And guess where else you can get a ton of classes with useful functions? That’s right: from the Android SDK! And now you’re starting to see how this whole thing relates back to Android development.
Basically, when you use Java to build Android apps, you are using the basics of Java that we’ve covered in these posts and then adding extra functionality from the Android SDK in order to use things like widgets or access aspects of the device. You never get to see all the code provided in the classes that Google built, but you can interact with it by using the public methods. When you say something like inflater.inflate() in an Android program, you are saying that you want to call the inflate method from the inflater class. This method of interacting with code other than your own is called an API – an Application Programming Interface.
Try Blocks
Using the Scanner class we can also check for passwords. Instead of a username, let’s use a PIN. We’ll use the PIN for my debit card, which is 8273… Or is it?
Anyway, to check this, we’ll use the same thing but look for an integer instead of a string. Now we can test to see if the user has entered the correct code or not and if not, we’ll tell them about it!
Scanner reader = new Scanner(System.in);
System.out.println("PIN: ");
Integer Code = reader.nextInt();
if (Code == 8273) {
System.out.println("Access Granted");
} else {
System.out.println("Access Denied");
}
Nice one! But now what happens if our user decides to be contrary and says ‘hello’ instead of entering a PIN?
Uho… now we have an error message coming up! Not terribly professional…
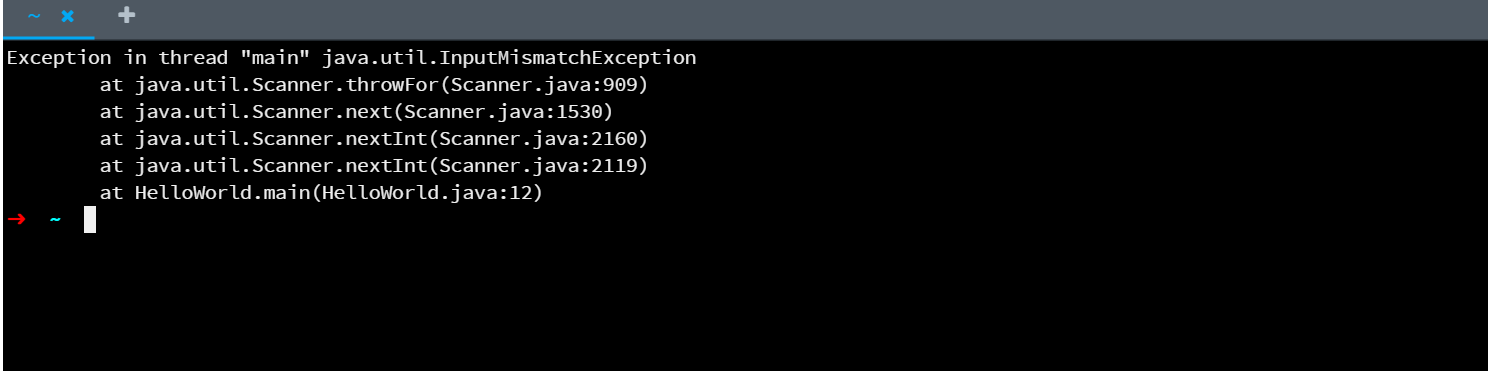
So what we need to do is use a try block. This basically means that we’re going to try to run some code and if it doesn’t work, we’re going to catch the ‘exception’ (the error) and prevent it from stopping the program:
Scanner reader = new Scanner(System.in);
System.out.println("PIN: ");
try {
Integer Code = reader.nextInt();
if (Code == 8273) {
System.out.println("Access granted");
} else {
System.out.println("Access denied");
}
} catch (Exception e) {
System.out.println("Please enter a valid PIN!");
}
Much better!

We can also report back to ourselves on what went wrong this way by saying:
System.out.println(e)
This makes debugging a lot easier because we can then look up exceptions!
Creating your first little program!
At this point, we nearly have enough code to create a fun little text-based game! But we’re actually not going to do that… Instead we’re going to make a not-fun log-in system. But I’ll show you how you could turn that into a game if you wanted to. And you should find the log-in system is surprisingly rewarding to make…
In doing this, we’ll learn a couple more concepts, including how to use maps and Booleans. You may recall earlier that I mentioned a Boolean was another type of variable that could only equal true or false. Now I’m going to show you how to implement that while inserting our password test into a while loop:
boolean loop = true;
Scanner reader = new Scanner(System.in);
System.out.println("PIN: ");
while (loop) {
try {
Integer Code = reader.nextInt();
if (Code == 8273) {
System.out.println("Access granted");
loop = false;
} else {
System.out.println("Access denied");
}
} catch (Exception e) {
System.out.println("Please enter a valid PIN!");
reader.next();
}
}
So what’s happening here is that the loop keeps going round and round until we enter the correct password and the loop becomes ‘false’. We’ve added another line reader.next() to prevent the error message from showing in an infinite loop.
Next we’re going to use a map… A map is very similar to an array, except it allows us to use ‘keys’ as references for where we stored data. So instead of a shopping list, we might use this to create, oh I don’t know, a database of usernames and their passwords?
To do this, we first need to import maps and hash maps:
import java.util.Map;
import java.util.HashMap;
Map is considered an ‘interface’, while HashMap is a class that implements that interface. This type of map allows null keys and values and has no order. But you don’t really need to know about that, you just need to know how to use it! So next, we create our map in a similar way to the array and populate it using get and put methods:
Map<String,Integer> logins = new HashMap<String,Integer>();
logins.put("Adam", 8273);
logins.put("Hannah", 3987);
Notice that we’re indicating that we want to use Strings for our ‘keys’ (the location) and Integers for our mapped values. That means we can look up an integer with a string. So the following returns the PIN ‘8273’ and we can compare this with an input using the equals method.
logins.get("Adam")
There are a few more fancy methods we can use with the map interface. For now though, the only other one you’ll need is containsKey, which returns ‘true’ if the map contains that value (in our case the username). Also, for your own use in future, you might find remove useful as well!
With all that in mind, we can now create our very simple username and password login screen…
String User = "";
boolean loop = true;
Map<String,Integer> logins = new HashMap<String,Integer>();
logins.put("Adam", 8273);
logins.put("Hannah", 3987);
Scanner reader = new Scanner(System.in);
System.out.println("Username:");
while (loop) {
User = reader.nextLine();
if (logins.containsKey(User)) {
loop = false;
} else {
System.out.println("Invalid username!");
}
}
loop = true;
System.out.println("PIN:");
while (loop) {
try {
Integer Code = reader.nextInt();
if (Code.equals(logins.get(User))) {
System.out.println("Access granted");
loop = false;
} else {
System.out.println("Access denied");
}
} catch (Exception e) {
System.out.println("Please enter a valid PIN!");
reader.next();
}
}
So what’s happening here is that we’re asking for the user name and then using this as the ‘key’ to look up the correct password at the next step. If the string entered for the username matches the key of the integer PIN, then the user is granted access!
This could very easily be made into a game like a quiz or test of some sort. And it actually wouldn’t take that much work to get it running on Android – throw in a little UI and you have your first app!
There’s scope for a lot more Java lessons in the future, but for now this should be a great foundation to build off of. And remember there are lots of additional resources listed in part one that you can use for further reading.