Affiliate links on Android Authority may earn us a commission. Learn more.
How to develop a simple Android Wear app
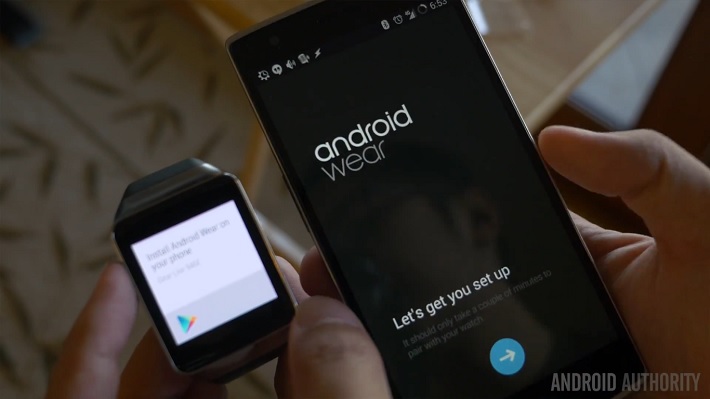
Hot Java Android Coding Bundle
If you are serious about coding you should checkout our Android Coding bundle course with 60+ Hours of Training.
Get all 5 courses for just: $29 $657 [95% off]
Before we begin, please keep the following at the back of your mind. Wearable apps, even though they are very similar to apps built for handhelds, should be quite small in size and functionality. You do not want to attempt to replicate the entire functionality of your handset app on a wearable. Rather, you should look for ways to complement the handheld app using the wearable. Ideally, most operations should be performed on the phone, and the results sent to the wearable.
Preparation
Our app will be a simple Android app, that sends a notification from a phone to a paired Wear device, with a corresponding wearable app, with a single clickable button.
For this article, we assume you are using Android Studio. Android Studio is the de-facto standard for Android app development. To develop apps for wearables, you need to update your SDK tools to version 23.0.0 or higher, and your SDK with Android 4.4W.2 or higher.
You should then set up either an Android Wear Device or an Android Wear Emulator.
For an emulator,
- Create an Android Wear square or round device using AVD Manager
- Start the emulator device
- Install the Android Wear app from Google Play
- Connect your handheld to your development machine through USB
- Forward the AVD communication port to the handheld device with the command
adb -d forward tcp:5601 tcp:5601
(this must be done every time you connect/reconnect your handset)
For an Android Wear Device,
- Install the Android Wear app on your smartphone via the Google Play Store
- Pair your handset and wearable using the instructions in the app
- Enable developer options on your wear device (tap build number seven times in Settings > About)
- Enable adb debugging
- Connect your wearable to your development machine, and you should be able to install and debug apps directly to your wearable.
Create your Project
The complete source code for this tutorial is available on github, but you might want to create your own project to get a feel for the process. Android Studio provides wizards to help create a project, and they are the best way to setup your Android wearable project. Click File > New Project, and follow the instructions
The process is similar to creating a phone/tablet project. Simply make sure you select both “Phone and Tablet” and “Wear” in the “Form Factors” window.
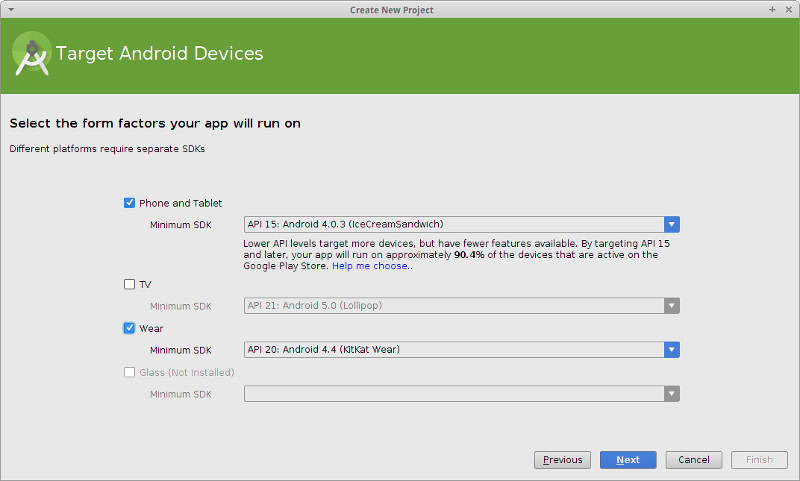
When the wizard completes, Android Studio will have created a new project with two modules, mobile and wear. For each module, you can create activities, services, layouts and more. Remember, the smartphone app (mobile module) should do most of the work, like intensive processing and network communications, and then send a notification to the wearable.
“mobile” module
The mobile module is the same Android development you are used to. For our mobile module, we create a simple Activity, with an EditText field, and a Button. The text entered into the EditText gets sent to the Wear device as a notification, when the Button is tapped.
