Affiliate links on Android Authority may earn us a commission. Learn more.
How to use classes in Java
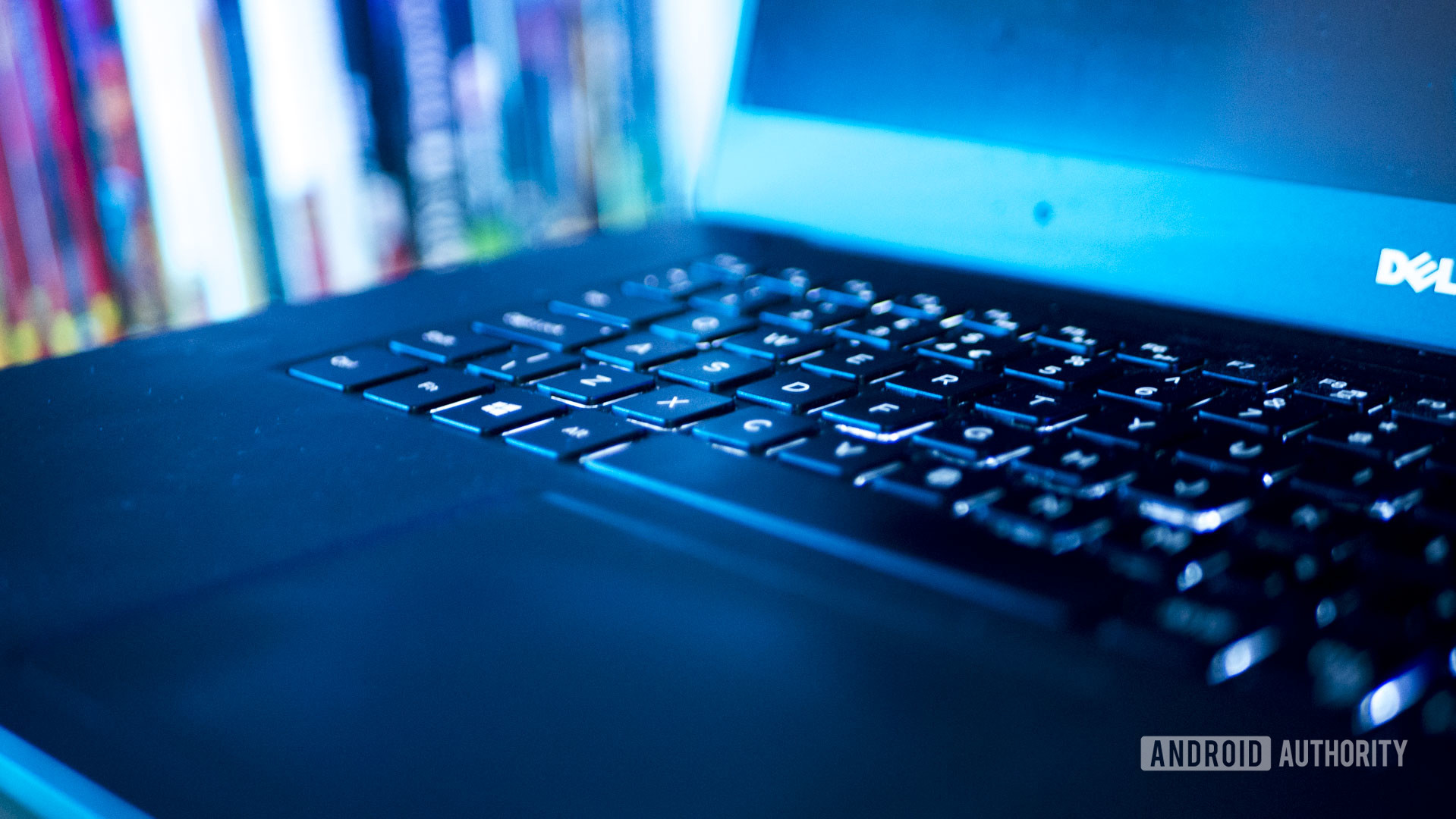
One of the features that make Java so powerful, is its object-oriented structure. This means that Java uses classes and objects to create more scalable, modular, and organized code. This can be a complex concept to wrap your head around as a new developer, though. So in this post, we’re going to examine how to use classes in Java.
