Affiliate links on Android Authority may earn us a commission. Learn more.
Using the Android Percent Support Library
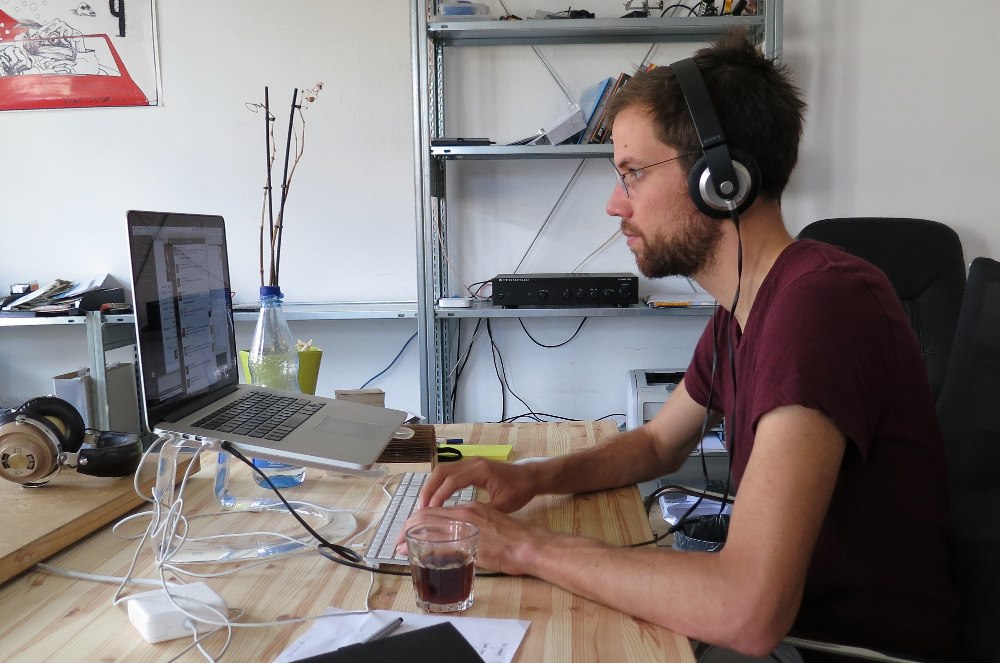
Android’s standard layouts can be confusing. When one attribute interacts with another attribute, you can get some surprising results. So it helps to have attributes with intuitive meanings, such as layout_widthPercent in the new Percent Support Library.
To test this new android.support.percent package, I created my own sample project. As usual, Android Studio created the default build.gradle and activity_mail.xml files. I tweaked the build.gradle file a tiny bit:
apply plugin: 'com.android.application'
android {
compileSdkVersion 21
buildToolsVersion "22.0.0"
defaultConfig {
applicationId "com.allmycode.percentlayout"
minSdkVersion 16
targetSdkVersion 21
versionCode 1
versionName "1.0"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile \
('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
compile 'com.android.support:percent:22.2.0'
}
In the build.gradle file, I changed the minimum SDK version to 16. More importantly, I added the line
compile 'com.android.support:percent:22.2.0'
to the file’s dependencies section. (Later, when I tried running the project, Android Studio noticed that I didn’t have this support:percent library on my local machine. Android Studio had no trouble locating the library for download and installing it automatically.)
Before editing the layout file’s XML code, I dropped three buttons from the Palette in Android Studio’s Designer Tool.
The Percent Support Library has two pre-built layouts – the PercentRelativeLayout and the PercentFrameLayout. To try out the PercentRelativeLayout, I edited some of the activity_main.xml file’s text:
<android.support.percent.PercentRelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:text="Button"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
app:layout_widthPercent="30%"/>
<Button
android:id="@+id/button2"
android:text="Button 2"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/button"
app:layout_widthPercent="60%"/>
<Button
android:id="@+id/button3"
android:text="Button 3"
android:layout_height="wrap_content"
android:layout_below="@+id/button"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
app:layout_widthPercent="90%"/>
</android.support.percent.PercentRelativeLayout>
In the activity_main.xml file, I typed only a few lines manually. Most importantly, I added three app:layout_widthPercent attributes. (The PercentRelativeLayout also has layout_heightPercent, layout_marginPercent, and other percentage-related attributes, but I used only layout_widthPercent in this first experiment.)
The new PercentRelativeLayout class extends Android’s existing RelativeLayout class. So, to specify the relative positions of things, I used familiar attributes like layout_toRightOf and layout_alignParentStart. I also mixed percentage sizes with the existing RelativeLayout sizes. In my example’s layout file, I specified android:layout_height=”wrap_content” for all three buttons.
Notice that, in each of the layout file’s <Button> elements, I didn’t bother specifying an android:layout_width attribute. That’s good because a layout_width attribute (even one that’s ignored by the runtime) would be redundant and confusing next to its layout_widthPercent cousin. Android Studio isn’t used to the lack of this layout_width attribute, so I saw an error marker on each <Button> element in the editor. But I ignored this error marker, and the code ran correctly.
Here’s my sample layout, running on a ZenFone 2 with Android 5.0:
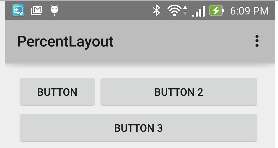
And here’s the same layout with the phone in landscape mode:
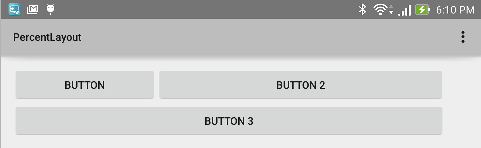
The layout adjusts nicely (and very naturally) to changes in the device’s orientation.
Of course, the Percent Support Library isn’t a silver bullet. This support library doesn’t eliminate interactions among attributes, and developers must be careful to test layouts before deploying them in the wild.
Here’s an example: I added a layout_centerHorizontal attribute to the first button in my layout:
<Button
android:id="@+id/button"
android:text="Button"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
app:layout_widthPercent="30%"/>
<Button
android:id="@+id/button2"
android:text="Button 2"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/button"
app:layout_widthPercent="60%"/>
When I did this, there wasn’t room to the right of the first button for a second button of width 60 percent, so Android ignored the second button’s layout_widthPercent=”60%” attribute:
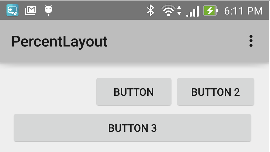
As an author, I’m excited about the new Percent layouts. I can spare my readers paragraphs of explanation about weights and other features by simply referring to the intuitive notion of percentage sizes.