The layout is pretty straightforward:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context=".MainActivity"
android:orientation="vertical">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="16dp"/>
<Button
android:id="@+id/actionButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
style="@style/Base.Widget.AppCompat.Button.Borderless"
android:text="@string/show_notification"
android:onClick="sendNotification" />
</LinearLayout>
The MainActivity is also pretty straightforward:
public class MainActivity extends AppCompatActivity {
EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = (EditText) findViewById(R.id.editText);
}
public void sendNotification(View view) {
String toSend = editText.getText().toString();
if(toSend.isEmpty())
toSend = "You sent an empty notification";
Notification notification = new NotificationCompat.Builder(getApplication())
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("AndroidAuthority")
.setContentText(toSend)
.extend(new NotificationCompat.WearableExtender().setHintShowBackgroundOnly(true))
.build();
NotificationManagerCompat notificationManager = NotificationManagerCompat.from(getApplication());
int notificationId = 1;
notificationManager.notify(notificationId, notification);
}
}
Notice that when building our Notification, we called the extend() method, providing a NotificationCompat.WearableExtender() object.
Running the mobile module
You run the mobile module the same way you run any other Android app. As long as you have it paired with a Wear device (emulator or real), running the project on your device will display notifications on your wearable.
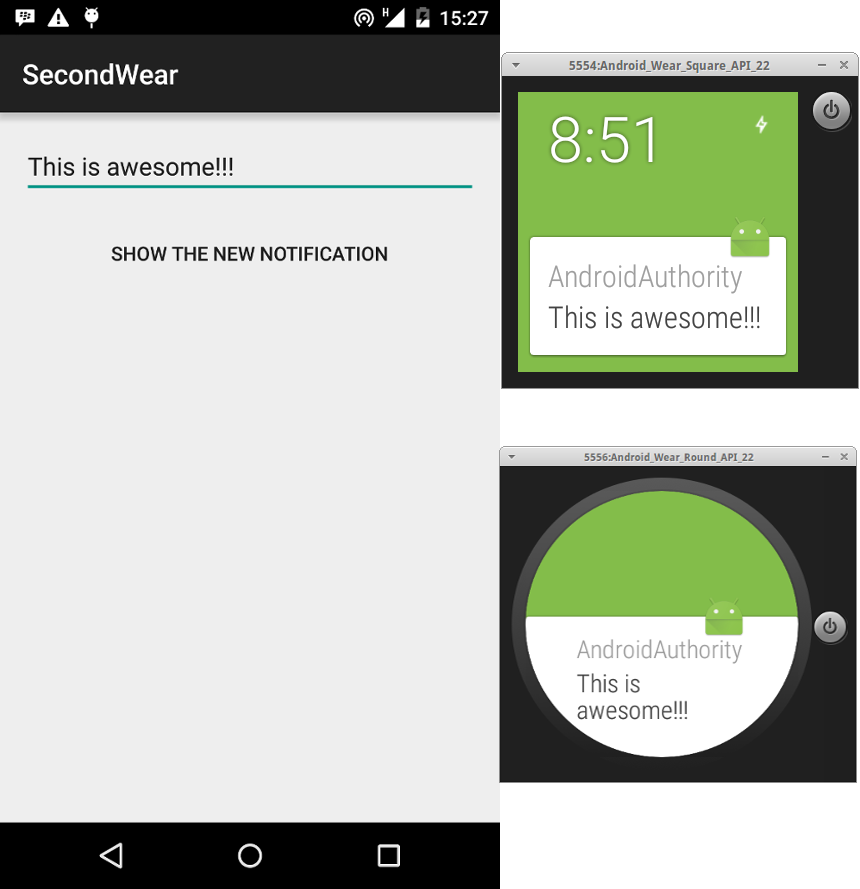
“wear” module
At this point, you should be able to view notifications from your mobile device on your wear device. We, however, are not content with that, and are going to build and run an actual Wear app. Wear devices, generally have a far less screen estate than handhelds, and are usually round or rectangular. This brings it’s own set of layout challenges. True to type, Google has some excellent design guidelines and UI patterns for Android Wear developers. The Wearable UI Library is included in your project automatically when you use the Android Studio project wizard to create your wearable app. Confirm that it’s there, if not then add it to your wear build.gradle file:
dependencies {
compile 'com.google.android.support:wearable:+'
}
If you created your project using the Android Studio Project Wizard, you will have an activity setup already for you with two different layout files for Round and Rectangular wear devices. The activity_wear.xml file is shown below:
<?xml version="1.0" encoding="utf-8"?>
<android.support.wearable.view.WatchViewStub
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/watch_view_stub"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:roundLayout="@layout/round_activity_wear"
app:rectLayout="@layout/rect_activity_wear"
tools:context=".WearActivity"
tools:deviceIds="wear">
</android.support.wearable.view.WatchViewStub>
Take note of the base widget. It is a WatchViewStub, which is a part of the Wearable UI library. You must declare the “app:” XML Namespace xmlns:app=”http://schemas.android.com/apk/res-auto” because the Wearable UI widgets declare their attributes using the “app:” namespace.
Take special note of the app:roundLayout and app:rectLayout items. This specifies the layout file to load depending on the shape of the wearable screen. Nifty!