Also read: Java beginner course – A free and comprehensive guide to the basics of Java
What are classes in Java?
If you’re not familiar with the concept of object-oriented programming, this is the best place to start. If you already know what a class is and just want to learn Java syntax, you can skip this section.
Also read: What is object-oriented programming?
So, what is a class? A class is a piece of code that builds an object. An object is a conceptual “thing” that exists purely in terms of the data that describes it. Objects have properties (called variables) and they have functions (called methods). This is just the same as an object in the real world: a dog, for example, has properties (brown fur, four legs, 30cm tall) and functions (bark, sleep, rollover, poop).
The only difference between an object in the real world and an object in code is that the code object does need a physical body.
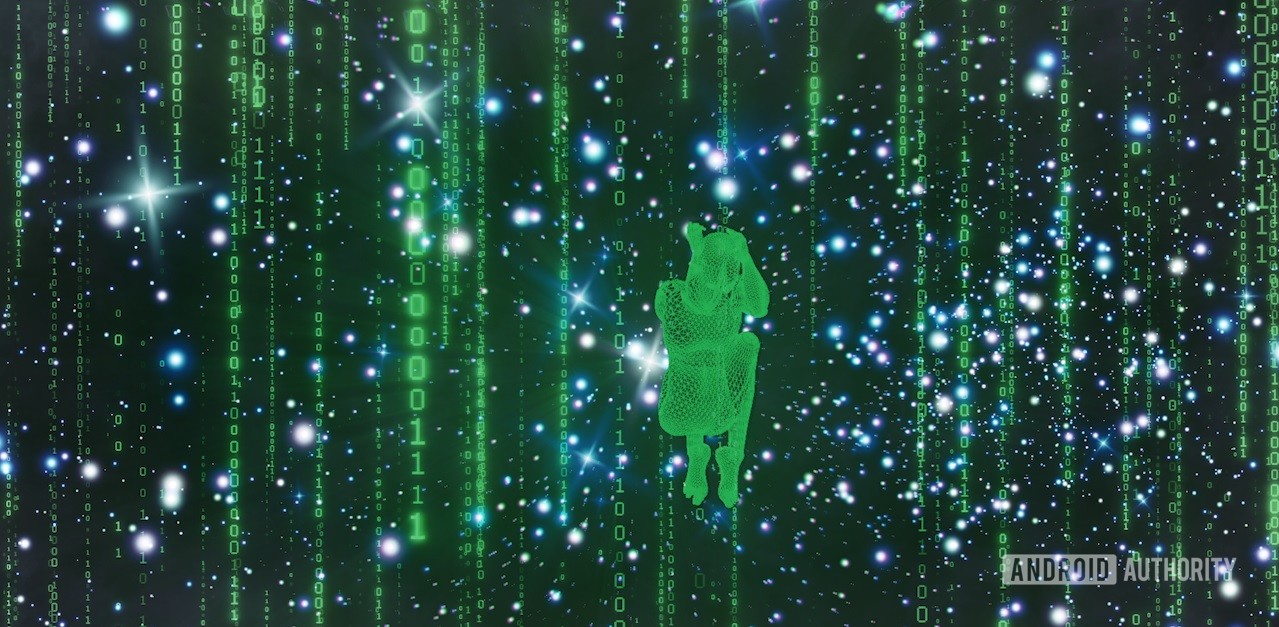
For example, a bad guy in a computer game might well be an object. This bad guy has variables (movement speed, health, sprites) and it has methods (shoot, move left, move right, explode). That bad guy “exists” in the code whether or not we can see it on the screen. Thus it is a data object.
Instances of objects
The class is the piece of code that creates the data object. Our “BadGuy” class, therefore, is used to build bad guys. But this is the next important distinction to make: the BadGuy code doesn’t control a single bad guy. Rather, it tells the computer to allocate data that will represent a bad guy. And we can run that code multiple times to create multiple bad guys.
There is one Goomba class that can create as many Goombas as are needed at any given time.
So in Super Mario, it’s unlikely that there would be a separate piece of code for every single Goomba in the game. Instead, there is probably one Goomba class that can create as many Goombas as are needed at any given time. This makes the code more efficient.
The metaphor that is often used here, is to say that classes in Java are the “blueprints” whereas the objects are single examples of that product.
And we call each individual product created by a given class an instance of that object.
