Affiliate links on Android Authority may earn us a commission. Learn more.
How to comment in Python - Tips and best practices
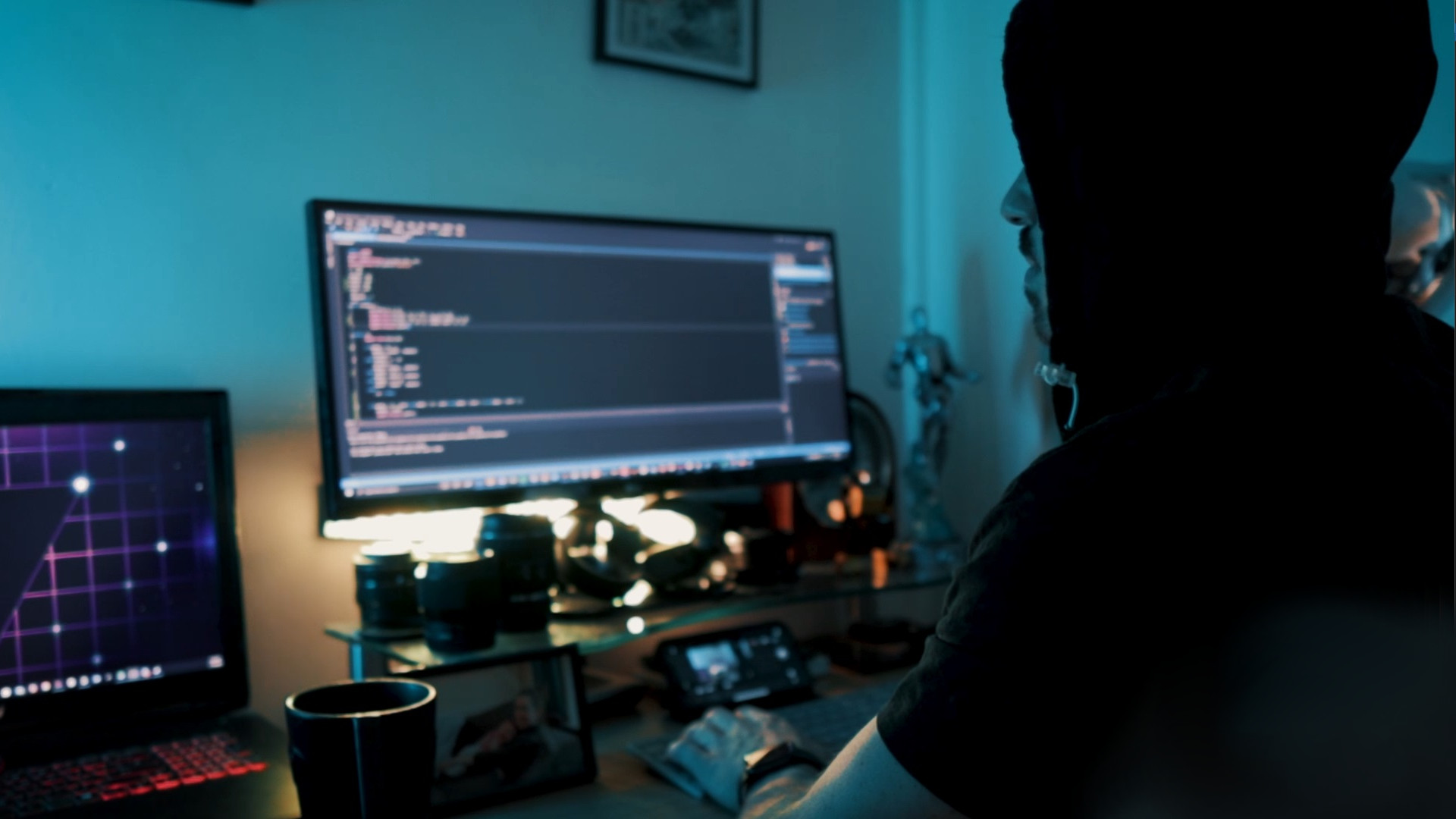
Commenting your code is good practice if you want to help other people understand what you’ve written. This makes it crucial to learn how to comment in Python if you work on a big team.
However, it’s also very important if you want to understand what you’ve written at a point in the future. Returning to old code can be disorienting, and this is a problem if you hope to offer ongoing support for an app.
