Affiliate links on Android Authority may earn us a commission. Learn more.
How to create an array in Java
Published onFebruary 20, 2021
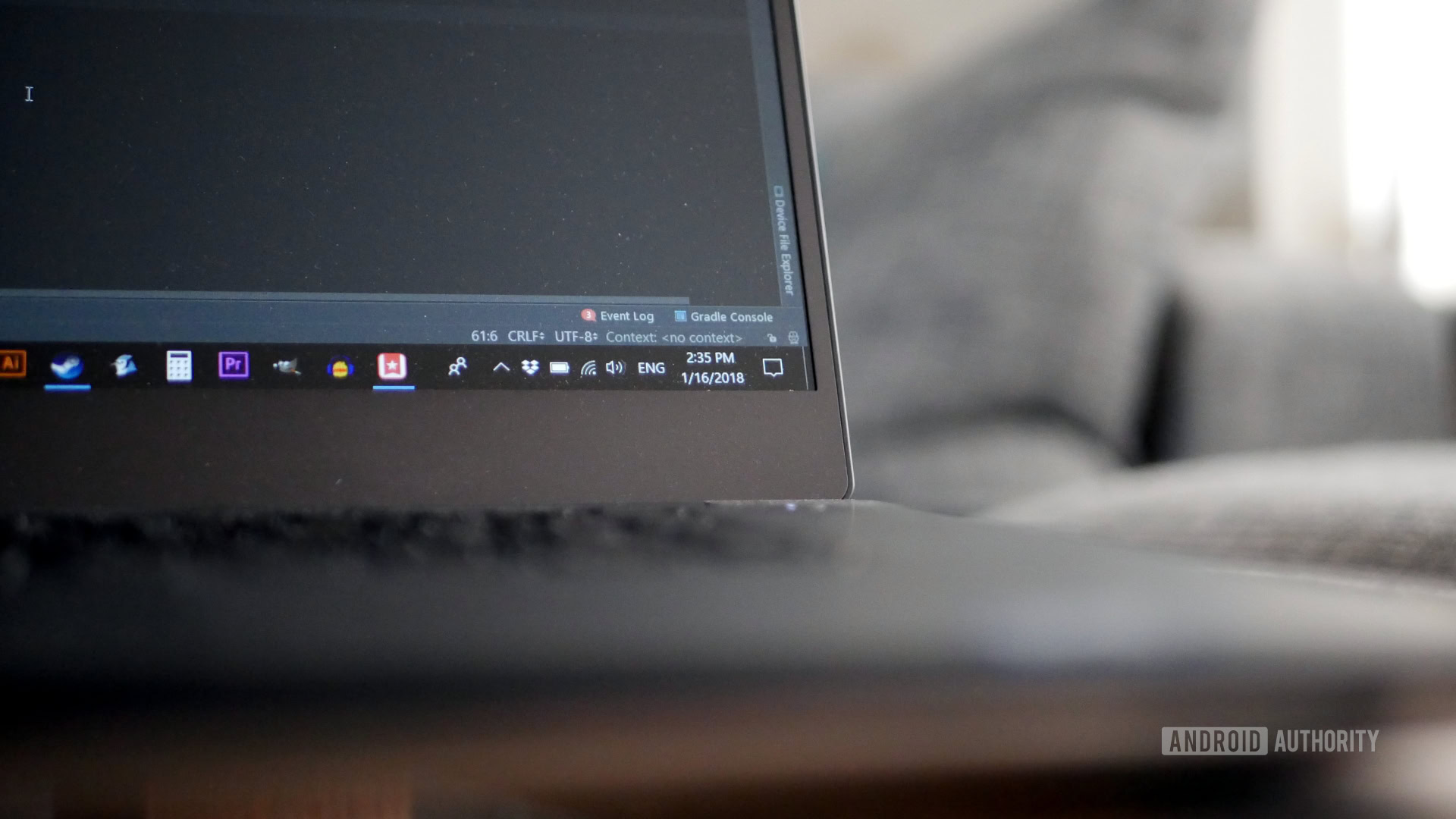
An array in Java is a type of variable that can store multiple values. It stores these values based on a key that can be used to subsequently look up that information.
Arrays can be useful for developers to store, arrange, and retrieve large data sets. Whether you are keeping track of high scores in a computer game, or storing information about clients in a database, an array is often the best choice.
Also read: How to use arrays in Python
So, how do you create an array in Java? That all depends on the type of array you want to use!