How to use classes in Java
So with all that in mind, how do you go about using classes in Java?
Here is a very simple “BadGuy” class that assigns values to variables like “health” and gives it the ability to shoot at us:
class BadGuy{
static int speed = 3;
static int health = 5;
static int xPosition = 20;
static int yPosition = 100;
public static void shoot() {
System.out.println("Pew pew!");
}
}
Now we can call upon our BadGuy class from within our “Main” code (which is a class in itself) simply by saying:
class Main {
public static void main(String[] args) {
BadGuy.shoot();
}
}
So, what’s going on here? First, we create our class by writing “class” followed by the name of our class. In Java, it is considered best practice to camel case when choosing class names with the first word capitalized. Camel case simply means that you remove all spaces and use capitalization for each word instead. Hence: “BadGuy”, not “Bad_Guy” or “badguy.” This is optional but it contributes to readable code, especially if anyone else is going to be working on it.
We then create global static variables, followed by a public static method called “shoot” that will draw “Pew pew!” onto the screen.
The word “public” means that a method can be accessed from outside of a class. That means we can call that method from our Main class but we need to tell Java where to look. That’s why we say “BadGuy.shoot();”.
Notice that a lot of the statements in Java use periods in this way too (like System.out.println). This is because classes in Java are absolutely fundamental to the way it works. Many of the statements you use regularly are actually methods belonging to built-in classes!
Creating instances of objects
The reason we use the keyword “static” when referring to variables and methods, is because we want don’t want to tie our class to any specific instance of the object. Right now, our BadGuy class can’t make multiple bad guys each with different values for their health and speed.
To change this, we need to remove the static keyword, and we need to build one or more instances of the object:
class Main {
public static void main(String[] args) {
BadGuy badGuy1 = new BadGuy();
BadGuy badGuy2 = new BadGuy();
System.out.println(Integer.toString(badGuy1.health));
System.out.println(Integer.toString(badGuy2.health));
}
}
class BadGuy{
int speed = 3;
int health = 5;
int xPosition = 20;
int yPosition = 100;
public static void shoot() {
System.out.println("Pew pew!");
}
}
This code builds two instances of the BadGuy and can thus can show us health for each of them. We can then affect the health of just one of our bad guys like so:
class Main {
public static void main(String[] args) {
BadGuy badGuy1 = new BadGuy();
BadGuy badGuy2 = new BadGuy();
badGuy2.getHit();
System.out.println(Integer.toString(badGuy1.health));
System.out.println(Integer.toString(badGuy2.health));
}
}
class BadGuy{
int speed = 3;
int health = 5;
int xPosition = 20;
int yPosition = 100;
public static void shoot() {
System.out.println("Pew pew!");
}
public void getHit() {
health--;
}
}
Notice that the “getHit” method doesn’t include the keyword “static.” That means the method will act on the instance of the object so that only a particular bad guy’s health will be affected. We cannot, therefore, say: BadGuy.getHit(); without first building a new object.
Hopefully, this is now all starting to make sense and you can see how we might use classes in Java to create lots of instances of the same “object” that can all run around at once!
How to use constructors in Java
The last thing you need to learn about using classes in Java, is the role of the constructor.
A constructor is a special type of method in a class that is used to “build” that class by defining its values as soon as it is created. You might want this, for example, in order to create multiple bad guys each with different amounts of strength to start with. Alternatively, if you were building a client database, you might use this to build new entries where each one had a different name, phone number, etc.
The constructor method should have the same name as the class. We then need to pass arguments (variables) that we can use to define the values we want to set during initialization. We then need to make sure we add those variables in the brackets when we create new instances of the objects.
The final code looks like this:
class Main {
public static void main(String[] args) {
BadGuy badGuy1 = new BadGuy(3, 5, 20, 100);
BadGuy badGuy2 = new BadGuy(3, 27, 20, 100);
badGuy2.getHit();
System.out.println(Integer.toString(badGuy1.health));
System.out.println(Integer.toString(badGuy2.health));
}
}
class BadGuy{
public int speed;
public int health;
public int xPosition;
public int yPosition;
public BadGuy(int badGuySpeed, int badGuyHealth, int badGuyxPosition, int badGuyyPosition) {
speed = badGuySpeed;
health = badGuyHealth;
xPosition = badGuyxPosition;
yPosition = badGuyyPosition;
}
public static void shoot() {
System.out.println("Pew pew!");
}
public void getHit() {
health--;
}
}
Don’t know what arguments are? Then make sure to read our guide to calling methods in Java.