Also read: How to use strings in Python
In this post, we’re going to look at how to comment in Python, and how to comment in a way that is logical and helpful.
How to comment in Python and make it useful
The good news is that it is extremely easy to comment in Python. You simply need to prefix whatever you are going to type with a hashtag:
#This is a comment!
This way, whatever you have written will be ignored by the interpreter and will be highlighted for anyone viewing your code. You can place a Python comment either on its own line, or even in-line with the code you want to explain.
Learning how to comment in Python is easy, then; the hard bit is knowing when to comment and how to ensure those comments are legible and helpful.
Also read: How to print in Python
One way to accomplish this is by ensuring that your comments follow basic best-practices. According to the Style Guide for Python Code, you should aim to keep your comments below 79 characters per line. This prevents the reader from needing to scroll horizontally and keeps everything neat.
While in-line comments can be useful, note that placing these consecutively can make it hard to know what’s code and what’s not – thus making it much more difficult to interpret the program at a glance.
This is confusing, for example:
if baddy_x + 40 > mine_x and baddy_y + 40 > mine_y and baddy_x < mine_x + 0 and baddy_y < mine_y + 19: #Checks the position of the bad guy in relation to the mine
baddy_x = 10000 #Sets the position of the bad guy to be far off the screen out of site
pygame.display.update() #Updates the graphics reflecting the new positions
for event in pygame.event.get(): #Looks for an event
if event.type == pygame.QUIT: #If the event is the player clicking the cross
run = False
A far better way to achieve something similar would be:
#If the baddy overlaps the mine, then the baddy is sent off page and the graphics update. Then we will check for events.
if baddy_x + 40 > mine_x and baddy_y + 40 > mine_y and baddy_x < mine_x + 0 and baddy_y < mine_y + 19:
baddy_x = 10000
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
But of course, either of these would be an example of unnecessary commenting!
When and how to comment in Python
As for what needs commenting…
Some common and useful captions to add to your code include:
- A little bit about any new function and what it does
- An explanation of what a variable or set of variables are for
- Explaining why you have done something a certain way (if it is not obvious)
- Highlighting key and important parts of your code
- Providing warnings
Some useful tips for keeping comments helpful rather than distracting:
- Keep comments succinct and no longer than necessary – be respectful of your reader’s time!
- Avoid comments that state the obvious; don’t over comment
- Don’t just explain what something does: explain why you put it there and why it’s important
- Be polite and friendly! Absolutely do not use comments to shame other coders. That’s a quick way to become the least popular person on your team.