How to create an array in Java
The word “array” is defined as a data structure, consisting of a collection of elements. These elements must be identified by at least one “index” or “key.”
There are multiple data objects in Java that we could describe as arrays, therefore. We refer to the first as the “Java array.” Though making matters a little more confusing, this is actually most similar to what we would call a “list” in many other programming languages!
This is the easiest way to think about a Java array: as a list of sequential values. Here, a key is automatically assigned to each value in the sequence based on its relative position. The first index is always “0” and from there, the number will increase incrementally with each new item.
Unlike a list in say Python, however, Java arrays are of a fixed size. There is no way to remove elements or to add to the array at run time. This restriction is great for optimized code but of course does have some limitations.
To create this type of array in Java, simply create a new variable of your chosen data type with square brackets to indicate that it is indeed an array. We then enter each value inside curly brackets, separated by commas. Values are subsequently accessed by using the index based on the order of this list.
String listOfFruit[] = {"apple", "orange", "lemon", "pear", "grape"};
System.out.println(listOfFruit[2]);
While it’s not possible to change the size of a Java array, we can change specific values:
listOfFruit[3] = “melon”;
ArrayLists
If you need to use arrays in Java that can be resized, then you might opt for the ArrayList. An ArrayList is not as fast, but it will give you more flexibility at runtime.
To build an array list, you need to initialize it using our chosen data type, and then we can add each element individually using the add method. We also need to import ArrayList from the Java.util package.
import java.util.ArrayList;
class Main {
public static void main(String[] args) {
ArrayList<String> arrayListOfFruit = new ArrayList<String>();
arrayListOfFruit.add("Apple");
arrayListOfFruit.add("Orange");
arrayListOfFruit.add("Mango");
arrayListOfFruit.add("Banana");
System.out.println(arrayListOfFruit);
}
}
Now, at any point in our code, we will be able to add and remove elements. But keep in mind that doing so will alter the positions of all the other values and their respective keys. Thus, were I to do this:
System.out.println(arrayListOfFruit.get(3));
arrayListOfFruit.add(2, "Lemon");
System.out.println(arrayListOfFruit.get(3));
I would get a different output each time I printed. Note that we use “get” in order to return values at specific indexes, and that I can add values at different positions by passing my index as the first argument.
How to create an array in Java using maps
Another type of array in Java is the map. A map is an associative array that uses key/value pairs that do not change.
This is a perfect way to store phone numbers, for example. Here, you might use the numbers as the values and the names of the contacts as the index. So “197701289321” could be given the key “Jeff.” This makes it much easier for us to quickly find the data we need, even as we add and remove data from our list!
We do this like so:
import java.util.HashMap;
import java.util.Map;
Map<String, String> phoneBook = new HashMap<String, String>();
phoneBook.put("Adam", "229901239");
phoneBook.put("Fred", "981231999");
phoneBook.put("Dave", "123879122");
System.out.println("Adam's Number: " + phoneBook.get("Adam"));
As you can see then, a Java Array is always an array, but an array is not always a Java Array!
How to use the multidimensional array in Java
Head not spinning enough yet? Then take a look at the multidimensional array in Java!
This is a type of Java Array that has two “columns.”
Imagine that your typical Java array is an Excel spreadsheet. Were that the case, you’d have created a table with just a single column. We might consider it a “one dimensional” database, in that the data only changes from top to bottom. We have as many rows as we like (1st dimension) but only one column (the hypothetical 2nd dimension).
To add more columns, we simply add a second set of square brackets. We then populate the rows and columns. The resulting data structure can be thought of as an “array of arrays,” wherein each element is an entire array itself!
In this example, we are using integers (whole numbers):
int[][] twoDimensions = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12},
};
But we can actually take this idea even further by creating a three dimensional array! This would be an array of 2D arrays. You would build it like this:
int[][][] threeDimensions = {
{
{1, 2, 3},
{4, 5, 6}
},
{
{-1, -2, -3},
{-4, -5. -61},
}
};
Although this idea is tricky to conceptualize, try to imagine a database that has three axes, with cells that move in each direction.
So that is how you create an array in Java! While many people reading this will never need to concern themselves with three-dimensional arrays, it just goes to show how powerful and adaptable Java really is.
In fact, the list of things you can accomplish with Java is limitless. As is the Array List. Why not continue your education with one of the best resources to learn Java?
For more developer news, features, and tutorials from Android Authority, don’t miss signing up for the monthly newsletter below!