Closing comments
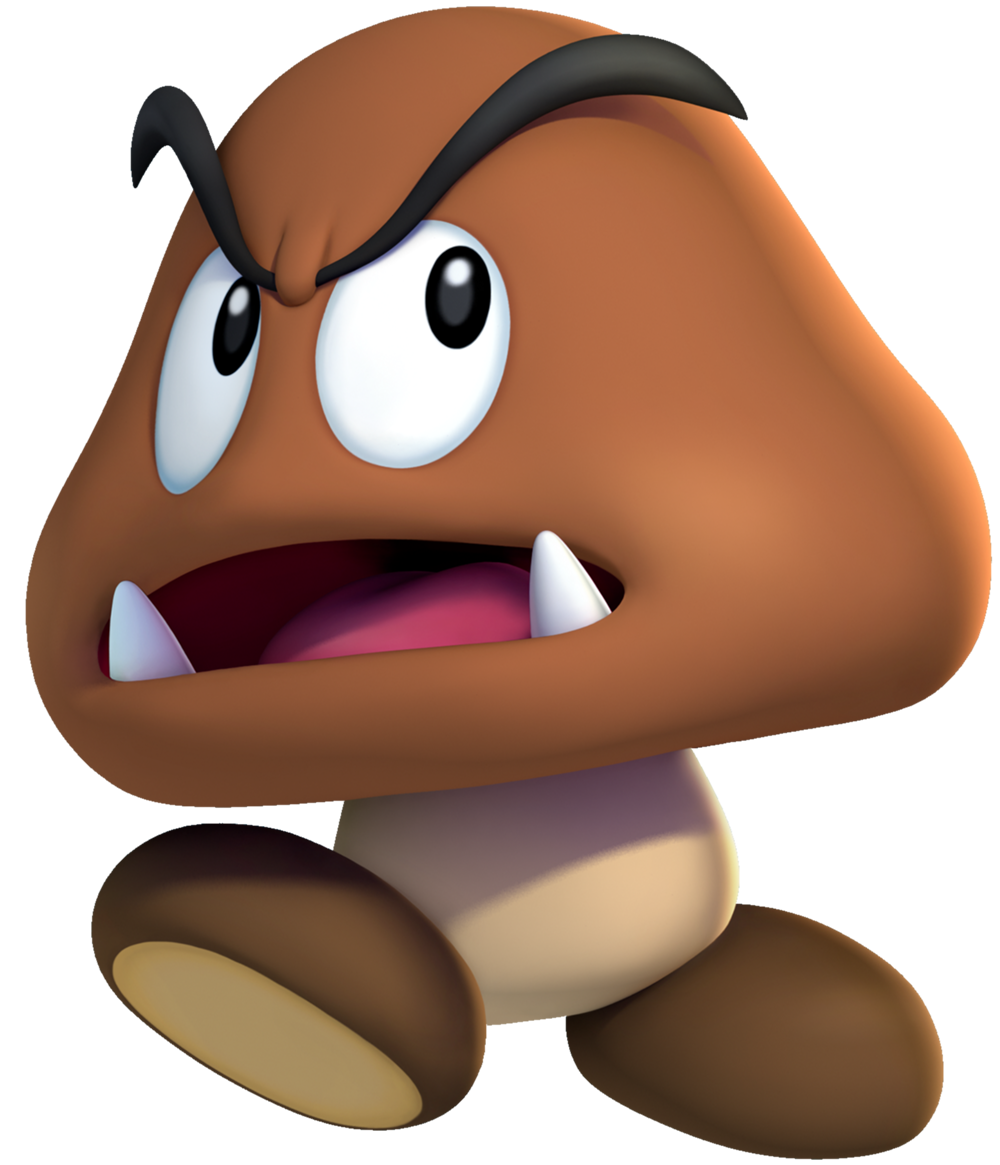
So, that’s everything you need to know about using classes in Java! That’s a high-level concept you’ve gotten to grips with, so pat yourself on the back! If you feel comfortable with classes and objects, then chances are you’re ready to take your learning further. Check out list of the best places to learn Java to gain a full Java education at heavily discounted rates!