More uses for Python comments
The main use for learning how to comment in Python is to provide useful guidance and instruction. This can help others navigate code. That said though, there are other scenarios where using code can be useful.
Also read: How to create a file in Python and more
Header comments, for example, go at the top of a file and can help to explain what the code underneath does. This could even include some useful directions that will help the reader to find important functions.
Header comments can also be used as a place to insert a copyright notice, or to declare your authorship of the code. Some people like to use over-the-top ASCII to give their code flamboyant titles.
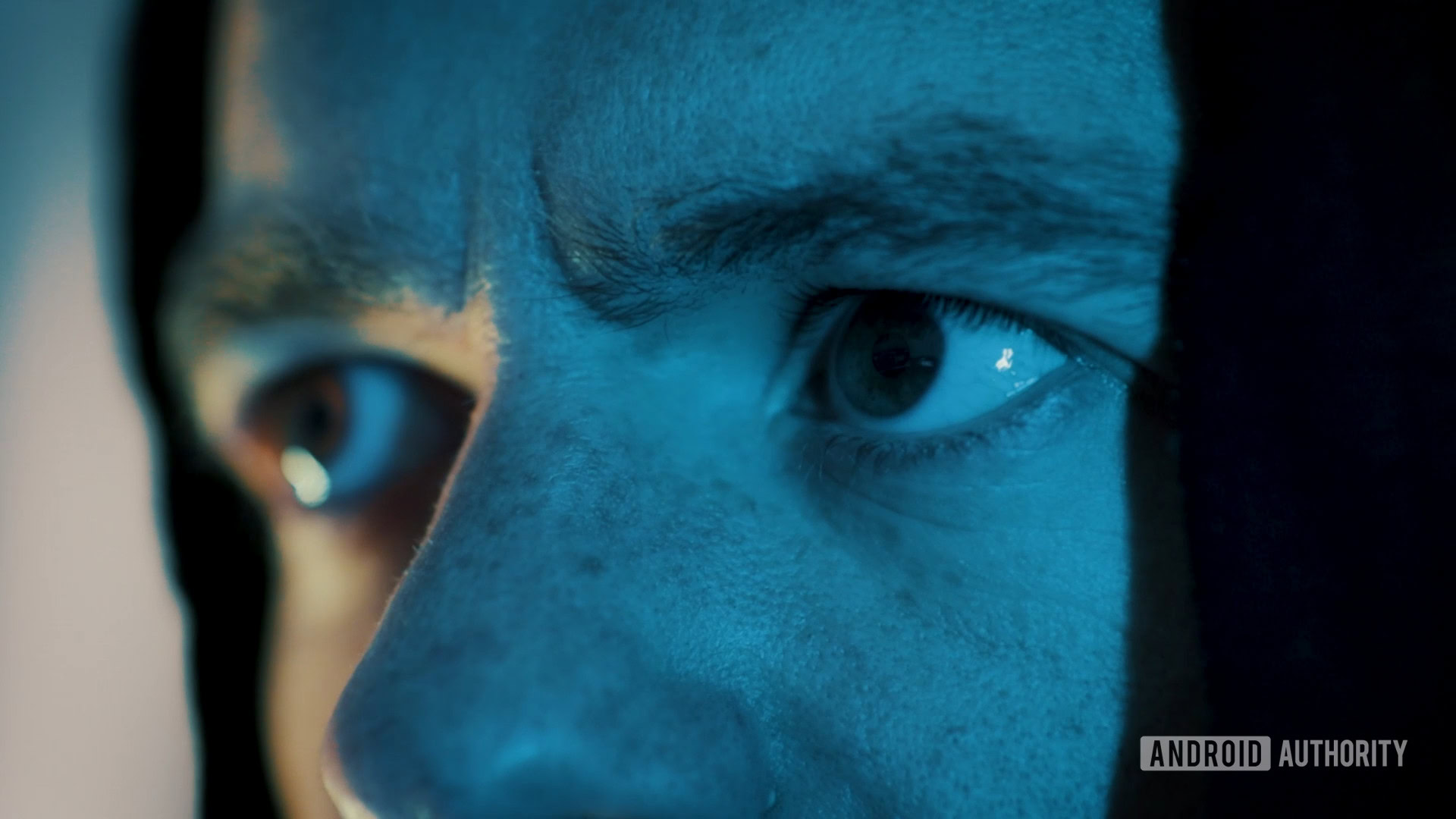
Another use for Python comments is to help you quickly find your way around your code with the search tool. I will often leave myself comments so that I can quickly jump between different points in my code, or as a way to mark something I need to do later. If I’m leaving something unfinished, I’ll often comment there so I can find it again easily at a later date.
Finally, you can use comments in Python to make jokes. This will irritate some people and it’s certainly not going to make your code as clean and efficient as possible. But personally? I find that programming can be a lonely job, and sometimes finding a little witticism or “hello” can lift the spirits.
It doesn’t cost anything to be nice!
Closing thoughts
Keep in mind that knowing how to comment in Python does not excuse you from needing to write clean, readable code. Your comments should serve as useful additional guidance for readers, not a Rosetta Stone for decoding your mad ramblings!
This means you should also:
- Structure your code in a logical manner
- Use smart names for variables and functions, along with a consistent naming convention
- Using new lines and indentations correctly (thankfully, Python forces us to do the latter)
There are those that believe commenting code is actually an indication that the code was not well-written to begin with. That crowd actually preaches against the use of comments altogether!
Ultimately, how sparingly or liberally you choose to comment your code is a matter of personal preference. But keep in mind that someone looking at your code may not be as experienced as you, and a little guidance could be a big help! The main goal is to ensure that anyone who needs to understand your code can, and as long as that’s the case, it’s up to you how you go about it!
So that’s how to comment in Python. What do you find helpful/irritating when reading code? Is there anything we missed? Let us know in the comments below!
If you want to learn more about Python coding, then we recommend trying an online course. This is the best way to quickly get to grips with a new programming language. Check out our breakdown of the best options.
For more developer news, features, and tutorials from Android Authority, don’t miss signing up for the monthly newsletter below!