Both our round_activity_wear.xml and rect_activity_wear.xml files are quite similar, except for a few caveats. The widgets in round_activity_wear are centered vertically and horizontally, while for rect_activity, they are simply centered horizontally. Using WatchViewStub, you have the freedom to design your layout completely differently for round and rectangular screens.
round_activity_wear.xml
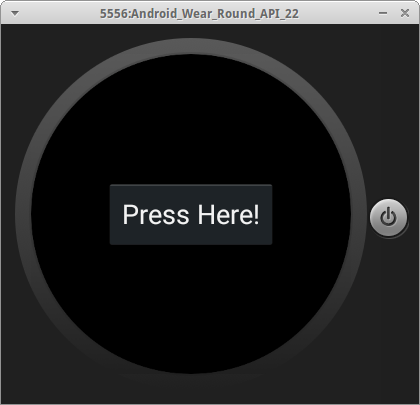
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_box="all"
tools:context=".WearActivity"
tools:deviceIds="wear_round">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="@string/hello_round"
android:onClick="beginCountdown" />
<android.support.wearable.view.DelayedConfirmationView
android:id="@+id/delayedView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
app:circle_border_color="@color/green"
app:circle_border_width="20dp"
app:circle_color="@color/white"
app:circle_radius="60dp"
app:circle_radius_pressed="60dp"
app:circle_padding="16dp"
app:update_interval="100"/>
</FrameLayout>
rect_activity_wear.xml
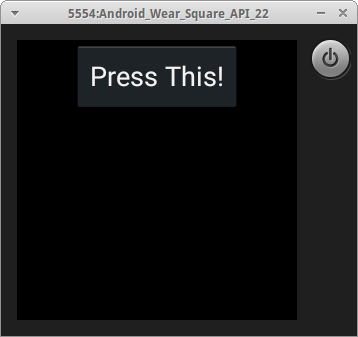
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".WearActivity"
tools:deviceIds="wear_square">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:text="@string/hello_square"
android:onClick="beginCountdown" />
<android.support.wearable.view.DelayedConfirmationView
android:id="@+id/delayedView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
app:circle_border_color="@color/green"
app:circle_border_width="20dp"
app:circle_color="@color/white"
app:circle_radius="60dp"
app:circle_radius_pressed="60dp"
app:circle_padding="16dp"
app:update_interval="100"/>
</FrameLayout>
WearActivity extends android.app.Activity (note not AppCompatActivity), just like any normal Android smartphone or tablet Activity. We set an OnLayoutInflatedListener object on our WatchViewStub, which gets called after the WatchViewStub has determined if the wearable device is round or rectangle. You locate your widgets using findViewById() in the onLayoutInflated method of the OnLayoutInflatedListener. In our case, we instantiate the Button and DelayedConfirmationView, and then call showOnlyButton() to hide the DelayedConfirmationView and show only the Button.
public class WearActivity extends Activity {
private Button button;
private DelayedConfirmationView delayedView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_wear);
final WatchViewStub stub = (WatchViewStub) findViewById(R.id.watch_view_stub);
stub.setOnLayoutInflatedListener(new WatchViewStub.OnLayoutInflatedListener() {
@Override
public void onLayoutInflated(WatchViewStub stub) {
button = (Button) stub.findViewById(R.id.button);
delayedView = (DelayedConfirmationView) stub.findViewById(R.id.delayedView);
delayedView.setTotalTimeMs(3000);
showOnlyButton();
}
});
}
public void beginCountdown(View view) {
button.setVisibility(View.GONE);
delayedView.setVisibility(View.VISIBLE);
delayedView.setListener(new DelayedConfirmationView.DelayedConfirmationListener() {
@Override
public void onTimerFinished(View view) {
showOnlyButton();
}
@Override
public void onTimerSelected(View view) {
}
});
delayedView.start();
}
public void showOnlyButton() {
button.setVisibility(View.VISIBLE);
delayedView.setVisibility(View.GONE);
}
}
Running the wear module
To run the wear module, select the wear run/debug configuration, and click the play button (or type Shift+F10). Since this is a debug build, you install directly to your wear device (or emulator). Make sure your device is connected (or a wear emulator is running) and select your device when prompted.
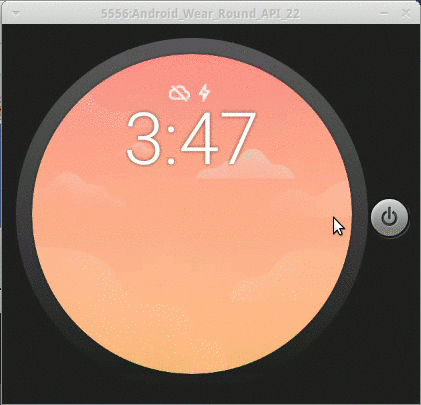
Deploying a release version
While you install your app directly unto a wearable during development, publishing and releasing an app for users is quite different. Your wearable app must be embedded in a handheld app, and it is automatically pushed onto wearables that are connected with the user’s handheld. Visit the Android Developer page on packaging wearable apps for more information on properly packaging your wearable app.
As usual, the complete code is available on github for use as you see fit. Happy coding.